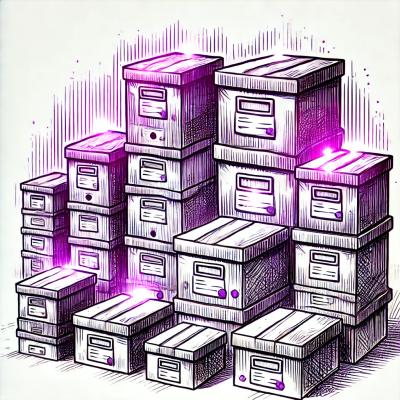
Security News
PyPI’s New Archival Feature Closes a Major Security Gap
PyPI now allows maintainers to archive projects, improving security and helping users make informed decisions about their dependencies.
A lightweight framework and utility for building powerful forms in React applications
Say hello to the best React form library you have ever used! Informed is an extensive, simple, and efficient solution for creating basic to complex forms in React. Out of the box you get the ability to grab and manipulate values, validate fields, create custom inputs, multi-step forms, array fields, and much much more!
Oh and YES WE USE HOOKS!
npm install --save informed
See for yourself.
By default it comes with native dom inputs that are controlled by informed.
import { Form, Input, Select, Checkbox, Relevant, Debug } from 'informed';
const onSubmit = ({ values }) => console.log(values);
const ExampleForm = () => (
<Form onSubmit={onSubmit}>
<Input name="name" label="Name" placeholder="Elon" />
<Input name="age" type="number" label="Age" required="Age Required" />
<Input name="phone" label="Phone" formatter="+1 (###)-###-####" />
<Select name="car" label="Car" initialValue="ms">
<option value="ms">Model S</option>
<option value="m3">Model 3</option>
<option value="mx">Model X</option>
<option value="my">Model Y</option>
</Select>
<Checkbox name="married" label="Married?" />
<Relevant when={({ formState }) => formState.values.married}>
<Input name="spouse" label="Spouse" />
</Relevant>
<button type="submit">Submit</button>
<Debug />
</Form>
);
But what if you dont want the out of the box stuff??
No problem, see example below!
import { useForm, useField, Relevant, FormState } from 'informed';
// Step 1. Build your form component ---------------------
const Form = ({ children, ...rest }) => {
const { formController, render, userProps } = useForm(rest);
return render(
<form noValidate {...userProps} onSubmit={formController.submitForm}>
{children}
</form>
);
};
// Step 2. Build your input components --------------------
const Input = props => {
const { render, informed, userProps, ref } = useField({
type: 'text',
...props
});
const { label, id, ...rest } = userProps;
return render(
<>
<label htmlFor={id}>{label}</label>
<input id={id} ref={ref} {...informed} {...rest} />
</>
);
};
const Checkbox = props => {
const { render, informed, userProps, ref } = useField({
type: 'checkbox',
...props
});
const { label, id, ...rest } = userProps;
return render(
<>
<label htmlFor={id}>{label}</label>
<input id={id} ref={ref} {...informed} {...rest} />
</>
);
};
const ErrorInput = props => {
const { render, informed, userProps, fieldState, ref } = useField({
type: 'text',
...props
});
const { label, id, ...rest } = userProps;
const { showError } = fieldState;
const style = showError ? { border: 'solid 1px red' } : null;
return render(
<>
<label htmlFor={id}>{label}</label>
<input id={id} ref={ref} {...informed} {...rest} style={style} />
{showError && <small style={{ color: 'red' }}>{fieldState.error}</small>}
</>
);
};
const Select = props => {
const { render, informed, userProps, ref } = useField({
type: 'select',
...props
});
const { label, id, children, ...rest } = userProps;
return render(
<>
<label htmlFor={id}>{label}</label>
<select id={id} ref={ref} {...informed} {...rest}>
{children}
</select>
</>
);
};
// Step 3. Build your forms! ---------------------------
const onSubmit = ({ values }) => console.log(values);
const ExampleForm = () => (
<Form onSubmit={onSubmit}>
<Input name="name" label="Name" placeholder="Elon" />
<ErrorInput name="age" type="number" label="Age" required="Age Required" />
<Input name="phone" label="Phone" formatter="+1 (###)-###-####" />
<Select name="car" label="Car" initialValue="ms">
<option value="ms">Model S</option>
<option value="m3">Model 3</option>
<option value="mx">Model X</option>
<option value="my">Model Y</option>
</Select>
<Checkbox name="married" label="Married?" />
<Relevant when={({ formState }) => formState.values.married}>
<Input name="spouse" label="Spouse" />
</Relevant>
<button type="submit">Submit</button>
<Debug />
</Form>
);
4.51.2 ( Sep 13th, 2023)
FAQs
A lightweight framework and utility for building powerful forms in React applications
The npm package informed receives a total of 11,118 weekly downloads. As such, informed popularity was classified as popular.
We found that informed demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PyPI now allows maintainers to archive projects, improving security and helping users make informed decisions about their dependencies.
Research
Security News
Malicious npm package postcss-optimizer delivers BeaverTail malware, targeting developer systems; similarities to past campaigns suggest a North Korean connection.
Security News
CISA's KEV data is now on GitHub, offering easier access, API integration, commit history tracking, and automated updates for security teams and researchers.