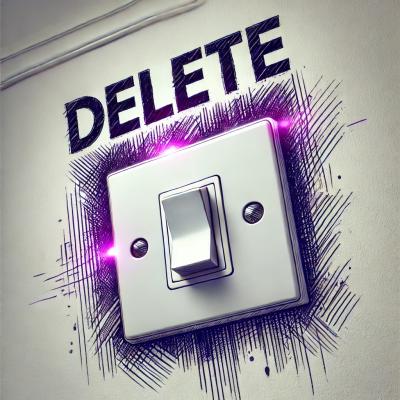
Security News
Research
Destructive npm Packages Disguised as Utilities Enable Remote System Wipe
Socket uncovered two npm packages that register hidden HTTP endpoints to delete all files on command.
instagram-graph-api
Advanced tools
A library to help perform requests to the Instagram Graph API.
This npm package lets you easily perform requests to the Instagram Graph API, aiming to reduce the integration time and allowing you to easily obtain the information you want through code and to publish your media without hassle.
This package is made by independent contributors and is in no way officially related to or supported by Instagram/Facebook.
You can find what changed in each version by checking the Changelog.
This lib is now dependency free 🎉
Axios has been removed as a dependency. execute()
and config()
methods still work exactly the same on the outside.
Error handling using AxiosError
no longer being possible is essentially the major change.
Simply run npm install instagram-graph-api
.
This lib supports making requests to most of the Instagram Graph API resources. It does not yet cover:
Mentions
, Mentioned Comments
and Mentioned Media
in the IG User
node.As it currently stands, this lib allows you to get a lot of information about your page and media, including basic information and insights. It also allows you to create and publish media (photos and videos) as well as to create and reply to comments, delete them and hide them. For now, this lib does not contain any complex logic. It simply models requests to the Instagram Graph API and gives you an easy way to execute them.
You can always check the typedoc documentation if you are having doubts.
This lib was built with a simple mindset: creating requests should be as straightforward as possible. With that in mind, to start pumping out requests left and right, just create a client and use it to build requests for you:
import { Client, GetPageInfoRequest, GetPageMediaRequest } from 'instagram-graph-api';
const client: Client = new Client(ACCESS_TOKEN, PAGE_ID);
const pageInfoRequest: GetPageInfoRequest = client.newGetPageInfoRequest();
const pageMediaRequest: GetPageMediaRequest = client.newGetPageMediaRequest();
[...]
You can also build each request yourself, and that won't be hard at all. You'll just have to pass the Access Token and the Page ID to each new request. Here's an example:
const request: GetPageInfoRequest = new GetPageInfoRequest(ACCESS_TOKEN, PAGE_ID);
After you build your request object, you can do one of two things:
You can execute the request with the built in method that returns a parsed response. This will use the native Node fetch
.
import { GetPageInfoRequest, GetPageInfoResponse } from 'instagram-graph-api';
const request: GetPageInfoRequest = new GetPageInfoRequest(ACCESS_TOKEN, PAGE_ID);
request.execute().then((response: GetPageInfoResponse) => {
console.log(`The page ${response.getName()} has ${response.getFollowers()} followers.`);
});
Alternatively, you can extract the request config to modify it, execute it and parse the response as you see fit.
import { GetPageInfoRequest, RequestConfig } from 'instagram-graph-api';
const request: GetPageInfoRequest = new GetPageInfoRequest(ACCESS_TOKEN, PAGE_ID);
const config: RequestConfig = request.config();
[...]
Publishing Media through the Instagram Graph API, and conversely through this lib, follows these steps:
PostPagePhotoMediaRequest
.PostPageVideoMediaRequest
.PostPageReelMediaRequest
.PostPageStoryPhotoMediaRequest
PostPageStoryVideoMediaRequest
FINISHED
(check the status through the GetContainerRequest
).PostPublishMediaRequest
).For more info on this flow, refer to the Content Publishing documentation.
