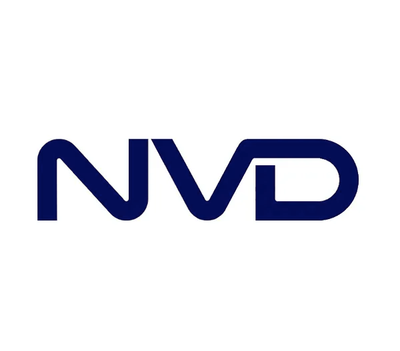
Security News
NVD Backlog Tops 20,000 CVEs Awaiting Analysis as NIST Prepares System Updates
NVD’s backlog surpasses 20,000 CVEs as analysis slows and NIST announces new system updates to address ongoing delays.
input-format
Advanced tools
Formatting user's text input on-the-fly
npm install input-format --save
Define parse()
and format()
functions. For example, for parsing a phone number.
import { templateParser, templateFormatter, parseDigit } from 'input-format'
// US phone number template
const TEMPLATE = '(xxx) xxx-xxxx'
// `parse` function parses input text characters one-by-one.
//
// `function parse(character, value) { return character }`
//
// Arguments:
// * `character` is the currently parsed input text character.
// * `value` is the parsed value so far.
//
// Returns:
// * If it returns anything (not `undefined`) then it is appended to the `value`
//
// `parseDigit` is an exported helper `parse` function
// that returns `character` if it's a digit
// (a common case, e.g. phone numbers input).
//
// `templateParser` wrapper is a small helper
// which enhances `parse` function to limit `value` max length
// to the number of "x"-es in the template.
//
const parse = templateParser(TEMPLATE, parseDigit)
// `format` function formats parsed value.
//
// function format(value) { return { text: '(800) 555-3535', template: '(xxx) xxx-xxxx' } }
//
// Arguments:
// * `value` is the parsed value to be formatted.
//
// Returns `{ text, template }`, where:
// * `text` is the formatted text
// * `template` is the template used to format the `text`
// (can be a partial template or a full template)
//
// If the `value` couldn't be formatted then
// `format()` should just return `undefined`.
//
// `templateFormatter` helper creates a formatter based on a template.
//
const format = templateFormatter(TEMPLATE)
Then pass these parse()
and format()
functions to the library.
React Component:
import { ReactInput } from 'input-format'
<ReactInput
value={this.state.phone}
onChange={phone => this.setState({ phone })}
parse={parse}
format={format}/>
Low-level Input Component API:
import
{
onChange,
onCut,
onPaste,
onKeyDown
}
from 'input-format'
const input = document.querySelector('input')
onChange(event, input, parse, format, onChangeHandler)
onCut(event, input, parse, format, onChangeHandler)
onPaste(event, input, parse, format, onChangeHandler)
onKeyDown(event, input, parse, format, onChangeHandler)
Core API:
import { parse, format } from 'input-format'
// Input character parser for `parse()`.
function _parse(character, value)
{
if (value.length < 10)
{
if (character >= '0' && character <= '9')
{
return character
}
}
}
// Output text formatter for `format()`.
function _format(value)
{
...
// Just as an example of a return value
return {
text: '(800) 555-3535',
template: '(xxx) xxx-xxxx'
}
}
// Testing.
let value
let text = '(800) 555-3535'
let caret = 4 // before the first zero
// `parse()`.
{ value, caret } = parse(text, caret, _parse)
value === '8005553535'
caret === 2
// `format()`.
{ text, caret } = format(value, caret, _format)
value === '(800) 555-3535'
caret === 4
There have been some reports of non-stock Android keyboards not handling caret positioning properly. I don't have such an Android phone at my disposal to debug that issue.
After cloning this repo, ensure dependencies are installed by running:
npm install
This module is written in ES6 and uses Babel for ES5 transpilation. Widely consumable JavaScript can be produced by running:
npm run build
Once npm run build
has run, you may import
or require()
directly from
node.
After developing, the full test suite can be evaluated by running:
npm test
When you're ready to test your new functionality on a real project, you can run
npm pack
It will build
, test
and then create a .tgz
archive which you can then install in your project folder
npm install [module name with version].tar.gz
FAQs
Formatting user's text input on-the-fly
The npm package input-format receives a total of 519,571 weekly downloads. As such, input-format popularity was classified as popular.
We found that input-format demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
NVD’s backlog surpasses 20,000 CVEs as analysis slows and NIST announces new system updates to address ongoing delays.
Security News
Research
A malicious npm package disguised as a WhatsApp client is exploiting authentication flows with a remote kill switch to exfiltrate data and destroy files.
Security News
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.