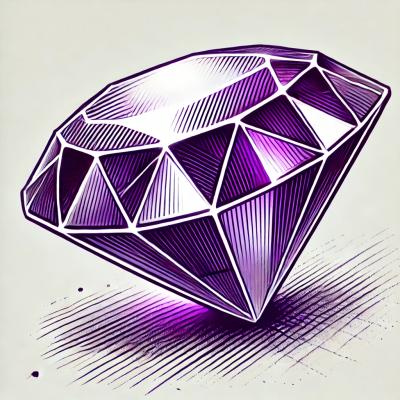
Security News
RubyGems.org Adds New Maintainer Role
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
humanize-duration
Advanced tools
The humanize-duration npm package is used to convert durations, given in milliseconds, into human-readable strings. It is useful for displaying time durations in a more understandable format for users.
Basic Usage
This feature allows you to convert a duration in milliseconds to a human-readable string. In this example, 3000 milliseconds is converted to '3 seconds'.
const humanizeDuration = require('humanize-duration');
console.log(humanizeDuration(3000)); // '3 seconds'
Custom Units
This feature allows you to specify custom units for the duration. In this example, 3600000 milliseconds is converted to '1 hour' using hours and minutes as units.
const humanizeDuration = require('humanize-duration');
console.log(humanizeDuration(3600000, { units: ['h', 'm'] })); // '1 hour'
Language Support
This feature allows you to specify the language for the output string. In this example, 3000 milliseconds is converted to '3 segundos' in Spanish.
const humanizeDuration = require('humanize-duration');
console.log(humanizeDuration(3000, { language: 'es' })); // '3 segundos'
Round
This feature allows you to round the duration to the nearest unit. In this example, 1234567 milliseconds is rounded to '20 minutes'.
const humanizeDuration = require('humanize-duration');
console.log(humanizeDuration(1234567, { round: true })); // '20 minutes'
Largest
This feature allows you to limit the number of units in the output string. In this example, 1234567890 milliseconds is converted to '14 days, 6 hours' with a limit of 2 units.
const humanizeDuration = require('humanize-duration');
console.log(humanizeDuration(1234567890, { largest: 2 })); // '14 days, 6 hours'
Moment.js is a comprehensive library for parsing, validating, manipulating, and formatting dates and times in JavaScript. It includes functionality for humanizing durations, but it is more heavyweight compared to humanize-duration.
date-fns is a modern JavaScript date utility library that provides a variety of functions for working with dates, including humanizing durations. It is modular and tree-shakeable, making it a lighter alternative to Moment.js.
Luxon is a modern JavaScript library for working with dates and times, created by one of the Moment.js developers. It offers a more modern API and includes features for humanizing durations, similar to humanize-duration.
I have the time in milliseconds and I want it to become "30 minutes" or "3 days, 1 hour". Enter Humanize Duration!
This library is in maintenance mode. New languages and bug fixes will be added but no new features will be. If you're interested in helping out by taking over the project, please see this GitHub issue.
This package is available as humanize-duration on npm and Bower. You can also include the JavaScript file in the browser.
In the browser:
<script src="humanize-duration.js"></script>
<script>
humanizeDuration(12000)
</script>
In Node (or Browserify or Webpack or anywhere with CommonJS):
var humanizeDuration = require('humanize-duration')
humanizeDuration(12000) // '12 seconds'
By default, Humanize Duration will humanize down to the second, and will return a decimal for the smallest unit. It will humanize in English by default.
humanizeDuration(3000) // '3 seconds'
humanizeDuration(2250) // '2.25 seconds'
humanizeDuration(97320000) // '1 day, 3 hours, 2 minutes'
You can change the settings by passing options as the second argument:
language
Language for unit display (accepts an ISO 639-1 code from one of the supported languages).
humanizeDuration(3000, { language: 'es' }) // '3 segundos'
humanizeDuration(5000, { language: 'ko' }) // '5 초'
delimiter
String to display between the previous unit and the next value.
humanizeDuration(22140000, { delimiter: ' and ' }) // '6 hours and 9 minutes'
humanizeDuration(22140000, { delimiter: '--' }) // '6 hours--9 minutes'
spacer
String to display between each value and unit.
humanizeDuration(260040000, { spacer: ' whole ' }) // '3 whole days, 14 whole minutes'
humanizeDuration(260040000, { spacer: '' }) // '3days, 14minutes'
largest
Number representing the maximum number of units to display for the duration.
