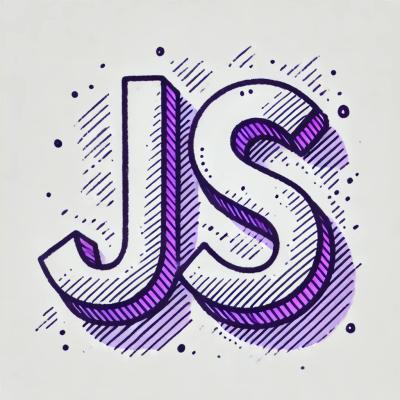
Security News
JavaScript Leaders Demand Oracle Release the JavaScript Trademark
In an open letter, JavaScript community leaders urge Oracle to give up the JavaScript trademark, arguing that it has been effectively abandoned through nonuse.
The io-ts npm package is a TypeScript library that allows for the definition of runtime types, and the automatic validation of runtime values against those types. It leverages TypeScript's type system to ensure that data structures conform to specified schemas, providing a bridge between the runtime data and compile-time types.
Runtime type validation
This feature allows you to define a type and then validate an object against that type at runtime. If the object matches the type, the 'Right' branch is executed; otherwise, the 'Left' branch indicates a validation error.
{"const t = require('io-ts');\nconst User = t.type({\n name: t.string,\n age: t.number\n});\nconst result = User.decode({ name: 'Alice', age: 25 });\nif (result._tag === 'Right') {\n console.log('Valid!', result.right);\n} else {\n console.log('Invalid!', result.left);\n}"}
Type composition
io-ts allows for the composition of types, enabling complex type definitions by combining simpler ones. This is useful for building up the shape of data structures from reusable type components.
{"const t = require('io-ts');\nconst Name = t.string;\nconst Age = t.number;\nconst User = t.type({ name: Name, age: Age });\nconst result = User.decode({ name: 'Bob', age: 'not-a-number' });\n// result will be an instance of Left since 'age' is not a number"}
Custom types
io-ts allows the creation of custom types with additional validation logic. In this example, a 'PositiveNumber' type is created that only accepts positive numbers.
{"const t = require('io-ts');\nconst PositiveNumber = t.brand(\n t.number,\n (n): n is t.Branded<number, { readonly PositiveNumber: unique symbol }> => n > 0,\n 'PositiveNumber'\n);\nconst result = PositiveNumber.decode(-5);\n// result will be an instance of Left since the number is not positive"}
Ajv is a JSON schema validator that provides runtime data validation using predefined JSON schemas. It is similar to io-ts in that it validates data structures at runtime, but it uses JSON schema as the basis for validation rather than TypeScript types.
Joi is an object schema validation library that allows for the description and validation of JavaScript objects. It is similar to io-ts in providing runtime validation, but it uses a fluent API for schema definition and does not integrate with TypeScript types in the same way.
Yup is a JavaScript schema builder for value parsing and validation. It defines a schema using a declarative API and validates objects against the schema. Like io-ts, it provides runtime validation, but it does not leverage TypeScript's type system for type definitions.
Class-validator allows for validation of class instances based on decorators. It is similar to io-ts in that it provides runtime validation, but it is designed to work with classes and decorators, offering a different approach to defining validation rules.
Blog post: "Typescript and validations at runtime boundaries" by @lorefnon
A value of type Type<A, O, I>
(called "runtime type") is the runtime representation of the static type A
.
Also a runtime type can
I
(through decode
)O
(through encode
)is
)export type mixed = object | number | string | boolean | symbol | undefined | null
class Type<A, O = A, I = mixed> {
readonly _A: A
readonly _O: O
readonly _I: I
constructor(
/** a unique name for this runtime type */
readonly name: string,
/** a custom type guard */
readonly is: (v: mixed) => v is A,
/** succeeds if a value of type I can be decoded to a value of type A */
readonly validate: (input: I, context: Context) => Either<Errors, A>,
/** converts a value of type A to a value of type O */
readonly encode: (a: A) => O
) {}
/** a version of `validate` with a default context */
decode(i: I): Either<Errors, A>
}
Note. The Either
type is defined in fp-ts, a library containing implementations of
common algebraic types in TypeScript.
Example
A runtime type representing string
can be defined as
import * as t from 'io-ts'
// runtime type definition
export class StringType extends t.Type<string> {
// equivalent to Type<string, string, mixed> as per type parameter defaults
readonly _tag: 'StringType' = 'StringType'
constructor() {
super(
'string',
(m): m is string => typeof m === 'string',
(m, c) => (this.is(m) ? t.success(m) : t.failure(m, c)),
t.identity
)
}
}
// runtime type instance: use this when building other runtime types instances
export const string = new StringType()
A runtime type can be used to validate an object in memory (for example an API payload)
const Person = t.type({
name: t.string,
age: t.number
})
// validation succeeded
Person.decode(JSON.parse('{"name":"Giulio","age":43}')) // => Right({name: "Giulio", age: 43})
// validation failed
Person.decode(JSON.parse('{"name":"Giulio"}')) // => Left([...])
