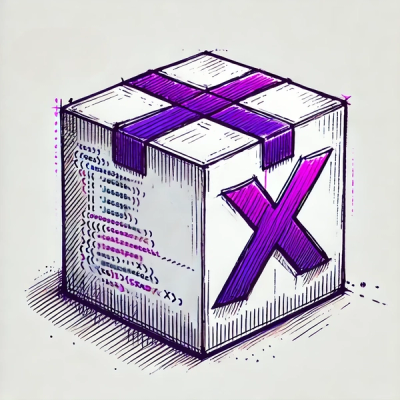
Security News
pnpm 10.0.0 Blocks Lifecycle Scripts by Default
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.
A type checking library where each exported function returns either true or false and does not throw. Also added tests.
is2 is a type-checking module for node.js to test values. Is does not throw exceptions and every function only returns true or false. Use is2 to validate types in your node.js code. Every function in is2 returns either true of false.
After finding Enrico Marino's module is, the concise syntax amazed, but there were syntax issues that made using is difficult. This fork of is fixes those issues, but the module is no longer cross-platform. Also, added tests via mocha which can be run using 'npm test'.
To install is2, type:
$ npm install is2
var is = require('is2');
console.log('true is equal to 1===1: '+(is.equal(true, 1===1));
console.log('10 is a positive number: '+(is.positiveNumber(10));
console.log('11 is an odd number: '+(is.oddNumber(11));
Test if 'value' is a type of 'type'. Alias: a
value value to test.
String type THe name of the type.
Test if 'value' is defined. Alias: def
Test is 'value' is either null or undefined. Alias: nullOrUndef
Test if 'value' is empty. To be empty means to be an array, object or string with nothing contained.
Do a deep comparision of two objects for equality. Will recurse without any limits. Meant to be called by equal only.
Object value The first object to compare.
Object other The second object to compare.
Test if 'value' is equal to 'other'. Works for objects and arrays and will do deep comparisions, using recursion. Alias: eq
Any value value.
Any other value to compare with.
JS Type definitions which cannot host values.
Test if 'key' in host is an object. To be hosted means host[value] is an object.
Any value The value to test.
Any host Host that may contain value.
Test if 'value' is an instance of 'constructor'. Aliases: instOf, instanceof
Test if 'value' is an instance of Buffer. Aliases: instOf, instanceof
Test if 'value' is null.
Test if 'value' is undefined. Aliases: undef, udef
Test if 'value' is an arguments object. Alias: args
Test if 'value' is an arguments object that is empty. Alias: args
Test if 'value' is an array. Alias: ary, arry
Test if 'value' is an array containing at least 1 entry. Aliases: nonEmptyArry, nonEmptyAry
Test if 'value' is an array containing no entries. Aliases: emptyArry, emptyAry
Test if 'value' is an empty array(like) object. Aliases: arguents.empty, args.empty, ary.empty, arry.empty
Test if 'value' is an arraylike object (i.e. it has a length property with a valid value) Aliases: arraylike, arryLike, aryLike
Test if 'value' is a boolean. Alias: bool
Test if 'value' is false.
Test if 'value' is true.
Test if 'value' is a date.
Test if 'value' is an error object. Alias: err
Test if 'value' is a function. Alias: func
Test if 'value' is a number. Alias: num
Test if 'value' is a positive number. Alias: positiveNum, posNum
Test if 'value' is a negative number. Aliases: negNum, negativeNum
Test if 'value' is a decimal number. Aliases: decimalNumber, decNum
Test if 'value' is divisible by 'n'. Alias: divisBy
Number value value to test.
Number n dividend.
Test if 'value' is an integer. Alias: integer
Test if 'value' is a positive integer. Alias: posInt
Test if 'value' is a negative integer. Aliases: negInt, negativeInteger
Test if 'value' is greater than 'others' values. Alias: max
Number value value to test.
Array others values to compare with.
Test if 'value' is less than 'others' values. Alias: min
Number value value to test.
Array others values to compare with.
is.nan
Test if value
is not a number.
value
is not a number, false otherwiseTest if 'value' is an even number.
Test if 'value' is an odd number.
Test if 'value' is greater than or equal to 'other'. Aliases: greaterOrEq, greaterOrEqual
Number value value to test.
Number other value to compare with.
Test if 'value' is greater than 'other'. Aliases: greaterThan
Number value value to test.
Number other value to compare with.
Test if 'value' is less than or equal to 'other'. Alias: lessThanOrEq, lessThanOrEqual
Number value value to test
Number other value to compare with
Test if 'value' is less than 'other'. Alias: lessThan
Number value value to test
Number other value to compare with
Test if 'value' is within 'start' and 'finish'. Alias: withIn
Number value value to test.
Number start lower bound.
Number finish upper bound.
Test if 'value' is an object. Note: Arrays, RegExps, Date, Error, etc all return false. Alias: obj
Test if 'value' is an object with properties. Note: Arrays are objects. Alias: nonEmptyObj
Test if 'value' is an instance type objType. Aliases: objInstOf, objectinstanceof, instOf, instanceOf
object objInst an object to testfor type.
object objType an object type to compare.
Test if 'value' is a regular expression. Alias: regexp
Test if 'value' is a string. Alias: str
Test if 'value' is an empty string. Alias: emptyStr
Test if 'value' is a non-empty string. Alias: nonEmptyStr
FAQs
A type checking library where each exported function returns either true or false and does not throw. Also added tests.
The npm package is2 receives a total of 1,015,709 weekly downloads. As such, is2 popularity was classified as popular.
We found that is2 demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.
Product
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
Research
Security News
Socket researchers have discovered multiple malicious npm packages targeting Solana private keys, abusing Gmail to exfiltrate the data and drain Solana wallets.