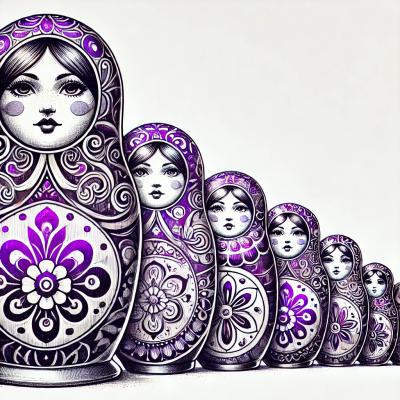
Research
Security News
Threat Actor Exposes Playbook for Exploiting npm to Build Blockchain-Powered Botnets
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
humanize-plus
Advanced tools
A simple utility library for making the web more humane.
Humanize Plus is available via node package manager.
npm install humanize-plus
Or download the minified version or the full version.
In your web page:
<script src="public/humanize.min.js"></script>
<script>
var capitalized = Humanize.capitalize("ten tiny ducklings.")
// "Ten tiny ducklings."
</script>
In your node package.json:
"dependencies": {
"humanize-plus": "1.3.x"
}
Formats a number to a human-readable string. Localize by overriding the precision, thousand and decimal arguments.
Humanize.formatNumber(123456789, 2)
// "123,456,789.00"
Converts an integer to a string containing commas every three digits.
Humanize.intcomma(123456789)
// "123,456,789"
Converts a large integer to a friendly text representation. This method is now a thin wrapper around compactInteger
Humanize.intword(num, ch, de) === Humanize(num, de)
Humanize.intword(123456789, 'nopnopnopnop', 1)
// "123.5M"
Humanize.intword(123456789, 'this is a nop', 3)
// "123.457M"
Humanize.intword(10, 'still a nop', 1)
// "10.0"
Converts an integer into its most compact representation.
Humanize.compactInteger(123456789, 1)
// "123.5M"
// Switch to scientific notation for trillons, because no one knows those abbreviations.
Humanize.compactInteger(-7832186132456328967, 4)
// "-7.8322x10^18"
Humanize.compactInteger(-100, 2)
// "-100.00"
Converts an integer to its ordinal as a string.
Humanize.ordinal(22)
// "22nd"
Interprets numbers as occurences. Also accepts an optional array/map of overrides.
for (i=0; i<5; i++) {
Humanize.times(i, {"4": "too many"});
// Bonus!
if (i === 1) {
Humanize.times(1.1);
}
}
// never
// once
// 1.1 times
// twice
// 3 times
// too many times
Formats the value like a 'human-readable' file size (i.e. '13 KB', '4.1 MB', '102 bytes', etc).
Humanize.filesize(1024 * 20)
// "20 Kb"
Humanize.filesize(1024 * 2000)
// "1.95 Mb"
Humanize.filesize(Math.pow(1000, 4))
// "931.32 Gb"
Returns the plural version of a given word if the value is not 1. The default suffix is 's'.
Humanize.pluralize(1, "duck")
// "duck"
Humanize.pluralize(3, "duck")
// "ducks"
Humanize.pluralize(3, "duck", "duckies")
// "duckies"
Truncates a string if it is longer than the specified number of characters. Truncated strings will end with a translatable ellipsis sequence ("…").
Humanize.truncate('long text is good for you')
// "long text is good for you"
Humanize.truncate('long text is good for you', 19)
// "long text is goo..."
Humanize.truncate('long text is good for you', 19, '... etc')
// "long text is... etc"
Truncates a string after a certain number of words.
Humanize.truncatewords('long text is good for you', 5)
// "long text is good for ..."
Converts a list of items to a human readable string with an optional limit.
items = ['apple', 'orange', 'banana', 'pear', 'pineapple']
Humanize.oxford(items)
// "apple, orange, banana, pear, and pineapple"
Humanize.oxford(items, 3)
// "apple, orange, banana, and 2 others"
// Pluralizes properly too!
Humanize.oxford(items, 4)
// "apple, orange, banana, and 1 other"
Humanize.oxford(items, 3, "and some other fruits")
// "apple, orange, banana, and some other fruits"
Describes how many times an item appears in a list
aznPics = [
'http://24.media.tumblr.com/77082543cb69af56ede38a0cdb2511d0/tumblr_mh96olWPLv1r8k4ywo1_1280.jpg',
'http://25.media.tumblr.com/3e2d318be34d5ef8f86a612cd1d795ff/tumblr_mhbhb96t3z1r8k4ywo1_1280.jpg',
'http://24.media.tumblr.com/8c5a052e33c27c784514e1b124b383a1/tumblr_mhexaqrk0w1r8k4ywo1_1280.jpg'
]
bigfootPics = []
"Asians " + Humanize.frequency(aznPics, "took pictures of food")
// "Asians took pictures of food 3 times"
"Bigfoot " + Humanize.frequency(bigfootPics, "took pictures of food")
// "Bigfoot never took pictures of food"
Matches a pace (value and interval) with a logical time frame. Very useful for slow paces.
second = 1000
week = 6.048e8
decade = 3.156e11
Humanize.pace(1.5, second, "heartbeat")
// Approximately 2 heartbeats per second
Humanize.pace(4, week)
// Approximately 4 times per week
Humanize.pace(1, decade, "life crisis")
// Less than 1 life crisis per week
Flexible conversion of <br/>
tags to newlines and vice versa.
// Use your imagination
Capitalizes the first letter in a string.
Humanize.capitalize("some boring string")
// "Some boring string"
Captializes the first letter of every word in a string.
Humanize.capitalizeAll("some boring string")
// "Some Boring String"
Intelligently capitalizes eligible words in a string and normalizes internal whitespace.
Humanize.titlecase("some of a boring string")
// "Some of a Boring String"
Humanize.titlecase("cool the iTunes cake, O'Malley!")
// "Cool the iTunes Cake, O'Malley!"
Fixes binary rounding issues (eg. (0.615).toFixed(2) === "0.61").
Humanize.toFixed(0.615, 2)
// "0.61"
Ensures precision value is a positive integer.
Humanize.normalizePrecision(-232.231)
// 232
Please don't edit files in the public
subdirectory as they are generated via grunt. You'll find source code in the coffee
subdirectory!
This assumes you have node.js and npm installed already.
npm install
to install the project's dependencies.And that's it!
Once grunt is installed, just run "grunt" in the root directory of the project to compile the CoffeeScript files into the public/ subdirectory.
In order for the test task to work properly, PhantomJS must be installed and in the system PATH (if you can run "phantomjs" at the command line, this task should work).
Unfortunately, PhantomJS cannot be installed automatically via npm or grunt, so you need to install it yourself. There are a number of ways to install PhantomJS.
Note that the phantomjs
executable needs to be in the system PATH
for grunt to see it.
Copyright (c) 2013 HubSpotDev Licensed under the MIT license.
FAQs
A simple utility library for making the web more humane.
The npm package humanize-plus receives a total of 34,688 weekly downloads. As such, humanize-plus popularity was classified as popular.
We found that humanize-plus demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
Security News
NVD’s backlog surpasses 20,000 CVEs as analysis slows and NIST announces new system updates to address ongoing delays.
Security News
Research
A malicious npm package disguised as a WhatsApp client is exploiting authentication flows with a remote kill switch to exfiltrate data and destroy files.