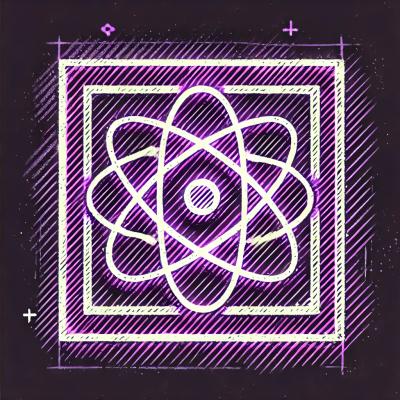
Security News
Create React App Officially Deprecated Amid React 19 Compatibility Issues
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
html-bundler-webpack-plugin
Advanced tools
HTML bundler plugin for webpack, extracts CSS and JS from their sources specified in HTML.
Warning
This is a brand new unique modern plugin that does exactly what you have wanted for a long time.
This plugin has come to replace an outdated, inconvenienthtml-webpack-plugin
and others.The plugin automatically extracts JS, CSS, images, fonts, etc. from their sources loaded directly in HTML via
<link>
<script>
<img>
tags.
Note
The purpose of this plugin is to make the developer's life much easier than it was with other plugins such as
html-webpack-plugin
andmini-css-extract-plugin
.
Note
The plugin is under continuous development. Many new feature will be implemented soon.
Кeep your finger on the pulse.
This plugin will work like the pug-plugin but the entry point is a HTML
file.
The plugin enable to use a HTML file as entry-point in Webpack, extracts JS and CSS files from their sources specified in HTML. The plugin generates HTML files containing hashed output JS and CSS filenames whose source files are specified in the HTML file.
The plugin automatically extracts the CSS from source style (e.g *.scss
, *.styl
) loaded via a <link>
tag and generates a separate file with hashed filename for it.
The plugin automatically extracts the JavaScript from source script (e.g. *.js
, *.ts
) loaded via a <script>
tag and generates a separate file with hashed filename for it.
The plugin automatically processes the images, fonts from sources loaded via <link>
, <img>
or <source>
tags and generates a separate file with hashed filename for it.
💡 Highlights:
Specify the HTML files in the Webpack entry:
const HtmlBundlerPlugin = require('html-bundler-webpack-plugin');
module.exports = {
entry: {
// define your HTML files here
index: './src/views/home/index.html', // output dist/index.html
about: './src/views/about/index.html', // output dist/about.html
},
plugins: [
// enable processing of HTML files defined in Webpack entry
new HtmlBundlerPlugin({
js: {
// output filename of extracted JS file from source script
filename: 'assets/js/[name].[contenthash:8].js',
},
css: {
// output filename of extracted CSS file from source style
filename: 'assets/css/[name].[contenthash:8].css',
},
}),
],
module: {
rules: [
{
test: /.html/,
loader: HtmlBundlerPlugin.loader, // HTML loader
},
],
},
};
Add source scripts and styles directly to HTML using relative path or Webpack alias:
<html>
<head>
<link href="./styles.scss" rel="stylesheet">
<script src="./main.js" defer="defer"></script>
</head>
<body>
<h1>Hello World!</h1>
</body>
</html>
The generated HTML contains hashed output CSS and JS filenames:
<html>
<head>
<link href="/assets/css/styles.05e4dd86.css" rel="stylesheet">
<script src="/assets/js/main.f4b855d8.js" defer="defer"></script>
</head>
<body>
<h1>Hello World!</h1>
</body>
</html>
The <link>
and <script>
tags stay where they are written.
This can be very important in some cases, as opposed to using other plugins like html-webpack-plugin
and mini-css-extract-plugin
that injects these tags somewhere in the HTML.
Just one HTML bundler plugin replaces the functionality of the plugins and loaders:
Package | Features |
---|---|
html-webpack-plugin | extract HTML and save in a file |
mini-css-extract-plugin | extract CSS and save in a file |
webpack-remove-empty-scripts | remove empty JS files generated by the mini-css-extract-plugin |
resolve-url-loader | resolve url in CSS |
svg-url-loader | encode SVG data-URL as utf8 |
posthtml-inline-svg | inline SVG icons in HTML |
It's very easy! Add to Webpack config the rule:
module: {
rules: [
{
test: /\.(png|jpe?g|ico)/,
type: 'asset/resource',
generator: {
filename: 'assets/img/[name].[hash:8][ext]',
},
},
],
Now you can write source file (relative path or a Webpack alias) in HTML:
<html>
<head>
<link href="./favicon.ico" rel="icon" />
</head>
<body>
<img src="./apple.png" srcset="./apple1.png 320w, ./apple2.png 640w" alt="apple">
<picture>
<source srcset="./fig1.jpg, ./fig2.jpg 320w, ./fig3.jpg 640w">
</picture>
</body>
</html>
The generated HTML contains hashed output images filenames:
<html>
<head>
<link href="/assets/img/favicon.05e4dd86.ico" rel="icon" />
</head>
<body>
<img src="/assets/img/apple.f4b855d8.png" srcset="/assets/img/apple1.855f4bd8.png 320w, /assets/img/apple2.d8f4b855.png 640w" alt="apple">
<picture>
<source srcset="/assets/img/fig1.605e4dd8.jpg, /assets/img/fig2.8605e4dd.jpg 320w, /assets/img/fig3.e4605dd8.jpg 640w">
</picture>
</body>
</html>
It's very easy! Add to Webpack config the rule:
module: {
rules: [
{
test: /\.(eot|ttf|woff|woff2)/,
type: 'asset/resource',
generator: {
filename: 'assets/fonts/[name][ext]',
},
},
],
Now you can write source file (relative path or a Webpack alias) in HTML:
<html>
<head>
<link href="./open-sans-regular.woff2" rel="preload" as="font" type="font/woff2" />
</head>
<img src="./apple.png" alt="apple">
</html>
The generated HTML contains output fonts filenames:
<html>
<head>
<link href="/assets/fonts/open-sans-regular.woff2" rel="preload" as="font" type="font/woff2" />
</head>
<body>
<h1>Hello World!</h1>
</body>
</html>
Note
Now you don't need a plugin to copy files from source directory to public.
0.1.0 (2023-01-12)
First beta release:
js
plugin option to extract JavaScript files from source scripts loaded in HTML via a <script>
tag and generates a separate file for itcss
plugin option to extract CSS files from source styles loaded in HTML via a <link>
tag and generates a separate file for it<link>
, <img>
or <source>
tags and generates a separate file for iturl()
in a style (css, scss)publicPath
FAQs
Generates complete single-page or multi-page website from source assets. Build-in support for Markdown, Eta, EJS, Handlebars, Nunjucks, Pug. Alternative to html-webpack-plugin.
The npm package html-bundler-webpack-plugin receives a total of 2,231 weekly downloads. As such, html-bundler-webpack-plugin popularity was classified as popular.
We found that html-bundler-webpack-plugin demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
Security News
Oracle seeks to dismiss fraud claims in the JavaScript trademark dispute, delaying the case and avoiding questions about its right to the name.
Security News
The Linux Foundation is warning open source developers that compliance with global sanctions is mandatory, highlighting legal risks and restrictions on contributions.