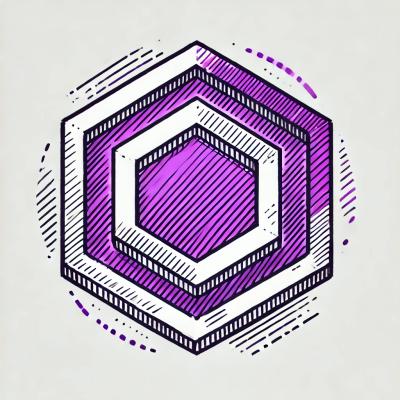
Security News
ESLint is Now Language-Agnostic: Linting JSON, Markdown, and Beyond
ESLint has added JSON and Markdown linting support with new officially-supported plugins, expanding its versatility beyond JavaScript.
Inferno is a fast, lightweight, and highly performant JavaScript library for building modern user interfaces. It is similar to React in terms of API and functionality but is optimized for speed and performance.
Component Creation
Inferno allows you to create components using a class-based approach similar to React. This example demonstrates how to create a simple component that renders a 'Hello, Inferno!' message.
const { Component } = require('inferno');
class MyComponent extends Component {
render() {
return <div>Hello, Inferno!</div>;
}
}
module.exports = MyComponent;
JSX Support
Inferno supports JSX syntax, which allows you to write HTML-like code within your JavaScript. This example shows how to use JSX to create a simple component.
const Inferno = require('inferno');
const MyComponent = () => (
<div>
<h1>Welcome to Inferno</h1>
<p>This is a JSX example.</p>
</div>
);
module.exports = MyComponent;
State Management
Inferno provides state management capabilities similar to React. This example demonstrates a simple counter component that increments the count when a button is clicked.
const { Component } = require('inferno');
class Counter extends Component {
constructor(props) {
super(props);
this.state = { count: 0 };
}
increment = () => {
this.setState({ count: this.state.count + 1 });
};
render() {
return (
<div>
<p>Count: {this.state.count}</p>
<button onClick={this.increment}>Increment</button>
</div>
);
}
}
module.exports = Counter;
Lifecycle Methods
Inferno supports lifecycle methods that allow you to hook into different stages of a component's lifecycle. This example demonstrates the use of `componentDidMount` and `componentWillUnmount` lifecycle methods.
const { Component } = require('inferno');
class LifecycleDemo extends Component {
componentDidMount() {
console.log('Component mounted');
}
componentWillUnmount() {
console.log('Component will unmount');
}
render() {
return <div>Check the console for lifecycle logs.</div>;
}
}
module.exports = LifecycleDemo;
React is a popular JavaScript library for building user interfaces, developed by Facebook. It offers a similar component-based architecture and lifecycle methods as Inferno but is more widely adopted and has a larger ecosystem.
Preact is a fast 3kB alternative to React with the same modern API. It is similar to Inferno in terms of performance and size but has a slightly different approach to handling components and state.
Vue.js is a progressive JavaScript framework for building user interfaces. It offers a more opinionated approach compared to Inferno and includes features like directives and a built-in state management system.
Inferno is a framework for building user-interface components (specifically for the browser's DOM). Inferno's key characteristic is performance, under the hood it uses virtual DOM as a lightweight representation of the actual DOM. However, unlike other frameworks that use virtual DOMs, Inferno does not diff its virtual DOM on each update, but rather it checks the non-static parts of the virtual DOM and checks parts that have only been updated. This technique allows Inferno to achieve blazingly fast DOM operations with very little overhead.
Let's start with some code. As you can see, Inferno intentionally keeps the same good (in our opinion) design ideas regarding components, one-way data passing and separation of concerns.
var message = "Hello world";
Inferno.render(
t7`<MyComponent message=${ message } />`,
document.getElementById("app")
)
Furthermore, Inferno also uses ES6 components like React:
t7.module(funciton(t7) {
class Component implements Inferno.Component {
constructor(props) {
super(props);
this.state.counter = 0;
}
render() {
return t7`
<div>
<h1>Header!</h1>
<span>Counter is at: ${ this.state.counter }</span>
</div>
`;
}
}
t7.assign("Component", Component);
Inferno.render(`<Component />`, document.body);
});
The real difference between React and Inferno is the performance offered at run-time. Inferno can handle large, complex DOM models without breaking a sweat. This is essential for low-power devices such as tablets and phones, where users of those devices are quickly demanding desktop like performance on their slower hardware.
t7
(which comes bundled with Inferno).Inferno tries to address two problems with creating UI components:
Writing code should be fun. Browsers are getting more advanced and the technologies being supported are growing by the week. It's about time a framework offered more fun without compromising performance.
Inferno is still in early development and there are still many missing features and optimisations to be had. Do not use this framework in production environments until a stable release has been stated. Features that still need to be completed:
FAQs
An extremely fast, React-like JavaScript library for building modern user interfaces
The npm package inferno receives a total of 113,685 weekly downloads. As such, inferno popularity was classified as popular.
We found that inferno demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
ESLint has added JSON and Markdown linting support with new officially-supported plugins, expanding its versatility beyond JavaScript.
Security News
Members Hub is conducting large-scale campaigns to artificially boost Discord server metrics, undermining community trust and platform integrity.
Security News
NIST has failed to meet its self-imposed deadline of clearing the NVD's backlog by the end of the fiscal year. Meanwhile, CVE's awaiting analysis have increased by 33% since June.