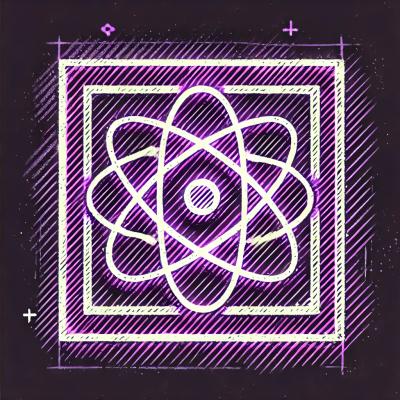
Security News
Create React App Officially Deprecated Amid React 19 Compatibility Issues
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
httpinvoke
Advanced tools
A no-dependencies HTTP client library for browsers and Node.js with a promise-based or Node.js-style callback-based API to progress events, text and binary file upload and download, partial response body, request and response headers, status code.
A no-dependencies HTTP client library for browsers and Node.js with a promise-based or Node.js-style callback-based API to progress events, text and binary file upload and download, partial response body, request and response headers, status code.
Install manually by adding to your HTML file:
<script src="/path/to/httpinvoke/httpinvoke-browser.js"></script>
Install with npm:
$ npm install --save httpinvoke
Install with component:
$ component install jakutis/httpinvoke
Install with bower:
$ bower install --save httpinvoke
var httpinvoke = require('httpinvoke');
httpinvoke('http://example.org', 'GET', function(err, body, statusCode, headers) {
if(err) {
return console.log('Failure', err);
}
console.log('Success', body, statusCode, headers);
});
var httpinvoke = require('httpinvoke');
httpinvoke('http://example.org', 'GET').then(function(res) {
console.log('Success', res.body, res.statusCode, res.headers);
}, function(err) {
console.log('Failure', err);
});
httpinvoke('http://example.org', {
partialOutputMode: 'chunked',
outputType: 'bytearray'
}).then(function(res) {
console.log('Success', res.body, res.statusCode, res.headers);
}, function(err) {
console.log('Error occurred', err);
}, function(progress) {
if(progress.type === 'upload') {
// total and current are always defined
console.log('progress: ' + (progress.total - progress.current) + ' bytes left to upload');
} else if(progress.type === 'download') {
var partialinfo = ' (received chunk of ' + progress.partial.length + ' bytes)';
if(typeof progress.total === 'undefined') {
console.log('progress: ' + progress.current + ' bytes downloaded' + partialinfo);
} else {
console.log('progress: ' + (progress.total - progress.current) + ' bytes left to download' + partialinfo);
}
} else if(progress.type === 'headers') {
console.log('progress: received response with status code ' + progress.statusCode + ' and headers: ', progress.headers);
}
});
var httpinvoke = require('httpinvoke');
var book = {
content: 'Hello World',
comment: 'initial version'
};
// convert the json object to application/x-www-form-urlencoded format string
var encodedBook = Object.keys(book).map(function(key) {
return encodeURIComponent(key) + '=' + encodeURIComponent(book[key]);
}).join('&');
// upload to server
httpinvoke('http://example.org', 'POST', {
headers: {
// remove this header if doing a cross-domain request
// or add a 'Content-Type' to 'Access-Control-Allow-Headers' server-side response header
'Content-Type': 'application/x-www-form-urlencoded'
},
input: encodedBook
}, function(err) {
if(err) {
return console.log('Failure', err);
}
console.log('Success');
});
var httpinvoke = require('httpinvoke');
var converters = {
'text json': JSON.parse,
'json text': JSON.stringify
};
httpinvoke('https://bower-component-list.herokuapp.com/', 'GET', {
outputType: 'json',
converters: converters
}).then(function(response) {
console.log('There are ' + response.body.length + ' bower packages.');
return httpinvoke('http://server.cors-api.appspot.com/server?id=9285649&enable=true&status=200&credentials=false&methods=POST', 'POST', {
input: response.body,
inputType: 'json',
converters: converters
});
}, function(err) {
console.log('Error receiving package list', err);
}, function(progress) {
console.log('Receiving package list progress', progress);
}).then(function(response) {
console.log('Uploading finished', response);
}, function(err) {
console.log('Error sending package list', err);
}, function(progress) {
console.log('Sending package list progress', progress);
});
var clarinet = require('clarinet');
var httpinvoke = require('httpinvoke');
var parser = clarinet.parser();
var lastKey = null;
parser.onvalue = function(v) {
if(lastKey === 'name') {
console.log('component', v);
}
};
parser.onopenobject = function(key) {
lastKey = key;
};
parser.onkey = function(key) {
lastKey = key;
};
// try with slow internet connection
httpinvoke('https://bower-component-list.herokuapp.com/', {
partialOutputMode: 'chunked'
}).then(function(res) {
parser.close();
console.log('OK, in total there are ' + JSON.parse(res.body).length + ' bower components');
}, function(err) {
console.log('Error occurred', err);
}, function(progress) {
if(progress.type === 'download') {
console.log('received chunk of length=' + progress.partial.length);
parser.write(progress.partial);
}
});
var abort = httpinvoke(url, method, options, cb)
Any one, two or three arguments can be skipped, except the url.
then()
method) that receives all the same events as the callbacks - uploading, downloading, gotStatus and finished - see an example. When invoked as a function, it immediately calls the "finished" callback with an Error. If "finished" callback is already called before the "abort", nothing happens."http://example.org/"
."HEAD"
, "GET"
, "PATCH"
, "POST"
, "PUT"
, "DELETE"
.See the Examples section for all the options being used. All options are optional.
