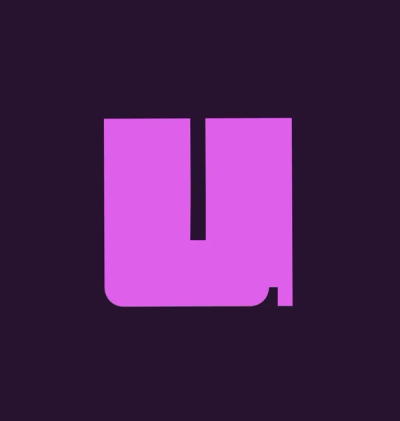
Product
Socket Now Supports uv.lock Files
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
intercom-client
Advanced tools
The intercom-client npm package is a Node.js client for the Intercom API. It allows developers to interact with Intercom's services programmatically, enabling functionalities such as managing users, sending messages, and handling conversations.
Managing Users
This feature allows you to create and manage users in your Intercom account. The code sample demonstrates how to create a new user with an email and name.
const Intercom = require('intercom-client');
const client = new Intercom.Client({ token: 'your_access_token' });
client.users.create({
email: 'test@example.com',
name: 'Test User'
}).then(response => {
console.log(response.body);
}).catch(error => {
console.error(error);
});
Sending Messages
This feature allows you to send messages to users. The code sample demonstrates how to send an in-app message from an admin to a user.
const Intercom = require('intercom-client');
const client = new Intercom.Client({ token: 'your_access_token' });
client.messages.create({
message_type: 'inapp',
body: 'Hello, this is a test message!',
from: {
type: 'admin',
id: 'admin_id'
},
to: {
type: 'user',
id: 'user_id'
}
}).then(response => {
console.log(response.body);
}).catch(error => {
console.error(error);
});
Handling Conversations
This feature allows you to list and manage conversations. The code sample demonstrates how to list all conversations for a specific admin.
const Intercom = require('intercom-client');
const client = new Intercom.Client({ token: 'your_access_token' });
client.conversations.list({
type: 'admin',
admin_id: 'admin_id'
}).then(response => {
console.log(response.body);
}).catch(error => {
console.error(error);
});
The hubspot-api package provides a client for interacting with HubSpot's API. Similar to intercom-client, it allows for managing contacts, sending messages, and handling conversations. However, it is specific to HubSpot's CRM and marketing tools.
The zendesk-node-api package is a client for the Zendesk API. It offers functionalities for managing tickets, users, and other support-related tasks. While it shares some similarities with intercom-client in terms of user and conversation management, it is tailored for Zendesk's customer support platform.
The freshdesk-api package provides a client for interacting with Freshdesk's API. It allows for managing tickets, contacts, and conversations, similar to intercom-client. However, it is designed specifically for Freshdesk's customer support and helpdesk services.
Official Node bindings to the Intercom API
This client library is in active development. Full API documentation can be found here.
npm install intercom-client
npm test
Compile using babel:
gulp babel
Require Intercom:
var Intercom = require('./dist/index');
Require Intercom:
var Intercom = require('intercom-node');
Create a client:
var client = new Intercom.Client('app_id', 'app_api_key');
// Create/update a user
client.users.create({ email: 'jayne@serenity.io' }, function (r) {
console.log(r);
});
// List users
client.users.list(callback);
// List users by tag or segment
client.users.listBy({ tag_id: 'haven' }, callback);
// Find user by id
client.users.find({ id: '1234' }, callback);
// Delete user by id
client.users.delete({ id: '1234' }, callback);
// Create a contact
client.contacts.create(function (r) {
console.log(r);
});
// Update a contact by id
client.contacts.update({ id: '5435345', email: 'wash@serenity.io' }, callback);
// List contacts
client.contacts.list(callback);
// List contacts by email
client.contacts.listBy({ email: 'wash@serenity.io' }, callback);
// Find contact by id
client.contacts.find({ id: '5342423' }, callback);
// Delete contact by id
client.contacts.delete({ id: '5342423' }, callback);
// Convert Contacts into Users
var conversion = {
contact: { user_id: '1234-5678-9876' },
user: { email: 'mal@serenity.io' }
};
client.contacts.convert(conversion, callback);
// Create/update a company
client.companies.create({ company_id: '1234', name: 'serenity' }, function (r) {
console.log(r);
});
// List companies
client.companies.list(callback);
// List companies by tag or segment
client.companies.listBy({ tag_id: 'haven' }, callback);
// Find company by id
client.companies.find({ id: '1234' }, callback);
// List company users
client.companies.listUsers({ id: '1234' }, callback);
// Create a event
client.events.create({
event_name: 'Foo',
created_at: 1439826340,
user_id: 'bar'
}, function (d) {
console.log(d);
});
client.counts.appCounts(callback);
client.counts.conversationCounts(callback);
client.counts.conversationAdminCounts(callback);
client.counts.userTagCounts(callback);
client.counts.userSegmentCounts(callback);
client.counts.companyTagCounts(callback);
client.counts.companySegmentCounts(callback);
client.counts.companyUserCounts(callback);
// List admins
client.admins.list(callback);
// Create a tag
client.tags.create({ name: 'haven' }, callback);
// Tag a user by id
client.tags.tag({ name: 'haven', users: [{ id: '54645654' }] }, callback);
// Tag a company by id
client.tags.tag({ name: 'haven', companies: [{ id: '54645654' }] }, callback);
// Untag a user by id
client.tags.untag({ name: 'haven', users: [{ id: '5345342' }] }, callback);
// List tags
client.tags.list(callback);
// Delete a tag by id
client.tags.delete({ id: '130963' }, callback);
// List segments
client.segments.list(callback);
// Find segment by id
client.segments.find({ id: '55719a4a' }, callback);
The Bulk APIs are themselves in Beta, but allow for the asynchronous creation and deletion of users:
client.users.bulk([
{ create: { email: 'wash@serenity.io' }},
{ create: { email: 'mal@serenity.io'}}
], callback);
When listing, the Intercom API may return a pagination object:
{
"pages": {
"next": "..."
}
}
You can grab the next page of results using the client:
client.nextPage(response.pages, callback);
Apache-2.0
FAQs
[](https://buildwithfern.com?utm_source=github&utm_medium=github&utm_campaign=readme&utm_source=https%3A%2F%2Fgithub.com%2Fintercom%2Fintercom-node) [![npm shield](ht
The npm package intercom-client receives a total of 68,689 weekly downloads. As such, intercom-client popularity was classified as popular.
We found that intercom-client demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
Research
Security News
Socket researchers have discovered multiple malicious npm packages targeting Solana private keys, abusing Gmail to exfiltrate the data and drain Solana wallets.
Security News
PEP 770 proposes adding SBOM support to Python packages to improve transparency and catch hidden non-Python dependencies that security tools often miss.