ipfs-bitswap


Node.js implementation of the Bitswap 'data exchange' protocol used by IPFS
Table of Contents
Install
npm
> npm install ipfs-bitswap --save
Use in Node.js
const Bitswap = require('ipfs-bitswap')
Use in a browser with browserify, webpack or any other bundler
The code published to npm that gets loaded on require is in fact a ES5 transpiled version with the right shims added. This means that you can require it and use with your favourite bundler without having to adjust asset management process.
const Bitswap = require('ipfs-bitswap')
Use in a browser using a script tag
Loading this module through a script tag will make the IpfsBitswap
object available in the global namespace.
<script src="https://unpkg.com/ipfs-bitswap/dist/index.min.js"></script>
<script src="https://unpkg.com/ipfs-bitswap/dist/index.js"></script>
Usage
For the documentation see API.md.
API
new Bitswap(libp2p, blockstore)
libp2p: Libp2p
, instance of the local network stack.blockstore: Blockstore
, instance of the local database (IpfsRepo.blockstore
)
Create a new instance.
getStream(cid)
Returns a source pull-stream
. Values emitted are the received blocks.
Example:
pull(
bitswap.getStream(cid),
pull.collect((err, blocks) => {
})
)
pull(
bitswap.getStream([cid1, cid2, cid3]),
pull.collect((err, blocks) => {
})
)
Note: This is safe guarded so that the network is not asked
for blocks that are in the local datastore
.
unwant(cids)
Cancel previously requested cids, forcefully. That means they are removed from the
wantlist independent of how many other resources requested these cids. Callbacks
attached to getBlock
are errored with Error('manual unwant: cid)
.
cancelWants(cids)
Cancel previously requested cids.
putStream()
Returns a duplex pull-stream
that emits an object {cid: CID}
for every written block when it was stored.
Objects passed into here should be of the form {data: Buffer, cid: CID}
put(blockAndCid, callback)
blockAndCid: {data: Buffer, cid: CID}
callback: Function
Announce that the current node now has the block containing data
. This will store it
in the local database and attempt to serve it to all peers that are known
to have requested it. The callback is called when we are sure that the block
is stored.
wantlistForPeer(peerId)
Get the wantlist for a given peer.
stat()
Get stats about about the current state of the bitswap instance.
Development
Structure

» tree src
src
├── components
│ ├── decision
│ │ ├── engine.js
│ │ ├── index.js
│ │ └── ledger.js
│ ├── network
│ │ └── index.js
│ └── want-manager
│ ├── index.js
│ └── msg-queue.js
├── constants.js
├── index.js
└── types
├── message
│ ├── entry.js
│ ├── index.js
│ └── message.proto.js
└── wantlist
├── entry.js
└── index.js
Contribute
Feel free to join in. All welcome. Open an issue!
This repository falls under the IPFS Code of Conduct.
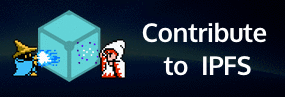
License
MIT