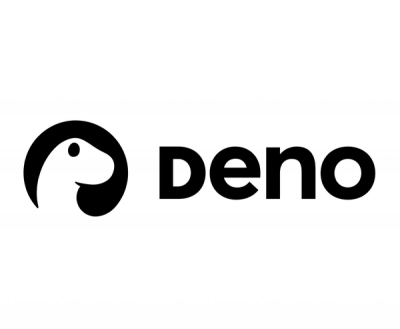
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Installation | Getting Started | Contributing | License
Send and receive faxes in Node / Javascript with the InterFAX REST API.
This library requires Node 4+ and can be installed via NPM.
npm install interfax --save
The module is written in ES6 and is compiled to ES5 for backwards compatibility. All documentation below is ES6.
All our API calls support Promises to handle asynchrounous callbacks. For example to send a fax from a PDF file:
import InterFAX from 'interfax';
let interfax = new InterFAX();
interfax.deliver({
faxNumber : '+11111111112',
file : 'folder/fax.pdf'
}).then(fax => {
console.log(fax.id); //=> the Fax ID just created
})
.catch(error => {
console.log(error); //=> an error object
});
Alternatively we also support callbacks instead of promises.
interfax.deliver({
faxNumber : '+11111111112',
file : 'folder/fax.pdf'
}, function(error, response) {
if (error) {
console.log(error); //=> an error object
} else {
console.log(response.id); //=> the Fax ID just created
}
});
Client | Account | Outbound | Inbound | Documents | Helper Classes
The client follows the 12-factor apps principle and can be either set directly or via environment variables.
import InterFAX from 'interfax';
// Initialize using parameters
let interfax = new InterFAX({
username: '...',
password: '...'
})
// Alternative: Initialize using
// environment variables
// * INTERFAX_USERNAME
// * INTERFAX_PASSWORD
let interfax = new InterFAX()
All connections are established over HTTPS.
interfax.account.balance(callback);
Determine the remaining faxing credits in your account.
interfax.account.balance()
.then(balance => {
console.log(balance); //=> 9.86
});
More: documentation
Send | Get list | Get completed list | Get record | Get image | Cancel fax | Search
TBD
interfax.outbound.all(options, callback);
Get a list of recent outbound faxes (which does not include batch faxes).
interfax.outbound.all({
limit: 5
}).then(faxes => {
console.log(faxes); //=> an array of fax objects
});
Options: limit
, lastId
, sortOrder
, userId
interfax.outbound.completed(array_of_ids, callback);
Get details for a subset of completed faxes from a submitted list. (Submitted id's which have not completed are ignored).
interfax.outbound.completed([123, 234])
.then(faxes => {
console.log(faxes); //=> an array of fax objects
});
More: documentation
interfax.outbound.find(fax_id, callback);
Retrieves information regarding a previously-submitted fax, including its current status.
interfax.outbound.find(123456)
.then(fax => {
console.log(fax); //=> fax object
});
More: documentation
interfax.outbound.image(fax_id, callback);
Retrieve the fax image (TIFF file) of a submitted fax.
interfax.outbound.image(123456)
.then(image => {
console.log(image.data); //=> TIFF image data
image.save('file.tiff'); //=> saves image to file
});
More: documentation
interfax.outbound.cancel(fax_id, callback);
Cancel a fax in progress.
interfax.outbound.cancel(123456)
.then(fax => {
console.log(fax); //=> fax object
});
More: documentation
interfax.outbound.search(options, callback);
Search for outbound faxes.
interfax.outbound.search({
faxNumber: '+1230002305555'
}).then(faxes => {
console.log(faxes); //=> an array of fax objects
});
Options: ids
, reference
, dateFrom
, dateTo
, status
, userId
, faxNumber
, limit
, offset
Get list | Get record | Get image | Get emails | Mark as read | Resend to email
interfax.inbound.all(options, callback);
Retrieves a user's list of inbound faxes. (Sort order is always in descending ID).
interfax.inbound.all({
limit: 5
}).then(faxes => {
console.log(faxes); //=> an array of fax objects
});
Options: unreadOnly
, limit
, lastId
, allUsers
interfax.inbound.find(fax_id, callback);
Retrieves a single fax's metadata (receive time, sender number, etc.).
interfax.inbound.find(123456)
.then(fax => {
console.log(fax); //=> fax object
});
More: documentation
interfax.inbound.image(fax_id, callback);
Retrieves a single fax's image.
interfax.inbound.image(123456)
.then(image => {
console.log(image.data); //=> TIFF image data
image.save('file.tiff'); //=> saves image to file
});
More: documentation
interfax.inbound.emails(fax_id, callback);
Retrieve the list of email addresses to which a fax was forwarded.
interfax.inbound.emails(123456)
.then(emails => {
console.log(emails); //=> a list of email objects
});
More: documentation
interfax.inbound.mark(fax_id, is_read, callback);
Mark a transaction as read/unread.
// mark as read
interfax.inbound.mark(123456, true)
.then((success) => {
console.log(success); // boolean
});
// mark as unread
interfax.inbound.mark(123456, false)
.then((success) => {
console.log(success); // boolean
});
More: documentation
interfax.inbound.resend(fax_id, to_email, callback);
Resend an inbound fax to a specific email address.
// resend to the email(s) to which the fax was previously forwarded
interfax.inbound.resend(123456)
.then((success) => {
console.log(success); // boolean
});
// resend to a specific address
interfax.inbound.resend(123456, 'test@example.com')
.then((success) => {
console.log(success); // boolean
});
=> true
More: documentation
Create | Upload chunk | Get list | Status | Cancel
Document allow for uploading of large files up to 20MB in 200kb chunks. For example to upload a 500
import fs from 'fs';
let upload = function(cursor = 0, document, data) {
if (cursor >= data.length) { return };
let chunk = data.slice(cursor, cursor+500).toString('ASCII');
let next_cursor = cursor+chunk.length;
interfax.documents.upload(document.id, cursor, next_cursor-1, chunk)
.then(() => { upload(next_cursor, document, data); });
}
fs.readFile('tests/test.pdf', function(err, data){
interfax.documents.create('test.pdf', data.length)
.then(document => { upload(0, document, data); });
});
interfax.documents.create(name, size, options, callback);
Create a document upload session, allowing you to upload large files in chunks.
interfax.documents.create('large_file.pdf', 231234)
.then(document => {
console.log(document.id); // the ID of the document created
});
Options: disposition
, sharing
interfax.documents.upload(id, range_start, range_end, chunk, callback);
Upload a chunk to an existing document upload session.
interfax.documents.upload(123456, 0, 999, "....binary-data....")
.then(document => {
console.log(document);
});
More: documentation
interfax.documents.all(options, callback);
Get a list of previous document uploads which are currently available.
interfax.documents.all({
offset: 10
}).then(documents => {
console.log(documents); //=> a list of documents
});
Options: limit
, offset
interfax.documents.find(document_id, callback);
Get the current status of a specific document upload.
interfax.documents.find(123456)
.then(document => {
console.log(document); //=> a document object
});
More: documentation
interfax.documents.cancel(document_id, callback);
Cancel a document upload and tear down the upload session, or delete a previous upload.
interfax.documents.cancel(123456)
.then(success => {
console.log(success); //=> boolean
})
More: documentation
This library is released under the MIT License.
[0.4.0]
Add chunk uploading.
FAQs
A wrapper around the InterFAX REST API for sending and receiving faxes.
The npm package interfax receives a total of 2,134 weekly downloads. As such, interfax popularity was classified as popular.
We found that interfax demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.