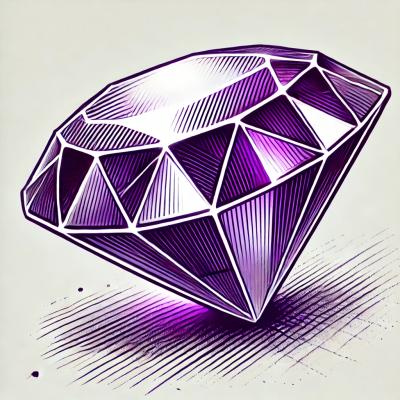
Security News
RubyGems.org Adds New Maintainer Role
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
http2-wrapper
Advanced tools
The http2-wrapper npm package is a module that provides an easy-to-use wrapper around the native HTTP/2 client and server capabilities in Node.js. It simplifies the process of making HTTP/2 requests and handling responses, as well as creating HTTP/2 servers.
Making HTTP/2 requests
This feature allows you to make HTTP/2 requests to a server. The code sample demonstrates how to perform a simple GET request using the http2-wrapper.
const http2wrapper = require('http2-wrapper');
const options = {
hostname: 'example.com',
protocol: 'https:',
path: '/',
method: 'GET'
};
http2wrapper.request(options, (res) => {
console.log(`Status Code: ${res.statusCode}`);
res.on('data', (chunk) => {
console.log(chunk);
});
}).end();
Creating HTTP/2 servers
This feature allows you to create an HTTP/2 server. The code sample shows how to set up a simple HTTP/2 server that responds with 'Hello World' to all requests.
const http2wrapper = require('http2-wrapper');
const http2 = require('http2');
const server = http2.createSecureServer({
key: fs.readFileSync('server-key.pem'),
cert: fs.readFileSync('server-cert.pem')
});
server.on('stream', (stream, headers) => {
stream.respond({
'content-type': 'text/html',
':status': 200
});
stream.end('<h1>Hello World</h1>');
});
http2wrapper.createServer(server).listen(8443);
The 'spdy' package is an HTTP/2 and SPDY protocol client and server implementation for Node.js. It provides similar functionalities to http2-wrapper but also includes support for SPDY, which is a now-deprecated protocol that was a precursor to HTTP/2.
While 'node-fetch' is primarily a light-weight module that brings window.fetch to Node.js, it can be used to make HTTP/2 requests when combined with the built-in http2 module in Node.js. It does not provide server capabilities like http2-wrapper.
HTTP2 client, just with the familiar
https
API
This package was created to support HTTP2 without the need to rewrite your code.
I recommend adapting to the http2
module if possible - it's much simpler to use and has many cool features! Well, it doesn't have agents yet...
Tip: http2-wrapper
is very useful when you rely on other modules that use the HTTP1 API and you want to support HTTP2.
$ npm install http2-wrapper
$ yarn add http2-wrapper
It's best to run http2-wrapper
under the latest version of Node. It provides the best stability.
const http2 = require('http2-wrapper');
const options = {
hostname: 'nghttp2.org',
protocol: 'https:',
path: '/httpbin/post',
method: 'POST',
headers: {
'content-length': 6
}
};
const request = http2.request(options, response => {
console.log('statusCode:', response.statusCode);
console.log('headers:', response.headers);
const body = [];
response.on('data', chunk => {
body.push(chunk);
});
response.on('end', () => {
console.log('body:', Buffer.concat(body).toString());
});
});
request.on('error', e => console.error(e));
request.write('123');
request.end('456');
// statusCode: 200
// headers: [Object: null prototype] {
// ':status': 200,
// date: 'Fri, 27 Sep 2019 19:45:46 GMT',
// 'content-type': 'application/json',
// 'access-control-allow-origin': '*',
// 'access-control-allow-credentials': 'true',
// 'content-length': '239',
// 'x-backend-header-rtt': '0.002516',
// 'strict-transport-security': 'max-age=31536000',
// server: 'nghttpx',
// via: '1.1 nghttpx',
// 'alt-svc': 'h3-23=":4433"; ma=3600',
// 'x-frame-options': 'SAMEORIGIN',
// 'x-xss-protection': '1; mode=block',
// 'x-content-type-options': 'nosniff'
// }
// body: {
// "args": {},
// "data": "123456",
// "files": {},
// "form": {},
// "headers": {
// "Content-Length": "6",
// "Host": "nghttp2.org"
// },
// "json": 123456,
// "origin": "xxx.xxx.xxx.xxx",
// "url": "https://nghttp2.org/httpbin/post"
// }
Note: The session
option was renamed to tlsSession
for better readability.
Performs ALPN negotiation.
Returns a Promise giving proper ClientRequest
instance (depending on the ALPN).
