ipfs-bitswap

JavaScript implementation of the Bitswap 'data exchange' protocol used by IPFS
Lead Maintainer
Dirk McCormick
Table of Contents
Install
npm
> npm install ipfs-bitswap
Use in Node.js or in the browser with browserify, webpack or any other bundler
const Bitswap = require('ipfs-bitswap')
Use in a browser using a script tag
Loading this module through a script tag will make the IpfsBitswap
object available in the global namespace.
<script src="https://unpkg.com/ipfs-bitswap/dist/index.min.js"></script>
<script src="https://unpkg.com/ipfs-bitswap/dist/index.js"></script>
API
See https://ipfs.github.io/js-ipfs-bitswap
Stats
const bitswapNode =
const stats = bitswapNode.stat()
Stats contains a snapshot accessor, a moving average acessor and a peer accessor.
Besides that, it emits "update" events every time it is updated.
stats.on('update', (stats) => {
console.log('latest stats snapshot: %j', stats)
})
Peer accessor:
You can get the stats for a specific peer by doing:
const peerStats = stats.forPeer(peerId)
The returned object behaves like the root stats accessor (has a snapshot, a moving average accessors and is an event emitter).
Global snapshot accessor:
const snapshot = stats.snapshot
console.log('stats: %j', snapshot)
the snapshot will contain the following keys, with the values being bignumber.js instances:
Moving average accessor:
const movingAverages = stats.movingAverages
This object contains these properties:
- 'blocksReceived',
- 'dataReceived',
- 'dupBlksReceived',
- 'dupDataReceived',
- 'blocksSent',
- 'dataSent',
- 'providesBufferLength',
- 'wantListLength',
- 'peerCount'
const dataReceivedMovingAverages = movingAverages.dataReceived
Each one of these will contain one key per interval (miliseconds), being the default intervals defined:
- 60000 (1 minute)
- 300000 (5 minutes)
- 900000 (15 minutes)
You can then select one of them
const oneMinuteDataReceivedMovingAverages = dataReceivedMovingAverages[60000]
This object will be a movingAverage instance.
Development
Structure

» tree src
src
├── constants.js
├── decision-engine
│ ├── index.js
│ └── ledger.js
├── index.js
├── network.js
├── notifications.js
├─── want-manager
│ ├── index.js
│ └── msg-queue.js
└─── types
├── message
│ ├── entry.js
│ ├── index.js
│ └── message.proto.js
└── wantlist
├── entry.js
└── index.js
Performance tests
You can run performance tests like this:
$ npm run benchmarks
Profiling
You can run each of the individual performance tests with a profiler like 0x.
To do that, you need to install 0x:
$ npm install 0x --global
And then run the test:
$ 0x test/benchmarks/get-many
This will output a flame graph and print the location for it.
Use the browser Chrome to open and inspect the generated graph.

Contribute
Feel free to join in. All welcome. Open an issue!
This repository falls under the IPFS Code of Conduct.
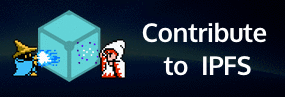
License
MIT