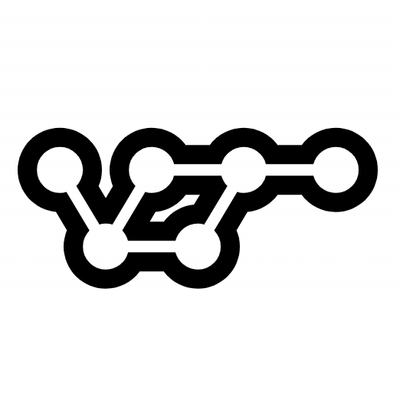
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
imagecachejs
Advanced tools
Imagecache is an express middleware that lets you manipulate images and then cache them for quick retrieval.
Imagecache is an express middleware that lets you manipulate images and then cache them for quick retrieval.
npm install --save imagecachejs
It's easy to just add imagecache to your application
import path from 'path'
import express from 'express'
import imagecache from 'imagecachejs'
const app = express()
app.use(express.static('./public'))
app.use('/imagecache', imagecache({
destination: path.resolve('cached'),
sources: [
'http://localhost:8080'
]
}))
app.listen(8080, function () {
console.log('Example app listening on port 8080')
})
Once your server is up and running, you can invoke an image transformation using one of the following two syntaxes:
# query string syntax
http://localhost:3000/imagecache/{imagePath}?{queryString}
# pathname syntax
http://localhost:3000/imagecache/{queryString}/{imagePath}
The pathname syntax is usually preferable because it is something that a CDN or caching proxy can easily request and save using the full pathname as the cache key
Increase or decrease the brightness
![]() | ![]() |
http://localhost:3000/imagecache/bri=50/images/kitten.png |
![]() | ![]() |
http://localhost:3000/imagecache/bri=-50/images/kitten.png |
Increase or decrease the brightness
![]() | ![]() |
http://localhost:3000/imagecache/con=50/images/kitten.png |
![]() | ![]() |
http://localhost:3000/imagecache/con=-50/images/kitten.png |
Rotate the hue of an image with a value between -360 and 360 degrees
![]() | ![]() |
http://localhost:3000/imagecache/hue=90/images/kitten.png |
![]() | ![]() |
http://localhost:3000/imagecache/hue=-90/images/kitten.png |
Increase or decrease the saturation of an image with an amount between -100% and 100%
![]() | ![]() |
http://localhost:3000/imagecache/sat=50/images/kitten.png |
![]() | ![]() |
http://localhost:3000/imagecache/sat=-50/images/kitten.png |
Tint the image with a layer of white with a opacity value between 1 and 100
![]() | ![]() |
http://localhost:3000/imagecache/tint=50/images/kitten.png |
Shade the image with a layer of black with a opacity value between 1 and 100
![]() | ![]() |
http://localhost:3000/imagecache/shade=50/images/kitten.png |
Tint the image with a layer of any color with a opacity value between 1 and 100
![]() | ![]() |
http://localhost:3000/imagecache/mix=red,50/images/kitten.png |
![]() | ![]() |
http://localhost:3000/imagecache/mix=blue,50/images/kitten.png |
Invert the colors of the image
![]() | ![]() |
http://localhost:3000/imagecache/invert=true/images/kitten.png |
Blur image with a radius
![]() | ![]() |
http://localhost:3000/imagecache/blur=15/images/kitten.png |
Flip the image horizontally, vertically, or both
![]() | ![]() |
http://localhost:3000/imagecache/flip=h/images/kitten.png |
![]() | ![]() |
http://localhost:3000/imagecache/flip=v/images/kitten.png |
![]() | ![]() |
http://localhost:3000/imagecache/flip=vh/images/kitten.png |
Rotate image and then crop to largest possible rectangle with same aspect ratio
![]() | ![]() |
http://localhost:3000/imagecache/rot=45/images/kitten.png |
Put x pixels of padding around the image
![]() | ![]() |
http://localhost:3000/imagecache/pad=50/images/kitten.png |
Draw an x pixel thick border around the image
![]() | ![]() |
http://localhost:3000/imagecache/border=50,red/images/kitten.png |
Crop the image using a reactangle in the format "x,y,w,h"
![]() | ![]() |
http://localhost:3000/imagecache/crop=100,100,400,200/images/kitten.png |
Resize the image
http://localhost:3000/imagecache/w=500/images/kitten.png
http://localhost:3000/imagecache/w=200&dpi=2/images/kitten.png
http://localhost:3000/imagecache/h=250/images/kitten.png
http://localhost:3000/imagecache/w=300&h=300/images/kitten.png
FAQs
Unknown package
The npm package imagecachejs receives a total of 2 weekly downloads. As such, imagecachejs popularity was classified as not popular.
We found that imagecachejs demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.