HTML Lightweight Template Engine v1.3.0 Documentation
Table of contents
Description
When you simply want to use template interpolation {{ someValue }}
and
Angular like ng-container
and ng-template
elements (with the limited
functionality) in plain HTML without any frameworks, you can use this
library to do that.
Installation
For the usage in ESM run the following command:
npm i html-lightweight-template-engine
For the script tag usage include the following script:
<!DOCTYPE html>
<html>
<body>
<script src="https://cdn.jsdelivr.net/npm/html-lightweight-template-engine@1.3.0/dist/html-lightweight-template-engine.min.js"></script>
</body>
</html>
Usage example
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8" />
<meta name="viewport" content="width=device-width, initial-scale=1" />
<title>{{ pageTitle }}</title>
</head>
<body>
<p>{{ greeting }}</p>
<app-container appTemplateOutlet="myIcon"></app-container>
<app-template myIcon>
<svg height="100" width="100">
<circle
cx="50"
cy="50"
r="40"
stroke="black"
stroke-width="3"
fill="yellow"
/>
</svg>
</app-template>
<script src="https://cdn.jsdelivr.net/npm/html-lightweight-template-engine@1.3.0/dist/html-lightweight-template-engine.min.js"></script>
<script>
window.onload = () => {
const templateEngine =
new htmlLightweightTemplateEngine.HtmlLightweightTemplateEngine({
pageTitle: "Mia's Blog",
greeting: 'Good evening'
})
templateEngine.renderTemplate()
}
</script>
</body>
</html>
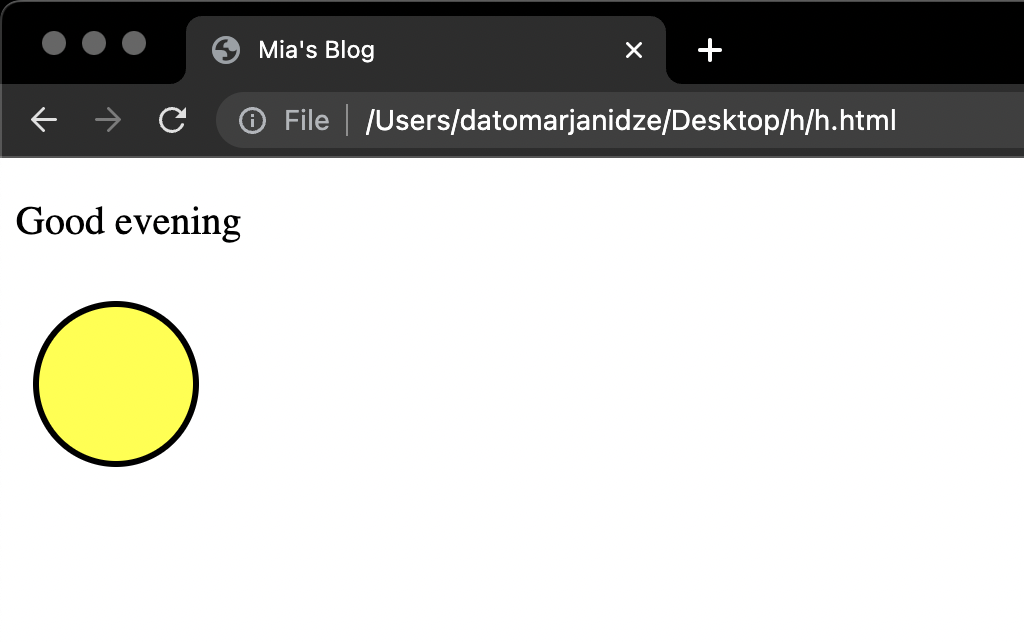
API
HtmlLightweightTemplateEngine
class constructor parameters:
texts
{[key: string]: string}: interpolation key-valueselementAttributePrefix
{string}: this value is used as a prefix to reference custom elements and attributes. Default - 'app'