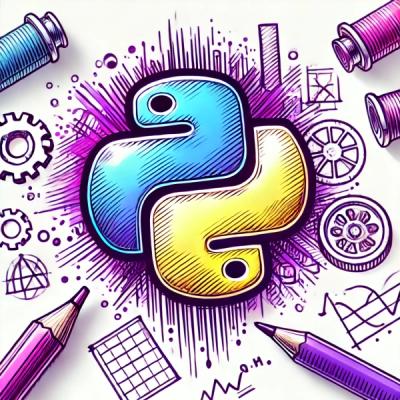
Security News
New Python Packaging Proposal Aims to Solve Phantom Dependency Problem with SBOMs
PEP 770 proposes adding SBOM support to Python packages to improve transparency and catch hidden non-Python dependencies that security tools often miss.
ipld-explorer-components
Advanced tools
React components for https://explore.ipld.io (https://github.com/ipfs/explore.ipld.io) and ipfs-webui
This module was extracted from the explore.ipld.io so it could be reused from the IPFS Web UI.
WARNING: This module is not intended to be re-used in it's current form by other projects. There is more work to do to make this a nice set of generic components.
Install it from npm:
npm install --save ipld-explorer-components
There are peerDependencies
so that the consuming app can pick the versions of common deps. You'll need to add relevant deps to your project.
You can see an example of how to use these components in the devPage.jsx file.
// index.tsx
import React from 'react'
import {render} from 'react-dom'
import MyHeader from './app'
const PageRenderer = (): React.ReactElement => {
/**
* This is a simple example of listening to the hash change event that occurs when the user clicks around in the content rendered by ExplorePage.
*/
const [route, setRoute] = useState(window.location.hash.slice(1) ?? '/')
useEffect(() => {
const onHashChange = (): void => { setRoute(window.location.hash.slice(1) ?? '/') }
window.addEventListener('hashchange', onHashChange)
return () => { window.removeEventListener('hashchange', onHashChange) }
}, [])
const RenderPage: React.FC = () => {
switch (true) {
case route.startsWith('/explore'):
return <ExplorePage />
case route === '/':
default:
return <StartExploringPage />
}
}
return (
<RenderPage />
)
}
const App = (): React.ReactElement => {
return (
<HeliaProvider>
<ExploreProvider>
<MyHeader />
<PageRenderer />
</ExploreProvider>
</HeliaProvider>
)
}
const rootEl = document.getElementById('root')
if (rootEl == null) {
throw new Error('No root element found with the id "root"')
}
const root = createRoot(rootEl)
root.render(
<I18nextProvider i18n={i18n}>
<App />
</I18nextProvider>
)
import { HeliaProvider, ExploreProvider } from 'ipld-explorer-components/providers'
import { StartExploringPage, ExplorePage } from 'ipld-explorer-components/pages'
import { IpldExploreForm, IpldCarExploreForm } from 'ipld-explorer-components/forms'
// or import all components at once
import { HeliaProvider, ExploreProvider, StartExploringPage, ExplorePage, IpldExploreForm, IpldCarExploreForm, CidInfo, ObjectInfo } from 'ipld-explorer-components'
The following Components are available:
export {
/**
* Helia provider required for IPLD Explorer components
*/
HeliaProvider,
/**
* A hook to gain access to the Helia node
*/
useHelia,
/**
* Explore provider required for IPLD Explorer components. This must be a child (direct or not) of HeliaProvider.
*/
ExploreProvider,
/**
* A hook to gain access to the Explore state. You can programmatically set the CID or path to explore using the provided functions.
*/
useExplore,
/**
* The page to render when you do not have an explicit CID in the URL to explore yet.
*/
StartExploringPage,
/**
* When there is a #/explore/CID in the URL, this component will render the ExplorePage
*/
ExplorePage,
/**
* The form to use to allow entry of a CID to explore. You can place this anywhere in your app within the ExploreProvider.
*/
IpldExploreForm,
/**
* The form to use to allow uploading of a CAR file to explore. You can place this anywhere in your app within the ExploreProvider.
*/
IpldCarExploreForm,
CidInfo,
ObjectInfo,
}
And, assuming you are using create-react-app
or a similar webpack set up, you'll need the following CSS imports:
import 'tachyons'
import 'ipfs-css'
import 'ipld-explorer-components/css'
To customize the links displayed in the start exploring page, you can pass a links
property to the StartExploringPage
component. This property should be an array of objects with the following properties:
{
name: 'Name of your example link',
cid: 'bafyfoo...',
type: 'dag-pb' // or dag-json, etc...