humanizeDuration(1000000000000) // '31 years, 8 months, 1 week, 19 hours, 46 minutes, 40 seconds'
humanizeDuration(1000000000000, { largest: 2 }) // '31 years, 8 month'
units
Array of strings to define which units are used to display the duration (if needed). Can be one, or a combination of any, of the following: ['y', 'mo', 'w', 'd', 'h', 'm', 's', 'ms']
humanizeDuration(3600000, { units: ['h'] }) // '1 hour'
humanizeDuration(3600000, { units: ['m'] }) // '60 minutes'
humanizeDuration(3600000, { units: ['d', 'h'] }) // '1 hour'
round
Boolean value. Use true
to round the smallest unit displayed (can be combined with largest
and units
).
humanizeDuration(1200) // '1.2 seconds'
humanizeDuration(1200, { round: true }) // '1 second'
humanizeDuration(1600, { round: true }) // '2 seconds'
decimal
String to substitute for the decimal point in a decimal fraction.
humanizeDuration(1200) // '1.2 seconds'
humanizeDuration(1200, { decimal: ' point ' }) // '1 point 2 seconds'
conjunction
String to include before the final unit. You can also set serialComma
to false
to eliminate the final comma.
humanizeDuration(22140000, { conjunction: ' and ' }) // '6 hours and 9 minutes'
humanizeDuration(22141000, { conjunction: ' and ' }) // '6 hours, 9 minutes, and 1 second'
humanizeDuration(22140000, { conjunction: ' and ', serialComma: false }) // '6 hours and 9 minutes'
humanizeDuration(22141000, { conjunction: ' and ', serialComma: false }) // '6 hours, 9 minutes and 1 second'
unitMeasures
Customize the value used to calculate each unit of time.
humanizeDuration(400) // '0.4 seconds'
humanizeDuration(400, { // '1 year, 1 month, 5 days'
unitMeasures: {
y: 365,
mo: 30,
w: 7,
d: 1
}
})
Combined example
humanizeDuration(3602000, {
language: 'es',
round: true,
spacer: ' glorioso ',
units: ['m']
}) // '60 glorioso minutos'
If you find yourself setting same options over and over again, you can create a humanizer that changes the defaults, which you can still override later.
var spanishHumanizer = humanizeDuration.humanizer({
language: 'es',
units: ['y', 'mo', 'd']
})
spanishHumanizer(71177400000) // '2 años, 3 meses, 2 días'
spanishHumanizer(71177400000, { units: ['d', 'h'] }) // '823 días, 19.5 horas'
You can also add new languages to humanizers. For example:
var shortEnglishHumanizer = humanizeDuration.humanizer({
language: 'shortEn',
languages: {
shortEn: {
y: function() { return 'y' },
mo: function() { return 'mo' },
w: function() { return 'w' },
d: function() { return 'd' },
h: function() { return 'h' },
m: function() { return 'm' },
s: function() { return 's' },
ms: function() { return 'ms' },
}
}
})
shortEnglishHumanizer(15600000) // '4 h, 20 m'
You can also add languages after initializing:
var humanizer = humanizeDuration.humanizer()
humanizer.languages.shortEn = {
y: function(c) { return c + 'y' },
// ...
Internally, the main humanizeDuration
function is just a wrapper around a humanizer.
Humanize Duration supports the following languages:
Language | Code |
---|---|
Arabic | ar |
Catalan | ca |
Chinese, simplified | zh_CN |
Chinese, traditional | zh_TW |
Czech | cs |
Danish | da |
Dutch | nl |
English | en |
Finnish | fi |
French | fr |
German | de |
Greek | gr |
Hungarian | hu |
Icelandic | is |
Indonesian | id |
Italian | it |
Japanese | ja |
Korean | ko |
Lithuanian | lt |
Malay | ms |
Norwegian | no |
Polish | pl |
Portuguese | pt |
Russian | ru |
Spanish | es |
Swedish | sv |
Turkish | tr |
Ukrainian | uk |
Vietnamese | vi |
For a list of supported languages, you can use the getSupportedLanguages
function.
humanizeDuration.getSupportedLanguages()
// ['ar', 'ca', 'da', 'de' ...]
This function won't return any new languages you define; it will only return the defaults supported by the library.
Lovingly made by Evan Hahn with help from:
Licensed under the permissive Unlicense. Enjoy!
3.10.1 / 2017-07-21
FAQs
Convert millisecond durations to English and many other languages.
The npm package humanize-duration receives a total of 478,794 weekly downloads. As such, humanize-duration popularity was classified as popular.
We found that humanize-duration demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.
Security News
Research
Socket's threat research team has detected five malicious npm packages targeting Roblox developers, deploying malware to steal credentials and personal data.