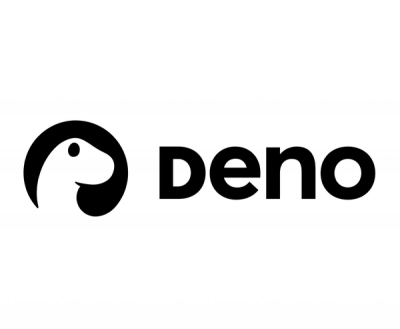
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Webcam-driven HTML5 QR code scanner.
<!DOCTYPE html>
<html>
<head>
<title>Instascan</title>
<script type="text/javascript" src="dist/instascan.min.js"></script>
</head>
<body>
<video id="monitor"></video>
<script type="text/javascript">
var opts = {
monitor: document.getElementById('monitor'),
mirror: true,
backgroundScan: false,
refractoryPeriod: 3 * 1000,
scanPeriod: 5,
captureImage: false
};
var scanner = new Instascan.Scanner(opts);
scanner.addListener('scan', function (content, image) {
console.log(content);
});
Instascan.Camera.getCameras(function (err, cameras) {
if (cameras && cameras.length > 0) {
scanner.start(cameras[0]);
}
});
</script>
</body>
</html>
new Instascan.Scanner(opts)
opts.monitor
: The HTML element to use for the camera's video preview. Must be a <video>
element. By default, an invisible element will be created.opts.mirror
: Whether to mirror the video preview. Default true
.opts.backgroundScan
: Whether to scan when the tab is not shown/focused. When false
, this reduces CPU usage when the page is not active. Default false
.opts.refractoryPeriod
: The period, in milliseconds, before the same QR code will be consecutively recognized. Default 5000
.opts.scanPeriod
: The period, in rendered frames, between scans. A lower scan period increases CPU usage but makes scan response faster. Default 1
(i.e. analyze every frame).opts.captureImage
: Whether to include the scanned image data as part of the scan result. See the scan
event for format details. Default false
.scanner.start(camera)
camera
as the source.camera
: Instance of Instascan.Camera
from Instascan.Camera.getCameras
.scanner.stop()
scanner.addListener('scan', callback)
callback
: function (content, image)
content
: Scanned content decoded from the QR code.image
: null
if scanner.captureImage
is false
, otherwise, a base64-encoded WebP-compressed data URI of the camera frame used to decode the QR code.scanner.addListener('active', callback)
scanner.start
or the tab gaining focus.callback
: function ()
scanner.addListener('inactive', callback)
scanner.stop
or the tab losing focus.callback
: function ()
scanner.addListener('error', callback)
callback
: function (err)
err
: Error details.Instascan.Camera.getCameras(callback)
callback
: function (err, cameras)
err
: Any errors that occurred when enumerating cameras.cameras
: Array of Instascan.Camera
instances available for use.camera.id
camera.name
Microsoft Lifecam HD-3000
.Copyright © 2016 Chris Schmich
MIT License. See LICENSE for details.
FAQs
Webcam-driven QR code scanner.
The npm package instascan receives a total of 7,764 weekly downloads. As such, instascan popularity was classified as popular.
We found that instascan demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.