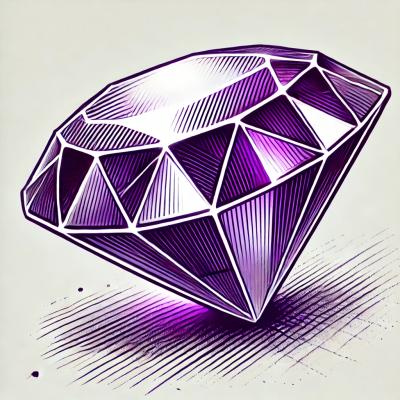
Security News
RubyGems.org Adds New Maintainer Role
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
http2-proxy
Advanced tools
A simple http/2 & http/1.1 to http/1.1 spec compliant proxy helper for Node.
A simple http/2 & http/1.1 to http/1.1 spec compliant proxy helper for Node.
$ npm install http2-proxy
http2-proxy
requires at least node v9.5.0.
Request & Response errors are emitted to the server object either as clientError
for http/1 or streamError
for http/2. See the NodeJS documentation for further details.
You need to use an final and/or error handler since errored responses won't be cleaned up automatically.
const finalhandler = require('finalhandler')
const defaultWebHandler = (err, req, res) => {
if (err) {
console.error('proxy error', err)
finalhandler(req, res)(err)
}
}
const defaultWSHandler = (err, req, socket, head) => {
if (err) {
console.error('proxy error', err)
socket.destroy()
}
}
You must pass allowHTTP1: true
to the http2.createServer
or http2.createSecureServer
factory methods.
import http2 from 'http2'
import proxy from 'http2-proxy'
const server = http2.createServer({ allowHTTP1: true })
server.listen(8000)
You can also use http-proxy2
with the old http
&& https
API's.
import http from 'http'
const server = http.createServer()
server.listen(8000)
server.on('request', (req, res) => {
proxy.web(req, res, {
hostname: 'localhost'
port: 9000
}, defaultWebHandler)
})
server.on('upgrade', (req, socket, head) => {
proxy.ws(req, socket, head, {
hostname: 'localhost'
port: 9000
}, defaultWsHandler)
})
const app = connect()
app.use(helmet())
app.use((req, res, next) => proxy
.web(req, res, {
hostname: 'localhost'
port: 9000
}, err => {
if (err) {
next(err)
}
})
)
server.on('request', app)
server.on('request', (req, res) => {
proxy.web(req, res, {
hostname: 'localhost'
port: 9000,
onReq: (req, { headers }) => {
headers['x-forwarded-for'] = req.socket.remoteAddress
headers['x-forwarded-proto'] = req.socket.encrypted ? 'https' : 'http'
headers['x-forwarded-host'] = req.headers['host']
}
}, defaultWebHandler)
})
const http = require('follow-redirects').http
server.on('request', (req, res) => {
proxy.web(req, res, {
hostname: 'localhost'
port: 9000,
onReq: (req, options) => http.request(options)
}, defaultWebHandler)
})
req
: http.IncomingMessage
or http2.Http2ServerRequest
.res
: http.ServerResponse
or http2.Http2ServerResponse
.options
: See Optionscallback(err, req, res)
: Called on completion or error. Optional.See request
req
: http.IncomingMessage
.socket
: net.Socket
.head
: Buffer
.options
: See Options.callback(err, req, socket, head)
: Called on completion or error. Optional.See upgrade
hostname
: Proxy http.request(options)
target hostname.port
: Proxy http.request(options)
target port.protocol
: 'string' agent protocol ('http' or 'https'). Defaults to 'http'.path
: 'string' target pathname. Defaults to req.originalUrl || req.url
.proxyTimeout
: Proxy http.request(options)
timeout.proxyName
: Proxy name used for Via header.timeout
: http.IncomingMessage
or http2.Http2ServerRequest
timeout.onReq(req, options)
: Called before proxy request. If returning a truthy value it will be used as the request.
req
: http.IncomingMessage
or http2.Http2ServerRequest
options
: Options passed to http.request(options)
.onRes(req, resOrSocket, proxyRes, callback)
: Called before proxy response.
req
: http.IncomingMessage
or http2.Http2ServerRequest
.resOrSocket
: For web
http.ServerResponse
or http2.Http2ServerResponse
and for ws
net.Socket
.proxyRes
: http.ServerResponse
.callback(err)
: Called on completion or error..FAQs
A simple http/2 & http/1.1 spec compliant proxy helper for Node.
The npm package http2-proxy receives a total of 21,235 weekly downloads. As such, http2-proxy popularity was classified as popular.
We found that http2-proxy demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.
Security News
Research
Socket's threat research team has detected five malicious npm packages targeting Roblox developers, deploying malware to steal credentials and personal data.