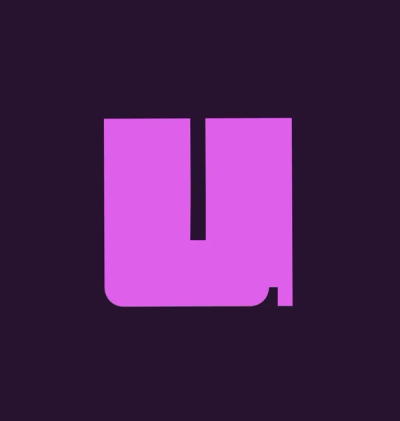
Product
Socket Now Supports uv.lock Files
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
in-process-request
Advanced tools
[](https://travis-ci.org/janaz/in-process-request)
A node.js library that executes a http handler function in the current process without having to start a local http server.
It supports the following frameworks
It has been tested with the following node versions
$ npm install in-process-request
const inProcessRequest = require('in-process-request');
const handler = inProcessRequest(app);
handler(requestOptions)
.then((response) => {
console.log(response);
// do something with the response
});
requestOptions
is an object with the following properties
path
(mandatory) - The request path with optional query string, for example '/my/resource/123?full=true'
method
- request method, the default is 'GET'
body
- request body, string
or Buffer
headers
- request headers object, for example: {'conent-type': 'application/json'}
remoteAddress
- IP address of the client making the requestremotePort
- IP port of the client connectionssl
- Set to true
to pretend that SSL connection is used. Defaults to false
response
is an object with the following properties
statusCode
- status code of the responseheaders
- object with response headersbody
- Buffer
containing the bodyisUTF8
- set to true
if the response is a utf-8 string. In that case response.body.toString()
can be used to extract the utf-8 stringconst inProcessRequest = require('in-process-request')
const express = require('express');
const myApp = express();
myApp.get('/test', (req, res) => {
res.json({ok: true, a: req.query.a});
});
cons myAppHandler = inProcessRequest(myApp);
const requestOptions = {
path: '/test',
method: 'GET',
}
myAppHandler(requestOptions).then(response => {
console.log('Body', response.body);
console.log('Headers', response.headers);
console.log('Status Code', response.statusCode);
console.log('Is UTF8 body?', response.isUTF8);
})
const { expect } = require('chai');
const inProcessRequest = require('in-process-request')
const express = require('express');
const app = express();
app.get('/test', (req, res) => {
res.set('X-Hello', 'world');
res.json({ok: true, a: req.query.a})
});
describe('handler', () => {
it('responds with 200, custom header and query param in JSON body', () => {
const reqOptions = {
path: '/test?a=xyz',
method: 'GET',
}
return inProcessRequest(app)(reqOptions).then(response => {
const json = JSON.parse(response.body.toString())
expect(json).to.deep.equal({ok: true, a: 'xyz'});
expect(response.headers['x-hello']).to.equal('world');
expect(response.statusCode).to.equal(200);
expect(response.isUTF8).to.be.true;
});
});
it('responds with 200, custom header and query param in JSON body', (done) => {
const reqOptions = {
path: '/test?a=xyz',
method: 'GET',
}
inProcessRequest(app)(reqOptions).then(response => {
const json = JSON.parse(response.body.toString())
expect(json).to.deep.equal({ok: true, a: 'xyz'});
expect(response.headers['x-hello']).to.equal('world');
expect(response.statusCode).to.equal(200);
expect(response.isUTF8).to.be.true;
done();
});
});
})
const inProcessRequest = require('in-process-request')
const express = require('express');
const app = express();
app.get('/test', (req, res) => {
res.set('X-Hello', 'world');
res.json({ok: true, a: req.query.a})
});
describe('handler', () => {
it('responds with 200, custom header and query param in JSON body', () => {
const reqOptions = {
path: '/test?a=xyz',
method: 'GET',
}
return inProcessRequest(app)(reqOptions).then(response => {
const json = JSON.parse(response.body.toString())
expect(json).toEqual({ok: true, a: 'xyz'});
expect(response.headers['x-hello']).toEequal('world');
expect(response.statusCode).toEequal(200);
expect(response.isUTF8).to.be.true;
});
});
it('responds with 200, custom header and query param in JSON body', (done) => {
const reqOptions = {
path: '/test?a=xyz',
method: 'GET',
}
inProcessRequest(app)(reqOptions).then(response => {
const json = JSON.parse(response.body.toString())
expect(json).toEqual({ok: true, a: 'xyz'});
expect(response.headers['x-hello']).toEequal('world');
expect(response.statusCode).toEequal(200);
expect(response.isUTF8).toBeTrue();
done();
});
});
})
FAQs
A node.js library that executes a http handler function in the current process without having to start a local http server.
The npm package in-process-request receives a total of 1,183 weekly downloads. As such, in-process-request popularity was classified as popular.
We found that in-process-request demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
Research
Security News
Socket researchers have discovered multiple malicious npm packages targeting Solana private keys, abusing Gmail to exfiltrate the data and drain Solana wallets.
Security News
PEP 770 proposes adding SBOM support to Python packages to improve transparency and catch hidden non-Python dependencies that security tools often miss.