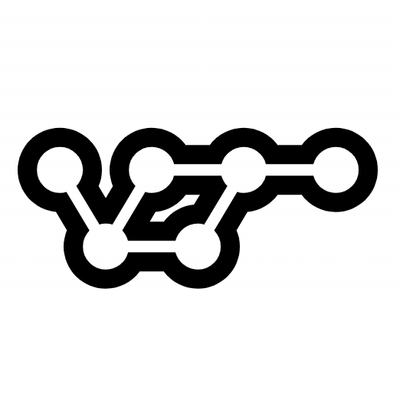
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
imagecachejs
Advanced tools
Imagecache is a express middleware that lets you manipulate images and then cache them for quick retrieval.
Imagecache is a express middleware that lets you manipulate images and then cache them for quick retrieval.
npm install --save imagecachejs
It's easy to just add imagecache to your application
import path from 'path'
import express from 'express'
import imagecache from 'imagecachejs'
const app = express()
app.use(express.static('./public'))
app.use('/imagecache', imagecache({
destination: path.resolve('cached'),
sources: [
'http://localhost:8080'
]
}))
app.listen(8080, function () {
console.log('Example app listening on port 8080')
})
Increase or decrease the brightness
![]() | ![]() |
http://localhost:3000/imagecache/images/kitten.jpg?bri=50 |
![]() | ![]() |
http://localhost:3000/imagecache/images/kitten.jpg?bri=-30 |
Increase or decrease the brightness
![]() | ![]() |
http://localhost:3000/imagecache/images/kitten.jpg?con=50 |
![]() | ![]() |
http://localhost:3000/imagecache/images/kitten.jpg?con=-30 |
Flip the image horizontally, vertically, or both
![]() | ![]() |
http://localhost:3000/imagecache/images/kitten.jpg?flip=h |
![]() | ![]() |
http://localhost:3000/imagecache/images/kitten.jpg?flip=v |
![]() | ![]() |
http://localhost:3000/imagecache/images/kitten.jpg?flip=vh |
Colorize image with a preset
![]() | ![]() |
http://localhost:3000/imagecache/images/kitten.jpg?col=greyscale |
![]() | ![]() |
http://localhost:3000/imagecache/images/kitten.jpg?col=sepia |
Blur image with a radius
![]() | ![]() |
http://localhost:3000/imagecache/images/kitten.jpg?blur=15 |
Rotate image and then crop to largest possible rectangle with same aspect ratio
![]() | ![]() |
http://localhost:3000/imagecache/images/kitten.jpg?rot=45 |
Crop the image using a reactangle in the format "x,y,w,h"
![]() | ![]() |
http://localhost:3000/imagecache/images/kitten.jpg?crop=100,100,400,200 |
Resize the image
http://localhost:3000/imagecache/images/kitten.jpg?w=500
http://localhost:3000/imagecache/images/kitten.jpg?w=200&dpi=2
http://localhost:3000/imagecache/images/kitten.jpg?h=250
http://localhost:3000/imagecache/images/kitten.jpg?w=300&h=300
FAQs
Unknown package
The npm package imagecachejs receives a total of 2 weekly downloads. As such, imagecachejs popularity was classified as not popular.
We found that imagecachejs demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.