The stable version is tested against TypeScript 2.9.x+
A reporter implements the following interface
interface Reporter<A> {
report: (validation: Validation<any>) => A
}
This package exports two default reporters
PathReporter: Reporter<Array<string>>
ThrowReporter: Reporter<void>
Example
import { PathReporter } from 'io-ts/lib/PathReporter'
import { ThrowReporter } from 'io-ts/lib/ThrowReporter'
const result = Person.decode({ name: 'Giulio' })
console.log(PathReporter.report(result))
// => ['Invalid value undefined supplied to : { name: string, age: number }/age: number']
ThrowReporter.report(result)
// => throws 'Invalid value undefined supplied to : { name: string, age: number }/age: number'
Runtime types can be inspected
This library uses TypeScript extensively. Its API is defined in a way which automatically infers types for produced values
Note that the type annotation isn't needed, TypeScript infers the type automatically based on a schema.
Static types can be extracted from runtime types using the TypeOf
operator
type IPerson = t.TypeOf<typeof Person>
// same as
type IPerson = {
name: string
age: number
}
import * as t from 'io-ts'
Type | TypeScript | Runtime type / combinator |
---|---|---|
null | null | t.null or t.nullType |
undefined | undefined | t.undefined |
void | void | t.void or t.voidType |
string | string | t.string |
number | number | t.number |
boolean | boolean | t.boolean |
any | any | t.any |
never | never | t.never |
object | object | t.object |
integer | ✘ | t.Integer |
array of any | Array<mixed> | t.Array |
array of type | Array<A> | t.array(A) |
dictionary of any | { [key: string]: mixed } | t.Dictionary |
dictionary of type | { [K in A]: B } | t.dictionary(A, B) |
function | Function | t.Function |
literal | 's' | t.literal('s') |
partial | Partial<{ name: string }> | t.partial({ name: t.string }) |
readonly | Readonly<T> | t.readonly(T) |
readonly array | ReadonlyArray<number> | t.readonlyArray(t.number) |
type alias | type A = { name: string } | t.type({ name: t.string }) |
tuple | [ A, B ] | t.tuple([ A, B ]) |
union | A | B | t.union([ A, B ]) or t.taggedUnion(tag, [ A, B ]) |
intersection | A & B | t.intersection([ A, B ]) |
keyof | keyof M | t.keyof(M) |
recursive types | see Recursive types | t.recursion(name, definition) |
refinement | ✘ | t.refinement(A, predicate) |
exact types | ✘ | t.exact(type) |
strict types (deprecated) | ✘ | t.strict({ name: t.string }) |
Recursive types can't be inferred by TypeScript so you must provide the static type as a hint
// helper type
interface ICategory {
name: string
categories: Array<ICategory>
}
const Category = t.recursion<ICategory>('Category', Category =>
t.type({
name: t.string,
categories: t.array(Category)
})
)
interface IFoo {
type: 'Foo'
b: IBar | undefined
}
interface IBar {
type: 'Bar'
a: IFoo | undefined
}
const Foo: t.RecursiveType<t.Type<IFoo>, IFoo> = t.recursion<IFoo>('Foo', _ =>
t.interface({
type: t.literal('Foo'),
b: t.union([Bar, t.undefined])
})
)
const Bar: t.RecursiveType<t.Type<IFoo>, IBar> = t.recursion<IBar>('Bar', _ =>
t.interface({
type: t.literal('Bar'),
a: t.union([Foo, t.undefined])
})
)
const FooBar = t.taggedUnion('type', [Foo, Bar])
If you are encoding tagged unions, instead of the general purpose union
combinator, you may want to use the
taggedUnion
combinator in order to get better performances
const A = t.type({
tag: t.literal('A'),
foo: t.string
})
const B = t.type({
tag: t.literal('B'),
bar: t.number
})
// the actual presence of the tag is statically checked
const U = t.taggedUnion('tag', [A, B])
You can refine a type (any type) using the refinement
combinator
const Positive = t.refinement(t.number, n => n >= 0, 'Positive')
const Adult = t.refinement(Person, person => person.age >= 18, 'Adult')
You can make a runtime type alias exact (which means that only the given properties are allowed) using the exact
combinator
const Person = t.type({
name: t.string,
age: t.number
})
const ExactPerson = t.exact(Person)
Person.decode({ name: 'Giulio', age: 43, surname: 'Canti' }) // ok
ExactPerson.decode({ name: 'Giulio', age: 43, surname: 'Canti' }) // fails
Note. This combinator is deprecated, use exact
instead.