Publishing carousels is similar to posting other media types, but you need to create the child containers first. The steps are:
FINISHED
. Do not publish them!.PostPageCarouselMediaRequest
.FINISHED
.You can give paging and range options to the requests, as supported by certain resources on the Instagram Graph API. (check the reference documentation to see which ones do). For example, the Get Page Media request supports paging, here's a naive example of how to use:
import { GetPageMediaRequest, GetPageMediaResponse, PageOption } from 'instagram-graph-api';
const request: GetPageMediaRequest = new GetPageMediaRequest(ACCESS_TOKEN, PAGE_ID);
request.execute().then((response: GetPageMediaResponse) => {
const nextPage: string | undefined = response.getPaging().getAfter();
if (nextPage) {
request.withPaging({ option: PageOption.AFTER, value: nextPage })
.execute([...]); // you can reuse the old request 😎
} else {
console.log('🛑🛑🛑');
}
});
Similarly, you can add a range option which the Get Insights requests use, like so:
import { GetPageLifetimeInsightsRequest, GetPageLifetimeInsightsRequest } from 'instagram-graph-api';
const request: GetPageLifetimeInsightsRequest = new GetPageLifetimeInsightsRequest(ACCESS_TOKEN, PAGE_ID)
.withRange(new Date('2021-01-01'), new Date('2021-01-15'))
request.execute().then((response: GetPageLifetimeInsightsResponse) => {
[...]
});
Finally, you can add a limit option that will limit the amount of objects retrieved with that request. For example:
import { GetPageMediaRequest, GetPageMediaResponse } from 'instagram-graph-api';
const request: GetPageMediaRequest = new GetPageMediaRequest(ACCESS_TOKEN, PAGE_ID).withLimit(5);
request.execute().then((response: GetPageMediaResponse) => {
console.log(response.getData().length); // This will be < 5
});
To use this lib you'll require an Access Token to Facebook's Graph API (over which the Instagram Graph API is built), which in turn will require a couple of other things. Check out the before you start on the getting started documentation.
You can follow Facebook's documentation on how to generate an access token to grab a token for yourself. You'll most likely want a Page Token. Check which permissions you'll need for the request you want to perform on the Instagram Graph API Reference.
You can also extend your token's validity to avoid having to generate a new one once in a while. Once again, Facebook's documentation on how to generate long-lived tokens will come in handy. Long-lived Page Tokens do not have an expiration date, making them ideal for most use cases of this lib.
For some use cases, for example when you want to build a tool for your own pages, it's fine to go through the above steps to generate a long-lived token. For other uses cases, such as when you have an app that will make request on behalf of users, it becomes a bother.
This lib provides a few requests to get you around that:
First, you still need to get your hands on an initial access token. You can get it via a facebook login prompt for users of your app.
When you have a user token, you can build and execute a GetUserLongLivedTokenRequest
:
import { GetUserLongLivedTokenRequest, GetUserLongLivedTokenResponse } from 'instagram-graph-api';
// You can get your APP_ID and APP_SECRET_ID through the Facebook Developers website.
const request: GetUserLongLivedTokenRequest = new GetUserLongLivedTokenRequest(
APP_ID,
APP_SECRET_ID,
USER_ACCESS_TOKEN
);
request.execute().then((response: GetUserLongLivedTokenResponse) => {
const longLivedToken: string = response.getLongLivedToken();
});
All the requests to a page will require the use of a Page ID. To find out what the ID of a page is, you can:
Instagram Page ID
on a search engine)GetLinkedInstagramAccountRequest
, as such:import { GetLinkedInstagramAccountRequest, GetLinkedInstagramAccountResponse } from 'instagram-graph-api';
const request: GetLinkedInstagramAccountRequest = new GetLinkedInstagramAccountRequest(ACCESS_TOKEN, FACEBOOK_PAGE_ID);
request.execute().then((response: GetLinkedInstagramAccountResponse) => {
const pageId: string = response.getInstagramPageId();
});
FACEBOOK_PAGE_ID
through the Facebook Developers portal but you can also get it through this lib:import { GetAuthorizedFacebookPagesRequest, GetAuthorizedFacebookPagesResponse } from 'instagram-graph-api';
const request: GetAuthorizedFacebookPagesRequest = new GetAuthorizedFacebookPagesRequest(ACCESS_TOKEN);
request.execute().then((response: GetAuthorizedFacebookPagesResponse) => {
const firstFacebookPage: string = pagesResponse.getAuthorizedFacebookPages()[0].id;
});
This project follows Semantic Release with a publish on every commit to master
.
FAQs
A library to help perform requests to the Instagram Graph API.
The npm package instagram-graph-api receives a total of 1,270 weekly downloads. As such, instagram-graph-api popularity was classified as popular.
We found that instagram-graph-api demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Research
Socket uncovered two npm packages that register hidden HTTP endpoints to delete all files on command.
Research
Security News
Malicious Ruby gems typosquat Fastlane plugins to steal Telegram bot tokens, messages, and files, exploiting demand after Vietnam’s Telegram ban.
Research
Security News
Socket uncovered four malicious npm packages that exfiltrate up to 85% of a victim’s Ethereum or BSC wallet using obfuscated JavaScript.