Note: The agent
option represents an object with http
, https
and http2
properties.
const http2 = require('http2-wrapper');
const options = {
hostname: 'httpbin.org',
protocol: 'http:', // Note the `http:` protocol here
path: '/post',
method: 'POST',
headers: {
'content-length': 6
}
};
(async () => {
try {
const request = await http2.auto(options, response => {
console.log('statusCode:', response.statusCode);
console.log('headers:', response.headers);
const body = [];
response.on('data', chunk => body.push(chunk));
response.on('end', () => {
console.log('body:', Buffer.concat(body).toString());
});
});
request.on('error', console.error);
request.write('123');
request.end('456');
} catch (error) {
console.error(error);
}
})();
// statusCode: 200
// headers: { connection: 'close',
// server: 'gunicorn/19.9.0',
// date: 'Sat, 15 Dec 2018 18:19:32 GMT',
// 'content-type': 'application/json',
// 'content-length': '259',
// 'access-control-allow-origin': '*',
// 'access-control-allow-credentials': 'true',
// via: '1.1 vegur' }
// body: {
// "args": {},
// "data": "123456",
// "files": {},
// "form": {},
// "headers": {
// "Connection": "close",
// "Content-Length": "6",
// "Host": "httpbin.org"
// },
// "json": 123456,
// "origin": "xxx.xxx.xxx.xxx",
// "url": "http://httpbin.org/post"
// }
An instance of quick-lru
used for ALPN cache.
There is a maximum of 100 entries. You can modify the limit through protocolCache.maxSize
- note that the change will be visible globally.
Same as https.request
.
Type: boolean
Default: true
If set to true
, it will try to connect to the server before sending the request.
Type: Http2Session
The session used to make the actual request. If none provided, it will use options.agent
.
Same as https.get
.
Same as https.ClientRequest
.
Same as https.IncomingMessage
.
Note: this is not compatible with the classic http.Agent
.
Usage example:
const http2 = require('http2-wrapper');
class MyAgent extends http2.Agent {
createConnection(origin, options) {
console.log(`Connecting to ${http2.Agent.normalizeOrigin(origin)}`);
return http2.Agent.connect(origin, options);
}
}
http2.get({
hostname: 'google.com',
agent: new MyAgent()
}, res => {
res.on('data', chunk => console.log(`Received chunk of ${chunk.length} bytes`));
});
Each option is assigned to each Agent
instance and can be changed later.
Type: number
Default: 60000
If there's no activity after timeout
milliseconds, the session will be closed.
Type: number
Default: Infinity
The maximum amount of sessions per origin.
Type: number
Default: 1
The maximum amount of free sessions per origin.
Type: number
Default: 100
The maximum amount of cached TLS sessions.
Returns a string representing the origin of the URL.
Type: object
Default: {enablePush: false}
Settings used by the current agent instance.
Returns a string representing normalized options.
Agent.normalizeOptions({servername: 'example.com'});
// => ':example.com'
Type: string
URL
object
An origin used to create new session.
Type: object
The options used to create new session.
Returns a Promise giving free Http2Session
. If no free sessions are found, a new one is created.
Type: object
{
reject: error => void,
resolve: session => void
}
If the listener
argument is present, the Promise will resolve immediately. It will use the resolve
function to pass the session.
Returns a Promise giving Http2Stream
.
Returns a new TLSSocket
. It defaults to Agent.connect(origin, options)
.
Makes an attempt to close free sessions. Only sessions with 0 concurrent streams will be closed.
Destroys all sessions.
agent.on('session', session => {
// A new session has been created by the Agent.
});
CPU: Intel i7-7700k (governor: performance)
Server: H2O v2.2.5 h2o.conf
Node: v13.8.0
auto
means http2wrapper.auto
.
http2-wrapper x 12,417 ops/sec ±3.72% (83 runs sampled)
http2-wrapper - preconfigured session x 14,517 ops/sec ±1.39% (83 runs sampled)
http2-wrapper - auto x 11,373 ops/sec ±3.17% (84 runs sampled)
http2 x 16,172 ops/sec ±1.21% (85 runs sampled)
https - auto - keepalive x 13,251 ops/sec ±3.84% (79 runs sampled)
https - keepalive x 13,158 ops/sec ±2.88% (78 runs sampled)
https x 1,618 ops/sec ±2.07% (82 runs sampled)
http x 5,922 ops/sec ±2.87% (79 runs sampled)
Fastest is http2
http2-wrapper
:
http2
https - keepalive
http
http2-wrapper - preconfigured session
:
http2
https - keepalive
http
http2-wrapper - auto
:
http2
https - keepalive
http
https - auto - keepalive
:
http2
https - keepalive
http
got
- Simplified HTTP requestsMIT
FAQs
HTTP2 client, just with the familiar `https` API
The npm package http2-wrapper receives a total of 4,782,192 weekly downloads. As such, http2-wrapper popularity was classified as popular.
We found that http2-wrapper demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.
Security News
Research
Socket's threat research team has detected five malicious npm packages targeting Roblox developers, deploying malware to steal credentials and personal data.