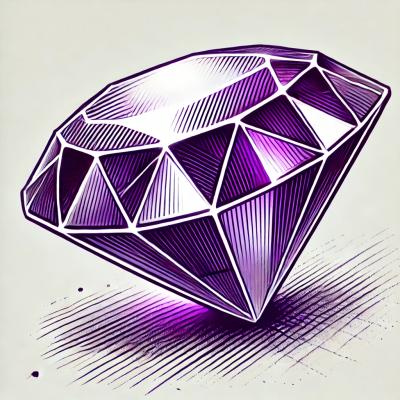
Security News
RubyGems.org Adds New Maintainer Role
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Isite Help You To Create Your Node Js WebSite with Advanced Development Featuers
- Auto Routes [Simple & Advanced & Custom]
- Merge Multi Files Contents in One Route
- Auto Handle Request & Response Headers [Cookies - Parameters]
- Auto Detect & Configer User Session
- Easy Creating Master Pages
- Auto Caching & Management Files in Memory
- Fast Read Files Content [Site Folder Structure]
- Custom Html Attributes [Server Tags]
- MongoDB Full Integration
- MD5 Hash Function
npm install isite --save
var isite = require('isite')
var site = isite()
site.run()
Advanced Using
var isite = require('isite')
site = isite({
port: 8080, // default 80
dir: __dirname + '/site_files', //default ./site_files
savingTime: 60 * 60, // default 60 * 60 - 1 hour
sessionEnabled: true, // default true
mongodbEnabled: true, // default false
mongodbURL: '127.0.0.1:27017' // default 127.0.0.1:27017 - local
});
site.run()
Easy and Auto Site Routing
site.addRoute({name: '/css/bootstrap.css',path: site.dir + '/css/bootstrap.min.css'});
site.addRoute({
name: '/js/script.js',
path: [site.dir + '/js/jquery.js' , site.dir + '/js/bootstrap.js']
});
site.addRoute({name: '/',path: site.dir + '/html/index.html'});
site.addRoute({name: '/api',path: site.dir + '/json/employees.json' , method:'POST'});
Advanced Site Routing
site.addRoute({
name: '/',
callback: function (req, res) {
res.setHeader('Content-type', 'text/html');
res.writeHead(200);
site.html('index', function (err, content) {
res.end(content);
});
}
});
site.addRoute({
name: '/api',
method: 'POST',
callback: function (req, res) {
res.setHeader('Content-type', 'application/json');
res.writeHead(200);
site.json('index', function (err, content) {
res.end(content);
});
}
});
Custom Route - Using * [any letters]
site.get('/post/*', function(req, res) {
res.end('Any Route like /post/11212154545 ')
})
site.get('*', function(req, res) {
res.end('Any Route Requested Not Handled Before This Code')
})
Request Parameters [GET , POST | PUT | Delete]
site.get('/api', function(req, res) {
res.end('GET | id : ' + req.url.query.id)
})
site.post('/api', function(req, res) {
res.end('POST | id : ' + req.body.id + ' , Name : ' + req.body.name)
})
site.put('/api', function(req, res) {
res.end('PUT | id : ' + req.body.id + ' , Name : ' + req.body.name)
})
site.delete('/api', function(req, res) {
res.end('Delete | id : ' + req.body.id)
})
site.all('/api', function(req, res) {
res.end('Any Request Type Not Handled : ' + req.method)
})
Dynamic Parameters
site.get('/api/:post_id/category/:cat_id', function(req, res) {
res.end('GET | postId : ' + req.url.query.post_id + ', catId : ' + req.url.query.cat_id)
})
//Example : http://127.0.0.1:7070/api/123456/category/99
MVC Custom Route
site.get("/:controller/:action/:arg1/:arg2", function(req, res) {
res.end(
"GET | Controller : " +
req.url.query.controller +
", Action : " +
req.url.query.action +
", Arg 1 : " +
req.url.query.arg1 +
", Arg 2 : " +
req.url.query.arg2
);
});
site.get('/api/set' , function(req , res){
req.cookie.set('id' , 'userid')
res.end('cookie set ok !!')
})
site.get('/api/get' , function(req , res){
res.end(' id : ' + req.cookie.get('id'))
})
site.get('/login', function(req, res) {
req.session.set('username', 'amr barakat')
res.end('loged ok !! ')
})
site.get('/userInfo', function(req, res) {
var userName = req.session.get('username')
res.end(userName)
})
site.html('index', function (err, content) {
console.log(content);
});
site.css('bootstrap', function (err, content) {
console.log(content);
});
site.js('jquery', function (err, content) {
console.log(content);
});
site.json('items', function (err, content) {
console.log(content);
});
add Custom Master Page And Using it ..
site.addMasterPage({
name: 'masterPage1',
header: site.dir + '/html/header.html',
footer: site.dir + '/html/footer.html'
})
site.get({
name: '/ContactUs',
masterPage : 'masterPage1',
path: site.dir + '/html/contact.html',
parser: 'html'
});
Add Custom Html Content
site.addRoute({name: '/',path: site.dir + '/html/index.html' , parser:'html'});
<div x-import="navbar.html"></div>
<div class="container">
<h2 > ... </h2>
<p x-import="info.html"></p>
</div>
Page "navbar.html" & "info.html" Must Be In HTML Site Folder ['/site_files/html/']
site.addVar('siteName', 'First Site With Isite Library ');
site.addVar('siteBrand', 'XSite');
<title>##var.siteName##</title>
<h2>##var.siteBrand##</h2>
//Create New Collection & Insert New Docs
site.post({
name: '/db',
callback: function (req, res) {
site.mongodb.connectDB('company', function (err, db) {
if (!err) {
db.createCollection('employees')
db.collection('employees').insertMany([{
name: 'Name 1',
phone: 'xxx'
},
{
name: 'Name 2',
phone: 'xxxxxx'
},
{
name: 'Name 3',
phone: 'xxxxxxxxxxx'
}
], function (err, result) {
db.close()
res.end('Data Inserted : ' + result.insertedCount)
})
} else {
res.end(err.message)
}
})
}
});
//Select Docs
site.get({
name: '/db',
callback: function (req, res) {
site.mongodb.connectDB('company', function (err, db) {
if (!err) {
db.collection("employees").find({}).toArray(function (err, result) {
if (!err) {
res.end(JSON.stringify(result))
}
db.close()
});
} else {
res.end(err.message)
}
})
}
});
// Update Docs
site.put({
name: '/db',
callback: function (req, res) {
site.mongodb.connectDB('company', function (err, db) {
if (!err) {
db.collection("employees").updateMany({
name: 'Name 1'
}, {
$set: {
name: "Updated Name"
}
}, function (err, result) {
if (!err) {
res.end('Data Updated : ' + result.result.nModified)
}
db.close()
});
} else {
res.end(err.message)
}
})
}
});
//Delete Docs
site.delete({
name: '/db',
callback: function (req, res) {
site.mongodb.connectDB('company', function (err, db) {
if (!err) {
db.collection("employees").deleteMany({}, function (err, result) {
if (!err) {
res.end('Data Deleted !!')
}
db.close()
});
} else {
res.end(err.message)
}
})
}
});
site.md5('this content will be hashed as md5')
FAQs
Create High Level Multi-Language Web Site [Fast and Easy]
The npm package isite receives a total of 29 weekly downloads. As such, isite popularity was classified as not popular.
We found that isite demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.
Security News
Research
Socket's threat research team has detected five malicious npm packages targeting Roblox developers, deploying malware to steal credentials and personal data.