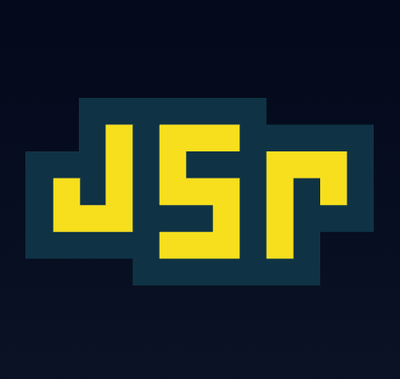
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
The is-hotkey npm package is a utility for detecting keyboard shortcuts in JavaScript applications. It allows developers to easily check if a specific key combination has been pressed, making it useful for implementing custom keyboard shortcuts in web applications.
Basic Hotkey Detection
This feature allows you to detect if a specific key combination, such as 'mod+s' (Ctrl+S on Windows/Linux or Command+S on macOS), has been pressed. The 'isHotkey' function takes a string representing the key combination and an event object, and returns true if the combination matches the event.
const isHotkey = require('is-hotkey');
if (isHotkey('mod+s', event)) {
console.log('Save command detected');
}
Custom Hotkey Detection
This feature allows you to create a custom hotkey detection function. The 'isHotkey' function can be used to create a reusable function that checks for a specific key combination. This is useful for adding multiple event listeners or for more complex hotkey logic.
const isHotkey = require('is-hotkey');
const customHotkey = isHotkey('ctrl+shift+k');
document.addEventListener('keydown', (event) => {
if (customHotkey(event)) {
console.log('Custom hotkey detected');
}
});
Multiple Hotkey Detection
This feature allows you to detect multiple hotkeys with a single function call. By passing an array of key combinations to the 'isHotkey' function, you can check if any of the specified combinations match the event.
const isHotkey = require('is-hotkey');
const hotkeys = ['mod+s', 'ctrl+shift+k'];
document.addEventListener('keydown', (event) => {
if (isHotkey(hotkeys, event)) {
console.log('One of the hotkeys detected');
}
});
Mousetrap is a simple library for handling keyboard shortcuts in JavaScript. It allows you to bind key combinations to specific functions and supports complex key sequences. Compared to is-hotkey, Mousetrap offers more features for handling keyboard shortcuts, such as key sequences and key combinations with modifiers.
Hotkeys-js is a robust library for managing keyboard shortcuts in web applications. It supports a wide range of key combinations and provides a simple API for binding and unbinding shortcuts. Compared to is-hotkey, hotkeys-js offers more advanced features like scope management and key sequence detection.
Keymaster is a lightweight library for defining and dispatching keyboard shortcuts. It provides a simple API for binding key combinations to functions and supports basic key sequences. Compared to is-hotkey, Keymaster is more focused on simplicity and ease of use, making it a good choice for smaller projects.
A simple way to check whether a browser event matches a hotkey.
mod+s
, cmd+alt+space
, etc.mod
for the classic "cmd
on Mac, ctrl
on Windows" use case.event.which
(default) or event.key
to work regardless of keyboard layout.< 1kb
minified and gzipped.The most basic usage...
import isHotkey from 'is-hotkey'
function onKeyDown(e) {
if (isHotkey('mod+s', e)) {
...
}
}
Or, you can curry the hotkey string for better performance, since it is only parsed once...
import isHotkey from 'is-hotkey'
const isSaveHotkey = isHotkey('mod+s')
function onKeyDown(e) {
if (isSaveHotkey(e)) {
...
}
}
That's it!
There are tons of hotkey libraries, but they're often coupled to the view layer, or they bind events globally, or all kinds of weird things. You don't really want them to bind the events for you, you can do that yourself.
Instead, you want to just check whether a single event matches a hotkey. And you want to define your hotkeys in the standard-but-non-trivial-to-parse syntax that everyone knows.
But most libraries don't expose their parsing logic. And even for the ones that do expose their hotkey parsing logic, pulling in an entire library just to check a hotkey string is overkill.
So... this is a simple and lightweight hotkey checker!
import isHotkey from 'is-hotkey'
isHotkey('mod+s')(event)
isHotkey('mod+s', { byKey: true })(event)
isHotkey('mod+s', event)
isHotkey('mod+s', { byKey: true }, event)
You can either pass hotkey, [options], event
in which case the hotkey will be parsed and compared immediately. Or you can passed just hotkey, [options]
to receive a curried checking function that you can re-use for multiple events.
isHotkey('mod+a')
isHotkey('Control+S')
isHotkey('⌘+d')
itHotkey('Meta+DownArrow')
itHotkey('cmd+down')
The API is case-insentive, and has all of the conveniences you'd expect—cmd
vs. Meta
, opt
vs. Alt
, down
vs. DownArrow
, etc.
It also accepts mod
for the classic "cmd
on Mac, ctrl
on Windows" use case.
import isHotkey from 'is-hotkey'
import { isCodeHotkey, isKeyHotkey } from 'is-hotkey'
isHotkey('mod+s')(event)
isHotkey('mod+s', { byKey: true })(event)
isCodeHotkey('mod+s', event)
isKeyHotkey('mod+s', event)
By default the hotkey string is checked using event.which
. But you can also pass in byKey: true
to compare using the KeyboardEvent.key
API, which stays the same regardless of keyboard layout.
Or to reduce the noise if you are defining lots of hotkeys, you can use the isCodeHotkey
and isKeyHotkey
helpers that are exported.
import { toKeyName, toKeyCode } from 'is-hotkey'
toKeyName('cmd') // "meta"
toKeyName('a') // "a"
toKeyCode('shift') // 16
toKeyCode('a') // 65
You can also use the exposed toKeyName
and toKeyCode
helpers, in case you want to add the same level of convenience to your own APIs.
import { parseHotkey, compareHotkey } from 'is-hotkey'
const hotkey = parseHotkey('mod+s', { byKey: true })
const passes = compareHotkey(hotkey, event)
You can also go even more low-level with the exposed parseHotkey
and compareHotkey
functions, which are what the default isHotkey
export uses under the covers, in case you have more advanced needs.
This package is MIT-licensed.
FAQs
Check whether a browser event matches a hotkey.
We found that is-hotkey demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.