"disabled"
(default, downloading will not receive the partial argument), "chunked"
(the received value will be the latest chunk), "joined"
(the received value will be the entire partial body).true
. Defaults to the value of anonymousByDefault feature flag. If specified, and false
, then overrides corsCredentials (makes it true
).true
. Defaults to the value of systemByDefault feature flag. If specified, and true
, then overrides anonymous (makes it true
)."timeout"
error,"upload timeout"
error;
0. a number (greater than 0 and less than 1073741824) for maximum duration in milliseconds between gotStatus call and finished call, if it timeouts - finished must be called with "download timeout"
error."bytearray"
or a custom outputType will be converted from bytearray), or undefined if partialOutputMode option is set to "disabled"
."HEAD"
),
* a string, if outputType is "text"
,
* a bytearray - instance of Uint8Array or instance of Buffer or instance of Array that has elements of type "number" with values ranging from 0
to 255
- if outputType is "bytearray"
,
* any value - if outputType is neither "bytearray"
, nor "text"
, i.e. a converter has been called.
0. status is:
* undefined, if err is not null or no correct value is available,
* otherwise, a number for an HTTP response status code, correct value.
0. headers is:
* undefined, if err is not null,
* otherwise, an object for HTTP response headers. Keys are lower-cased header names, values are strings."text"
(default), "bytearray"
or a custom value that has corresponding converters."auto"
(default), "bytearray"
, "text"
, "formdata"
or a custom value that has corresponding converters. If not "auto"
, input must be not be undefined."auto"
and request headers does not have Content-Type
,"text"
or "auto"
,0
to 255
- if inputType is "bytearray"
or "auto"
."formdata"
or "auto"
,"bytearray"
or "text"
. Example: {"json text": JSON.stringify, "text json": JSON.parse}
. If you use custom inputType, then there must be at least one converter from that type to "text"
or "bytearray"
, and the other way around for outputType.false
.Access-Control-Allow-Headers
server-side header, see dummyserver.js
for an example of server-side part of the workaround implementation.The callbacks are called in this strict sequence:
hook is a function to hook into how httpinvoke works. It leaves the current instance of httpinvoke untouched. It returns a new instance of httpinvoke with a new hook, and all the hooks inherited from the old httpinvoke. It takes these arguments:
// a new httpinvoke with a hook to fail on 4xx and 5xx statuses, just like jQuery
httpinvoke = httpinvoke.hook('finished', function(err, output, statusCode, headers) {
if(err) {
return arguments;
}
if(typeof statusCode === 'undefined') {
return [new Error('Server or client error - undefined HTTP status'), output, statusCode, headers];
}
if(statusCode >= 400 && statusCode <= 599) {
return [new Error('Server or client error - HTTP status ' + statusCode), output, statusCode, headers];
}
return arguments;
});
// err will be set; output, status, headers are unchanged
httpinvoke('http://example.org/foobar', function(err, output, statusCode, headers) {
console.log(err, output, statusCode, headers);
});
// will be rejected (prints 'Failure with status=404')
httpinvoke('http://example.org/foobar').then(function(res) {
console.log('Success', res.body, res.statusCode, res.headers);
}, function(err, res) {
console.log('Failure with status=', res.statusCode);
});
There are feature flags to be queried for platform-specific features.
if(httpinvoke.cors) {
console.log('Cross-Origin Resource Sharing support is available!');
}
"bytearray"
is not supported (though "formdata"
may be supported, check for window.FormData constructor on your browser)"PATCH"
method is supported"DELETE"
method"HEAD"
method"PATCH"
method"PUT"
method"text"
"/foo"
, "./bar"
, "foobar"
) and protocol-relative URLs (e.g. "//example.org/foo"
) are supportedThe finished callback will be called with an instance of Error only when strictly either one of these things happen:
"abort"
);"network error"
) - this can happen due to various network errors, server response sending or request receiving errors, or simply an unsupported status code - e.g. Firefox 3.0 ends up here after a status 408 response;"upload timeout"
);"download timeout"
);"timeout"
);"Error code #%. See https://github.com/jakutis/httpinvoke#error-codes"
) - calling options validation failed or a feature that is not supported by the platform was used (various error messages are passed, e.g. "Unsupported method TRACE"). See error codes.So generally, finishing with an error means that a response has not been received.
Please note that a request can finish successfully, with an err set to null
, but also with an undefined status, undefined output and an empty headers object - generally status is defined at all times, but some older browsers provide status code only for 2XX responses - e.g. Opera 11.50.
After cloning the repo, and on each modification of src
folder, you have to run npm run compile
.
To test for NodeJS functionality run npm run test-node
.
To test for web browser functionality run npm run test-browser
, copy the URL link that is displayed in console and open it in any web browser.
npm test
npm run compile
release X.X.X
commit created and tagged as X.X.X
npm publish
FAQs
A no-dependencies HTTP client library for browsers and Node.js with a promise-based or Node.js-style callback-based API to progress events, text and binary file upload and download, partial response body, request and response headers, status code.
The npm package httpinvoke receives a total of 47 weekly downloads. As such, httpinvoke popularity was classified as not popular.
We found that httpinvoke demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
Security News
Oracle seeks to dismiss fraud claims in the JavaScript trademark dispute, delaying the case and avoiding questions about its right to the name.
Security News
The Linux Foundation is warning open source developers that compliance with global sanctions is mandatory, highlighting legal risks and restrictions on contributions.