You can make a runtime type strict (which means that only the given properties are allowed) using the strict
combinator
const Person = t.type({
name: t.string,
age: t.number
})
const StrictPerson = t.strict(Person.props)
Person.decode({ name: 'Giulio', age: 43, surname: 'Canti' }) // ok
StrictPerson.decode({ name: 'Giulio', age: 43, surname: 'Canti' }) // fails
You can mix required and optional props using an intersection
const A = t.type({
foo: t.string
})
const B = t.partial({
bar: t.number
})
const C = t.intersection([A, B])
type CT = t.TypeOf<typeof C>
// same as
type CT = {
foo: string
bar?: number
}
You can define your own types. Let's see an example
import * as t from 'io-ts'
// represents a Date from an ISO string
const DateFromString = new t.Type<Date, string>(
'DateFromString',
(m): m is Date => m instanceof Date,
(m, c) =>
t.string.validate(m, c).chain(s => {
const d = new Date(s)
return isNaN(d.getTime()) ? t.failure(s, c) : t.success(d)
}),
a => a.toISOString()
)
const s = new Date(1973, 10, 30).toISOString()
DateFromString.decode(s)
// right(new Date('1973-11-29T23:00:00.000Z'))
DateFromString.decode('foo')
// left(errors...)
Note that you can deserialize while validating.
No, however you can define your own logic for that (if you really trust the input)
import * as t from 'io-ts'
import { Either, right } from 'fp-ts/lib/Either'
const { NODE_ENV } = process.env
export function unsafeDecode<A, O>(value: t.mixed, type: t.Type<A, O>): Either<t.Errors, A> {
if (NODE_ENV !== 'production' || type.encode !== t.identity) {
return type.decode(value)
} else {
// unsafe cast
return right(value as A)
}
}
// or...
import { failure } from 'io-ts/lib/PathReporter'
export function unsafeGet<A, O>(value: t.mixed, type: t.Type<A, O>): A {
if (NODE_ENV !== 'production' || type.encode !== t.identity) {
return type.decode(value).getOrElseL(errors => {
throw new Error(failure(errors).join('\n'))
})
} else {
// unsafe cast
return value as A
}
}
Use keyof
instead of union
when defining a union of string literals
const Bad = t.union([
t.literal('foo'),
t.literal('bar'),
t.literal('baz')
// etc...
])
const Good = t.keyof({
foo: null,
bar: null,
baz: null
// etc...
})
Benefits
VS Code might display weird types for nested types
const NestedInterface = t.type({
foo: t.string,
bar: t.type({
baz: t.string
})
})
type NestedInterfaceType = t.TypeOf<typeof NestedInterface>
/*
Hover on NestedInterfaceType will display
type NestedInterfaceType = {
foo: string;
bar: t.TypeOfProps<{
baz: t.StringType;
}>;
}
instead of
type NestedInterfaceType = {
foo: string;
bar: {
baz: string
}
}
*/
clean
and alias
functionsThe pattern
// private runtime type
const _NestedInterface = t.type({
foo: t.string,
bar: t.type({
baz: t.string
})
})
// a type alias using interface
export interface NestedInterface extends t.TypeOf<typeof _NestedInterface> {}
//
// Two possible options for the exported runtime type
//
// a clean NestedInterface which drops the kind...
export const NestedInterface = t.clean<NestedInterface, NestedInterface>(_NestedInterface)
/*
NestedInterface: t.Type<NestedInterface, NestedInterface, t.mixed>
*/
// ... or an alias of _NestedInterface which keeps the kind
export const NestedInterface = t.alias(_NestedInterface)<NestedInterface, NestedInterface>()
/*
t.InterfaceType<{
foo: t.StringType;
bar: t.InterfaceType<{
baz: t.StringType;
}, t.TypeOfProps<{
baz: t.StringType;
}>, t.OutputOfProps<{
baz: t.StringType;
}>, t.mixed>;
}, NestedInterface, NestedInterface, t.mixed>
*/
// you can also alias the props
interface NestedInterfaceProps extends t.PropsOf<typeof _NestedInterface> {}
export const NestedInterface = t.alias(_NestedInterface)<NestedInterface, NestedInterface, NestedInterfaceProps>()
/*
const NestedInterface: t.InterfaceType<NestedInterfaceProps, NestedInterface, NestedInterface, t.mixed>
*/
1.2.1
FAQs
TypeScript runtime type system for IO decoding/encoding
The npm package io-ts receives a total of 975,517 weekly downloads. As such, io-ts popularity was classified as popular.
We found that io-ts demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
In an open letter, JavaScript community leaders urge Oracle to give up the JavaScript trademark, arguing that it has been effectively abandoned through nonuse.
Security News
The initial version of the Socket Python SDK is now on PyPI, enabling developers to more easily interact with the Socket REST API in Python projects.
Security News
Floating dependency ranges in npm can introduce instability and security risks into your project by allowing unverified or incompatible versions to be installed automatically, leading to unpredictable behavior and potential conflicts.