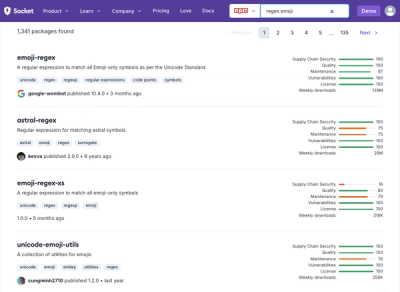
Security News
Weekly Downloads Now Available in npm Package Search Results
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.
http-browserify
Advanced tools
The http-browserify package is a browser-compatible implementation of the Node.js 'http' module. It allows developers to use the same HTTP client code in both Node.js and browser environments, facilitating code reuse and simplifying the development process for applications that need to make HTTP requests.
Making HTTP GET Requests
This feature allows you to make HTTP GET requests from the browser, similar to how you would in a Node.js environment. The code sample demonstrates how to perform a GET request to 'http://example.com' and handle the response.
const http = require('http-browserify');
http.get('http://example.com', (response) => {
let data = '';
response.on('data', (chunk) => {
data += chunk;
});
response.on('end', () => {
console.log(data);
});
}).on('error', (err) => {
console.error('Error: ' + err.message);
});
Making HTTP POST Requests
This feature allows you to make HTTP POST requests from the browser. The code sample demonstrates how to send a POST request with JSON data to 'http://example.com/upload' and handle the response.
const http = require('http-browserify');
const postData = JSON.stringify({
'msg': 'Hello World'
});
const options = {
hostname: 'example.com',
port: 80,
path: '/upload',
method: 'POST',
headers: {
'Content-Type': 'application/json',
'Content-Length': Buffer.byteLength(postData)
}
};
const req = http.request(options, (res) => {
let data = '';
res.on('data', (chunk) => {
data += chunk;
});
res.on('end', () => {
console.log(data);
});
});
req.on('error', (e) => {
console.error(`Problem with request: ${e.message}`);
});
req.write(postData);
req.end();
Axios is a popular promise-based HTTP client for the browser and Node.js. It provides a simple and easy-to-use API for making HTTP requests and supports features like interceptors, automatic JSON transformation, and request cancellation. Compared to http-browserify, Axios offers a more modern and feature-rich API.
Fetch is a built-in web API for making HTTP requests in the browser. It provides a modern and flexible interface for fetching resources and handling responses. Unlike http-browserify, Fetch is natively supported in modern browsers and does not require any additional libraries.
Superagent is a small, progressive HTTP request library for Node.js and the browser. It provides a simple and intuitive API for making HTTP requests and supports features like file uploads, redirects, and query string parsing. Superagent is similar to http-browserify but offers additional features and a more user-friendly API.
The http module from node.js, but for browsers.
When you require('http')
in
browserify,
this module will be loaded.
var http = require('http');
http.get({ path : '/beep' }, function (res) {
var div = document.getElementById('result');
div.innerHTML += 'GET /beep<br>';
res.on('data', function (buf) {
div.innerHTML += buf;
});
res.on('end', function () {
div.innerHTML += '<br>__END__';
});
});
var http = require('http');
options
can have:
The callback will be called with the response object.
A shortcut for
options.method = 'GET';
var req = http.request(options, cb);
req.end();
Set an http header.
Get an http header.
Remove an http header.
Write some data to the request body.
Close and send the request body, optionally with additional data
to append.
Return an http header, if set. key
is case-insensitive.
This module has been tested and works with:
Multipart streaming responses are buffered in all versions of Internet Explorer
and are somewhat buffered in Opera. In all the other browsers you get a nice
unbuffered stream of "data"
events when you send down a content-type of
multipart/octet-stream
or similar.
You can do:
var bundle = browserify({
require : { http : 'http-browserify' }
});
in order to map "http-browserify" over require('http')
in your browserified
source.
FAQs
http module compatability for browserify
We found that http-browserify demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.
Security News
A Stanford study reveals 9.5% of engineers contribute almost nothing, costing tech $90B annually, with remote work fueling the rise of "ghost engineers."
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.