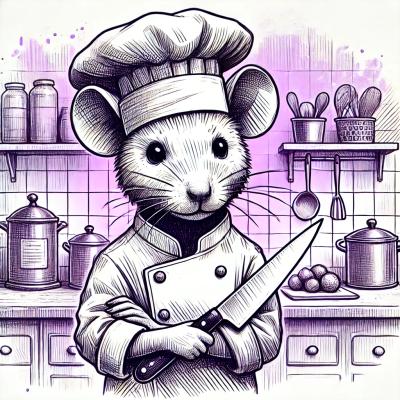
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
The is-cidr npm package is a utility for validating and parsing CIDR (Classless Inter-Domain Routing) notations. It helps in determining whether a given string is a valid CIDR notation for IPv4 or IPv6 addresses.
Validate IPv4 CIDR
This feature allows you to check if a given string is a valid IPv4 CIDR notation. The function returns true if the input is a valid IPv4 CIDR and false otherwise.
const isCidr = require('is-cidr');
console.log(isCidr.v4('192.168.0.1/24')); // true
console.log(isCidr.v4('192.168.0.1')); // false
Validate IPv6 CIDR
This feature allows you to check if a given string is a valid IPv6 CIDR notation. The function returns true if the input is a valid IPv6 CIDR and false otherwise.
const isCidr = require('is-cidr');
console.log(isCidr.v6('2001:db8::/32')); // true
console.log(isCidr.v6('2001:db8::')); // false
Validate both IPv4 and IPv6 CIDR
This feature allows you to check if a given string is a valid CIDR notation for either IPv4 or IPv6. The function returns 4 for valid IPv4 CIDR, 6 for valid IPv6 CIDR, and 0 for invalid CIDR.
const isCidr = require('is-cidr');
console.log(isCidr('192.168.0.1/24')); // 4
console.log(isCidr('2001:db8::/32')); // 6
console.log(isCidr('invalid-cidr')); // 0
The cidr-regex package provides regular expressions for matching CIDR notations. It can be used to validate and extract CIDR notations from strings. Unlike is-cidr, it focuses on pattern matching using regular expressions.
The ip-cidr package offers more comprehensive CIDR manipulation capabilities, including validation, parsing, and conversion between CIDR and IP ranges. It provides a broader set of functionalities compared to is-cidr, which is primarily focused on validation.
The netmask package is used for IP address and network mask manipulation. It allows for CIDR validation, subnet calculations, and IP range generation. It offers more advanced network-related functionalities compared to the simpler validation focus of is-cidr.
Check if a string is an IP address in CIDR notation
npm i is-cidr
import isCidr from "is-cidr";
isCidr("192.168.0.1/24"); //=> 4
isCidr("1:2:3:4:5:6:7:8/64"); //=> 6
isCidr("10.0.0.0"); //=> 0
isCidr.v6("10.0.0.0/24"); //=> false
Check if input
is a IPv4 or IPv6 CIDR. Returns either 4
, 6
(indicating the IP version) or 0
if the string is not a CIDR.
Check if input
is a IPv4 CIDR. Returns a boolean.
Check if input
is a IPv6 CIDR. Returns a boolean.
© silverwind, distributed under BSD licence
Based on previous work by Felipe Apostol
FAQs
Check if a string is an IP address in CIDR notation
The npm package is-cidr receives a total of 227,710 weekly downloads. As such, is-cidr popularity was classified as popular.
We found that is-cidr demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.