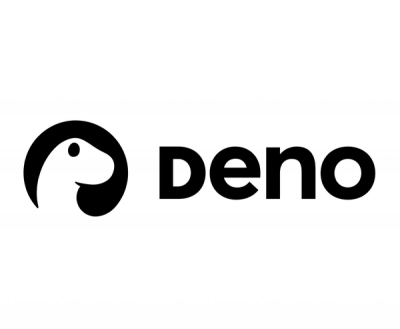
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
http-client
Advanced tools
http-client lets you compose HTTP clients using JavaScript's fetch API. This library has the following goals:
Using npm:
$ npm install --save http-client
http-client requires you to bring your own global fetch
function. isomorphic-fetch is a great polyfill.
Then, use as you would anything else:
// using ES6 modules
import { fetch, createFetch } from 'http-client'
// using CommonJS modules
var fetch = require('http-client').fetch
var createFetch = require('http-client').createFetch
The UMD build is also available on npmcdn:
<script src="https://npmcdn.com/http-client/umd/http-client.min.js"></script>
You can find the library on window.HTTPClient
.
http-client simplifies the process of creating flexible HTTP clients that work in both node and the browser. You create your own fetch
function using the createFetch
method, optionally passing middleware as arguments.
import { createFetch, base, accept, parseJSON } from 'http-client'
const fetch = createFetch(
base('https://api.stripe.com/v1'), // Prefix all request URLs
accept('application/json'), // Set "Accept: application/json" in the request headers
parseJSON() // Read the response as JSON and put it in response.jsonData
)
fetch('/customers/5').then(response => {
console.log(response.jsonData)
})
http-client also exports a base fetch
function if you need it (i.e. don't want middleware).
fetch([input], [options])
An enhanced fetch
function. Use this directly if you don't need any middleware.
createFetch(...middleware)
Creates a fetch
function that is fronted by some middleware. This function has the same signature as the enhanced fetch
function in the top-level API.
createStack(...middleware)
Combines several middleware into one, in the same order they are provided as arguments. Use this function to create re-usable middleware stacks.
handleResponse(handler)
A helper for creating middleware that enhances the response
object in some way. The handler
function should return the new response value, or a promise for it. This function is used internally to create the parseText
and parseJSON
middleware.
http-client provides a variety of middleware that may be used to extend the functionality of the client. Out of the box, http-client ships with the following middleware:
init(propertyName, value)
Sets the value of an arbitrary property in the options object.
import { createFetch, init } from 'http-client'
const fetch = createFetch(
init('credentials', 'include')
)
method(verb)
Sets the request method.
import { createFetch, method } from 'http-client'
const fetch = createFetch(
method('POST')
)
header(name, value)
Adds a header to the request.
import { createFetch, header } from 'http-client'
const fetch = createFetch(
header('Content-Type', 'application/json')
)
auth(value)
Adds an Authorization
header to the request.
import { createFetch, auth } from 'http-client'
const fetch = createFetch(
auth('Bearer ' + oauth2Token)
)
accept(contentType)
Adds an Accept
header to the request.
import { createFetch, accept } from 'http-client'
const fetch = createFetch(
accept('application/json')
)
base(baseURL)
Adds the given baseURL
to the beginning of the request URL.
import { createFetch, base } from 'http-client'
const fetch = createFetch(
base('https://api.stripe.com/v1')
)
fetch('/customers/5') // GET https://api.stripe.com/v1/customers/5
query(object)
Adds the data in the given object (or string) to the query string of the request URL.
body(content, contentType)
Sets the given content
string as the request body.
import { createFetch, body } from 'http-client'
const fetch = createFetch(
body(JSON.stringify(data), 'application/json')
)
json(object)
Adds the data in the given object as JSON to the request body.
params(object)
Adds the given object to the query string of GET
/HEAD
requests and as a x-www-form-urlencoded
payload on all others.
import { createFetch, method, params } from 'http-client'
// Create a client that will append hello=world to the URL in the query string
const fetch = createFetch(
params({ hello: 'world' })
)
// Create a client that will send hello=world as POST data
const fetch = createFetch(
method('POST'),
params({ hello: 'world' })
)
parseText(propertyName = 'textString')
Reads the response body as text and puts it on response.textString
.
import { createFetch, parseText } from 'http-client'
const fetch = createFetch(
parseText()
)
fetch(input).then(response => {
console.log(response.textString)
})
parseJSON(propertyName = 'jsonData')
Reads the response body as JSON and puts it on response.jsonData
.
import { createFetch, parseJSON } from 'http-client'
const fetch = createFetch(
parseJSON()
)
fetch(input).then(response => {
console.log(response.jsonData)
})
requestInfo()
Adds requestURL
and requestOptions
properties to the response (or error) object so you can inspect them. Mainly useful for testing/debugging (should be put last in the list of middleware).
import { createFetch, requestInfo } from 'http-client'
const fetch = createFetch(
// ...
requestInfo()
)
fetch(input).then(response => {
console.log(response.requestURL, response.requestOptions)
})
Middleware may be combined together into re-usable middleware "stacks" using createStack
. A stack is itself a middleware that is composed of one or more other pieces of middleware.
This is useful when you have a common set of functionality that you'd like to share between several different fetch
methods, e.g.:
import { createStack, createFetch, header, base, parseJSON } from 'http-client'
const commonStack = createStack(
header('X-Auth-Key', key),
header('X-Auth-Email', email),
base('https://api.cloudflare.com/client/v4'),
parseJSON()
)
// This fetch function can be used standalone...
const fetch = createFetch(commonStack)
// ...or we can add further middleware to create another fetch function!
const fetchSinceBeginningOf2015 = createFetch(
commonStack,
query({ since: '2015-01-01T00:00:00Z' })
)
[v4.0.0-0]
Apr 27, 2016
handleResponse
runs response handlers top to bottom, just like Expressoptions.responseHandlers
array to specify an array of transforms to
run on the response after it is receivedenhanceFetch
to top-level exports for adding options.responseHandlers
support to an arbitrary fetch
functionoptions.fetch
to fetch functions created using createFetch
so users
can swap out usage of the "global" fetch function, which makes testing easierfetch
method signatureFAQs
Compose HTTP clients using JavaScript's fetch API
The npm package http-client receives a total of 3,940 weekly downloads. As such, http-client popularity was classified as popular.
We found that http-client demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.