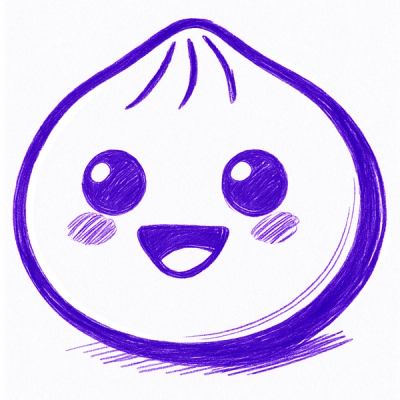
Security News
Bun 1.2.19 Adds Isolated Installs for Better Monorepo Support
Bun 1.2.19 introduces isolated installs for smoother monorepo workflows, along with performance boosts, new tooling, and key compatibility fixes.
html-form-validation
Advanced tools
This module is to validate HTML forms. Text fields, emails, phones, checkobxes etc. Check out demo.
Install validator module
npm i -S html-form-validation
or with yarn
yarn add html-form-validation
Add validator to your project
AMD
require(['html-form-validation'], function (Validator) {});
CommonJS
var Validator = require('html-form-validation');
ES6
import Validator from 'html-form-validation';
Inline
<script src="html-form-validation.js"></script>
Also, include CSS file
<link href="validator.css" rel="stylesheet">
Validator module needs proper HTML-markup (more in example section)
<form>
<!-- Email field -->
<label class="form-input" data-validation="required" data-validation-type="email">
<input type="email">
<div class="error"></div>
</label>
<!-- Text field. With min and max length -->
<label class="form-input" data-validation="required" data-validation-type="text">
<textarea data-validation-condition="length" data-min-length="50" data-max-length="200"></textarea>
<div class="error"></div>
</label>
<!-- Text field. With custom error message, 'equal' codition -->
<label class="form-input" data-validation="required" data-validation-type="text" data-validation-text="Incorrect data">
<input type="text" data-validation-condition="equal" data-equal="dataToCompare">
<div class="error"></div>
</label>
<!-- Validate form button -->
<button class="validate-form-button" type="submit">Validate form</button>
</form>
// initialize
$('form').validator();
// initialize with options
$('form').validator({
removeErrorOnFocusOut: true
});
// import validator
import Validator from 'html-form-validation';
// fix jQuery conflict
Validator.expose($);
// use it
$('form').validator();
Option name | Possible values | Description |
---|---|---|
data-validation | required / false | Validates the field when set to true. |
data-validation-type | text / phone / email / checkbox / radio / select | Which method used to validate field. Each type has its own. |
data-validation-text | any string | Text used as error message. Otherwise validator will use its own messages for every field type. |
Type | Description | Available input types |
---|---|---|
text | Validates text field. Input can have additional attribute data-validation-condition with available length and equal values. If set to length - Validator will look for data-min-length, data-max-length or data-length attributes. If set to equal - Validator will look for data-equal attribute. Then validator will match value with values from attributes. | input / textarea |
phone | Under development. No additional options are available. | input |
Validates email field. No additional options are available. | input | |
checkbox | At least one checkbox in field should be checked and visible. No additional options are available. | input[type="checkbox"] |
radio | At lease one radio should be selected. No additional options are available. | input[type="radio"] |
select | Checks for selected option. Its value should not equal 0 or false. No additional options are available. | select |
Type: Object
Default: {}
AJAX options. If set - request will be performed after validation (if form is valid).
Type: String
Default: en
Error text language (en/ru).
Type: Boolean
Default: false
When true, remove fields incorrect state when clicked outside the form.
Type: String
Default: '.form-input'
Form fields selector string.
Type: Function
Default: null
Parameter: validator
Example:
$('form').validator({
beforeValidation: function (validator) {
console.log('performed before validation');
}
});
Callback performed before form validation.
Type: Function
Default: null
Parameter: validator
Callback performed after form validation.
Type: Function
Default: null
Parameter: validator
Callback performed after validation, if form is valid (before AJAX).
// initialize and get access to validator's instance
// (if inited on multiple jQuery objects returns an array of instances)
var validatorInstance = $('form').validator();
// run form validation
validatorInstance.runFormValidation();
// reset form
validatorInstance.resetForm();
// get serialized data
var formData = validatorInstance.serializedFormData();
// unbind validation from button
validatorInstance.unbindOnClick();
/**
* Expose validator module as jquery plugin.
* Use before initialazing validator.
* (fixes jquery conflict when using webpack's "import")
*
* @static
* @param {jQuery} jQuery
*/
Validator.expose($);
Current version is 0.2.2
FAQs
HTML forms validation plugin
The npm package html-form-validation receives a total of 1 weekly downloads. As such, html-form-validation popularity was classified as not popular.
We found that html-form-validation demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Bun 1.2.19 introduces isolated installs for smoother monorepo workflows, along with performance boosts, new tooling, and key compatibility fixes.
Security News
Popular npm packages like eslint-config-prettier were compromised after a phishing attack stole a maintainer’s token, spreading malicious updates.
Security News
/Research
A phishing attack targeted developers using a typosquatted npm domain (npnjs.com) to steal credentials via fake login pages - watch out for similar scams.