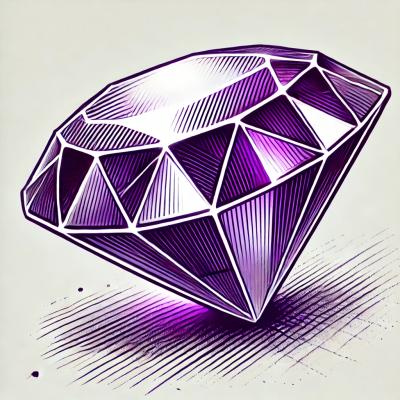
Security News
RubyGems.org Adds New Maintainer Role
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
http2-proxy
Advanced tools
A simple http/2 & http/1.1 to http/1.1 spec compliant proxy helper for Node.
A simple http/2 & http/1.1 to http/1.1 spec compliant proxy helper for Node.
$ npm install http2-proxy
http2-proxy
requires at least node v10.0.0.
Fully async/await compatible and all callback based usage is optional and discouraged.
During 503 it is safe to assume that the request never made it to the upstream server. This makes it safe to retry non idempotent methods.
Use a final and/or error handler since errored responses won't be cleaned up automatically. This makes it possible to perform retries.
const finalhandler = require('finalhandler')
const defaultWebHandler = (err, req, res) => {
if (err) {
console.error('proxy error', err)
finalhandler(req, res)(err)
}
}
const defaultWSHandler = (err, req, socket, head) => {
if (err) {
console.error('proxy error', err)
socket.destroy()
}
}
You must pass allowHTTP1: true
to the http2.createServer
or http2.createSecureServer
factory methods.
import http2 from 'http2'
import proxy from 'http2-proxy'
const server = http2.createServer({ allowHTTP1: true })
server.listen(8000)
You can also use http-proxy2
with the old http
&& https
API's.
import http from 'http'
const server = http.createServer()
server.listen(8000)
server.on('request', (req, res) => {
proxy.web(req, res, {
hostname: 'localhost'
port: 9000
}, defaultWebHandler)
})
server.on('upgrade', (req, socket, head) => {
proxy.ws(req, socket, head, {
hostname: 'localhost'
port: 9000
}, defaultWsHandler)
})
const app = connect()
app.use(helmet())
app.use((req, res, next) => proxy
.web(req, res, {
hostname: 'localhost'
port: 9000
}, err => {
if (err) {
next(err)
}
})
)
server.on('request', app)
server.on('request', (req, res) => {
proxy.web(req, res, {
hostname: 'localhost'
port: 9000,
onReq: (req, { headers }) => {
headers['x-forwarded-for'] = req.socket.remoteAddress
headers['x-forwarded-proto'] = req.socket.encrypted ? 'https' : 'http'
headers['x-forwarded-host'] = req.headers['host']
}
}, defaultWebHandler)
})
const http = require('follow-redirects').http
server.on('request', (req, res) => {
proxy.web(req, res, {
hostname: 'localhost'
port: 9000,
onReq: (req, options) => http.request(options)
}, defaultWebHandler)
})
server.on('request', (req, res) => {
proxy.web(req, res, {
hostname: 'localhost'
port: 9000,
onReq: (req, options) => http.request(options),
onRes: (req, res, proxyRes) => {
res.setHeader('x-powered-by', 'http2-proxy')
res.writeHead(proxyRes.statusCode, proxyRes.headers)
proxyRes.pipe(res)
}
}, defaultWebHandler)
})
const http = require('http')
const proxy = require('http2-proxy')
const createError = require('http-errors')
server.on('request', async (req, res) => {
try {
res.statusCode = null
for await (const { port, timeout, hostname } of upstream) {
if (req.aborted || finished) {
return
}
let error = null
let finished = false
let bytesWritten = 0
try {
return await proxy.web(req, res, {
port,
timeout,
hostname,
onRes: async (req, res, proxyRes) => {
if (proxyRes.statusCode >= 500) {
throw createError(proxyRes.statusCode, proxyRes.message)
}
function setHeaders () {
if (!bytesWritten) {
res.statusCode = proxyRes.statusCode
for (const [ key, value ] of Object.entries(headers)) {
res.setHeader(key, value)
}
}
}
// NOTE: At some point this will be possible
// proxyRes.pipe(res)
proxyRes
.on('data', buf => {
setHeaders()
bytesWritten += buf.length
if (!res.write(buf)) {
proxyRes.pause()
}
})
.on('end', () => {
// WORKAROUND: https://github.com/nodejs/node/pull/27984
if (!proxyRes.aborted) {
setHeaders()
res.addTrailers(proxyRes.trailers)
res.end()
// WORKAROUND: https://github.com/nodejs/node/pull/24347
finished = true
}
})
.on('close', () => {
res.off('drain', onDrain)
}))
res.on('drain', onDrain)
function onDrain () {
proxyRes.resume()
}
}
})
} catch (err) {
if (!err.statusCode) {
throw err
}
error = err
if (err.statusCode === 503) {
continue
}
if (req.method === 'HEAD' || req.method === 'GET') {
if (!bytesWritten) {
continue
}
// TODO: Retry range request
}
throw err
}
}
throw error || new createError.ServiceUnavailable()
} catch (err) {
defaultWebHandler(err)
}
}
[async] web (req, res, options[, callback])
req
: http.IncomingMessage
or http2.Http2ServerRequest
.res
: http.ServerResponse
or http2.Http2ServerResponse
.options
: See Optionscallback(err, req, res)
: Called on completion or error.See request
[async] ws (req, socket, head, options[, callback])
req
: http.IncomingMessage
.socket
: net.Socket
.head
: Buffer
.options
: See Options.callback(err, req, socket, head)
: Called on completion or error.See upgrade
options
hostname
: Proxy http.request(options)
target hostname.port
: Proxy http.request(options)
target port.protocol
: Agent protocol ('http'
or 'https'
). Defaults to 'http'
.path
: Target pathname. Defaults to req.originalUrl || req.url
.proxyTimeout
: Proxy http.request(options)
timeout.proxyName
: Proxy name used for Via header.timeout
: http.IncomingMessage
or http2.Http2ServerRequest
timeout.[async] onReq(req, options[, callback])
: Called before proxy request. If returning a truthy value it will be used as the request.
req
: http.IncomingMessage
or http2.Http2ServerRequest
options
: Options passed to http.request(options)
.callback(err)
: Called on completion or error.[async] onRes(req, resOrSocket, proxyRes[, callback])
: Called on proxy response. Writing of response must be done inside this method if provided.
req
: http.IncomingMessage
or http2.Http2ServerRequest
.resOrSocket
: For web
http.ServerResponse
or http2.Http2ServerResponse
and for ws
net.Socket
.proxyRes
: http.ServerResponse
.callback(err)
: Called on completion or error.These are some existing issues in NodeJS to keep in mind when writing proxy code.
And some pending PR's:
Some of these are further referenced in the examples.
FAQs
A simple http/2 & http/1.1 spec compliant proxy helper for Node.
The npm package http2-proxy receives a total of 21,235 weekly downloads. As such, http2-proxy popularity was classified as popular.
We found that http2-proxy demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.
Security News
Research
Socket's threat research team has detected five malicious npm packages targeting Roblox developers, deploying malware to steal credentials and personal data.