}
The translations used for this library are provided in dist/locales
. You can use them in your project by importing them and passing them to the i18n
instance in your project.
import i18n from 'i18next'
import LanguageDetector from 'i18next-browser-languagedetector'
import Backend from 'i18next-chained-backend'
import HttpBackend from 'i18next-http-backend'
import ICU from 'i18next-icu'
import LocalStorageBackend from 'i18next-localstorage-backend'
import { version } from '../package.json'
import locales from './lib/languages.json'
export const localesList = Object.values(locales)
await i18n
.use(ICU)
.use(Backend)
.use(LanguageDetector)
.init({
backend: {
backends: [
LocalStorageBackend,
HttpBackend
],
backendOptions: [
{ // LocalStorageBackend
defaultVersion: version,
expirationTime: (!import.meta.env.NODE_ENV || imObjectInfo.publicGatewayport.meta.env.NODE_ENV === 'development') ? 1 : 7 * 24 * 60 * 60 * 1000
},
{ // HttpBackend
// ensure a relative path is used to look up the locales, so it works when loaded from /ipfs/<cid>
loadPath: (lngs, namespaces) => {
const lang = lngs[0]
const ns = namespaces[0]
if (ns === 'explore') {
// use the ipld-explorer-components locales
return 'node_modules/ipld-explorer-components/dist/locales/{{lng}}/{{ns}}.json'
}
// you can override keys in the explore namespace with your own translations. If they are not found, the explore translations will be used.
return `locales/${lang}/${ns}.json`
}
}
]
},
ns: ['explore', 'app'],
defaultNS: 'app',
fallbackNS: 'explore', // fallback to explore namespace if the key is not found in the app namespace
fallbackLng: {
'zh-Hans': ['zh-CN', 'en'],
'zh-Hant': ['zh-TW', 'en'],
zh: ['zh-CN', 'en'],
default: ['en']
},
debug: import.meta.env.DEBUG,
// react i18next special options (optional)
react: {
// wait: true,
// useSuspense: false,
bindI18n: 'languageChanged loaded',
bindStore: 'added removed',
nsMode: 'default'
}
})
NOTE: PRs adding an old IPLDFormat codec would need the old blockcodec-to-ipld-format
tool, which has many out-of-date deps. We will only accept PRs for adding BlockCodec interface codecs.
To add another codec, you will need to update all locations containing the comment // #WhenAddingNewCodec
:
resolveIpldPath
returns the expected results
resolveFn
in ./src/lib/get-codec-for-cid.ts doesn't resolve your paths correctly, you will need to add a resolver method for your codec to the codecResolverMap
in ./src/lib/get-codec-for-cid.tssee https://github.com/ipfs/ipld-explorer-components/pull/360#discussion_r1206251817 for history.
To add another hasher, you will need to update all locations containing the comment // #WhenAddingNewHasher
:
SupportedHashers
to include your hasher typegetHasherForCode
to return your hasherhashers
property passed to Helia init in ./src/lib/init-helia.tssee https://github.com/ipfs/ipld-explorer-components/pull/395 for an example.
Feel free to dive in! Open an issue or submit PRs.
To contribute to IPFS in general, see the contributing guide.
tx pull -a
to pull the latest translations from Transifex (i18n#transifex-101))npm version major/minor/patch
)git push && git push --follow-tags
)npm publish
)MIT © Protocol Labs
FAQs
React components for https://explore.ipld.io
The npm package ipld-explorer-components receives a total of 28 weekly downloads. As such, ipld-explorer-components popularity was classified as not popular.
We found that ipld-explorer-components demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PEP 770 proposes adding SBOM support to Python packages to improve transparency and catch hidden non-Python dependencies that security tools often miss.
Security News
Socket CEO Feross Aboukhadijeh discusses open source security challenges, including zero-day attacks and supply chain risks, on the Cyber Security Council podcast.
Security News
Research
Socket researchers uncover how threat actors weaponize Out-of-Band Application Security Testing (OAST) techniques across the npm, PyPI, and RubyGems ecosystems to exfiltrate sensitive data.