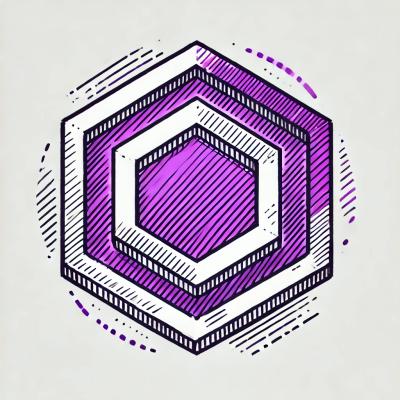
Security News
ESLint is Now Language-Agnostic: Linting JSON, Markdown, and Beyond
ESLint has added JSON and Markdown linting support with new officially-supported plugins, expanding its versatility beyond JavaScript.
Inferno is a fast, lightweight, and highly performant JavaScript library for building modern user interfaces. It is similar to React in terms of API and functionality but is optimized for speed and performance.
Component Creation
Inferno allows you to create components using a class-based approach similar to React. This example demonstrates how to create a simple component that renders a 'Hello, Inferno!' message.
const { Component } = require('inferno');
class MyComponent extends Component {
render() {
return <div>Hello, Inferno!</div>;
}
}
module.exports = MyComponent;
JSX Support
Inferno supports JSX syntax, which allows you to write HTML-like code within your JavaScript. This example shows how to use JSX to create a simple component.
const Inferno = require('inferno');
const MyComponent = () => (
<div>
<h1>Welcome to Inferno</h1>
<p>This is a JSX example.</p>
</div>
);
module.exports = MyComponent;
State Management
Inferno provides state management capabilities similar to React. This example demonstrates a simple counter component that increments the count when a button is clicked.
const { Component } = require('inferno');
class Counter extends Component {
constructor(props) {
super(props);
this.state = { count: 0 };
}
increment = () => {
this.setState({ count: this.state.count + 1 });
};
render() {
return (
<div>
<p>Count: {this.state.count}</p>
<button onClick={this.increment}>Increment</button>
</div>
);
}
}
module.exports = Counter;
Lifecycle Methods
Inferno supports lifecycle methods that allow you to hook into different stages of a component's lifecycle. This example demonstrates the use of `componentDidMount` and `componentWillUnmount` lifecycle methods.
const { Component } = require('inferno');
class LifecycleDemo extends Component {
componentDidMount() {
console.log('Component mounted');
}
componentWillUnmount() {
console.log('Component will unmount');
}
render() {
return <div>Check the console for lifecycle logs.</div>;
}
}
module.exports = LifecycleDemo;
React is a popular JavaScript library for building user interfaces, developed by Facebook. It offers a similar component-based architecture and lifecycle methods as Inferno but is more widely adopted and has a larger ecosystem.
Preact is a fast 3kB alternative to React with the same modern API. It is similar to Inferno in terms of performance and size but has a slightly different approach to handling components and state.
Vue.js is a progressive JavaScript framework for building user interfaces. It offers a more opinionated approach compared to Inferno and includes features like directives and a built-in state management system.
FAQs
An extremely fast, React-like JavaScript library for building modern user interfaces
The npm package inferno receives a total of 113,685 weekly downloads. As such, inferno popularity was classified as popular.
We found that inferno demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
ESLint has added JSON and Markdown linting support with new officially-supported plugins, expanding its versatility beyond JavaScript.
Security News
Members Hub is conducting large-scale campaigns to artificially boost Discord server metrics, undermining community trust and platform integrity.
Security News
NIST has failed to meet its self-imposed deadline of clearing the NVD's backlog by the end of the fiscal year. Meanwhile, CVE's awaiting analysis have increased by 33% since June.