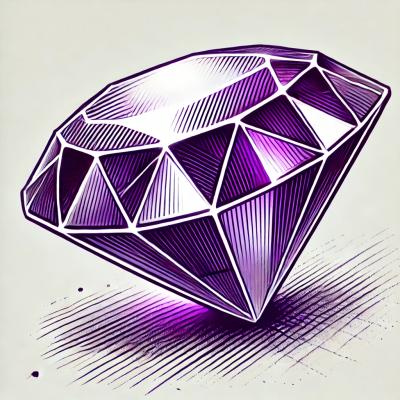
Security News
RubyGems.org Adds New Maintainer Role
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Isite Help You To Create Your Node Js WebSite with Advanced Development Featuers
- Auto Routes [Simple & Advanced & Custom]
- Auto Handle File Types Encoding [Fonts - Images - ...]
- Merge Multi Files Contents in One Route
- Auto Handle Request & Response Headers [Cookies - Parameters]
- Auto Detect & Configer User Session
- Easy Creating Master Pages
- Auto Caching & Management Site Files in Memory
- Fast Read Files Content [Site Folder Structure]
- Custom Html Attributes [Server Tags]
- MongoDB Full Integration
- Client libraries [jquery - bootstrap - font-awesome - angular]
- Development Helper Functions
- Site Dynamic Events Callback
npm install isite --save
Fast Startup Web Server
var isite = require('isite')
var site = isite({port:8080})
site.run()
Advanced Using with Custom Options
var isite = require('isite')
site = isite({
port: 8080,
dir: __dirname + "/site_files",
savingTime: 60,
admin: {
name: 'admin',
password: 'admin'
},
cache: {
js: 60 * 24 * 30,
css: 60 * 24 * 30,
images: 60 * 24 * 30,
fonts: 60 * 24 * 30
},
session: {
enabled: true,
storage: "mongodb",
dbName: "sessions",
userSessionCollection: "user_sessions"
},
security: {
enabled: true,
storage: "mongodb",
dbName: "security",
userCollection: "users"
},
mongodb: {
enabled: true,
prefix : '',
url: "127.0.0.1",
port: "27017",
userName: "",
password: "",
dbName : '',
collectionName : ''
}
});
site.run()
site.html('index', function (err, content) {
console.log(content);
});
site.css('bootstrap', function (err, content) {
console.log(content);
});
site.js('jquery', function (err, content) {
console.log(content);
});
site.json('items', function (err, content) {
console.log(content);
});
site.xml('rss', function (err, content) {
console.log(content);
});
-Custom Read Files
- Read From Local File in First Time
- Secound Read Will Read From Memory
//read file with custom header
site.get("/rss", function(req, res) {
site.readFile(__dirname + "/site_files/xml/rss.xml", function(err, content) {
res.writeHead(200, { "content-type": "text/xml" })
res.end(content)
})
})
site.get("/rss2", function(req, res) {
site.xml("rss2", function(err, content) {
res.writeHead(200, { "content-type": "text/xml" })
res.end(content);
})
})
//read multi files with custom header
site.get("/", function(req, res) {
site.readFiles(
[
__dirname + "/site_files/html/head.html",
__dirname + "/site_files/html/content.html",
__dirname + "/site_files/html/footer.html"
],
function(err, content) {
res.writeHead(200, { "content-type": "text/html" });
res.end(content);
})
})
Easy and Auto Site Routing
site.get({name: '/',path: site.dir + '/html/index.html'});
site.get({name: '/css/bootstrap.css',path: site.dir + '/css/bootstrap.min.css'});
site.get({name: '/js/jquery.js',path: site.dir + '/js/jquery.js'});
site.get({name: '/js/bootstrap.js',path: site.dir + '/js/bootstrap.js'});
site.get({name: '/favicon.png',path: site.dir + '/images/logo.png'})
site.post({name: '/api',path: site.dir + '/json/employees.json' });
Merge Multi Files in one route
site.get({
name: '/css/style.css',
path: [site.dir + '/css/bootstrap.css' , site.dir + '/css/custom.css']
});
site.get({
name: '/js/script.js',
path: [site.dir + '/js/jquery.js' , site.dir + '/js/bootstrap.js', site.dir + '/js/custom.js']
});
Advanced Site Routing
site.addRoute({
name: '/',
method: 'custom method', // dfeault - GET
path: site.dir + '/html/index.html', // default null
parser: 'html', // default static
cache: false // default true
});
site.addRoute({
name: '/',
method: 'custom method', // dfeault - GET
callback: function(req, res) {
res.setHeader('Content-type', 'text/html');
res.writeHead(200);
site.html('index', function(err, content) {
res.end(content);
});
}
});
Auto Route All Files in Folder
site.get({name: '/js', path: __dirname + '/js'})
site.get({name: '/css', path: __dirname + '/css'})
Custom Route - Using * [any letters]
site.get('/post/*', function(req, res) {
res.end('Any Route like /post/11212154545 ')
})
site.get('*', function(req, res) {
res.end('Any Route Requested Not Handled Before This Code')
})
Request Parameters [GET , POST | PUT | Delete] Restful API
site.get('/api', function(req, res) {
res.end('GET | id : ' + req.query.id)
})
site.post('/api', function(req, res) {
res.end('POST | id : ' + req.body.id + ' , Name : ' + req.body.name)
})
site.put('/api', function(req, res) {
res.end('PUT | id : ' + req.body.id + ' , Name : ' + req.body.name)
})
site.delete('/api', function(req, res) {
res.end('Delete | id : ' + req.body.id)
})
site.all('/api', function(req, res) {
res.end('Any Request Type Not Handled : ' + req.method)
})
Dynamic Parameters
site.get('/post/:id/category/:cat_id', function(req, res) {
res.end('GET | Id : ' + req.params.id + ', catId : ' + req.params.cat_id)
})
//example : /post/9999999/category/5
MVC Custom Route
site.get("/:controller/:action/:arg1", function(req, res) {
res.end(
"GET | Controller : " + req.params.controller +
", Action : " + req.params.action +
", Arg 1 : " + req.params.arg1
);
});
//example : /facebook/post/xxxxxxxxxx
site.get("/setCookie", function(req, res) {
req.cookie.set('name', req.query.name)
res.end('cookie set')
})
//example : /setcookie?name=amr
site.get("/getCookie", function(req, res) {
res.end('name from cookie : ' + req.cookie.get('name'))
})
//example : /getcookie
site.get('/setSession', function(req, res) {
req.session.set('user_name', req.query.user_name)
res.end('Session Set ok !! ')
})
//example : /setSession?user_name=absunstar
site.get('/getSession', function(req, res) {
res.end('User Name from session : ' + req.session.get('user_name'))
})
//example : /getSession
add Custom Master Page And Using it ..
site.addMasterPage({
name: 'masterPage1',
header: site.dir + '/html/header.html',
footer: site.dir + '/html/footer.html'
})
site.get({
name: '/ContactUs',
masterPage : 'masterPage1',
path: site.dir + '/html/contact.html',
parser: 'html'
});
Add Custom Html Content
site.addRoute({name: '/',path: site.dir + '/html/index.html' , parser:'html'});
<div x-import="navbar.html"></div>
<div class="container">
<h2 > ... </h2>
<p x-import="info.html"></p>
</div>
Page "navbar.html" & "info.html" Must Be In HTML Site Folder ['/site_files/html/']
site.addVar('siteName', 'First Site With Isite Library ');
site.addVar('siteBrand', 'XSite');
<title>##var.siteName##</title>
<h2>##var.siteBrand##</h2>
- Manage Closed Connections and Timeout
- Manage Multi Connections
- Manage Bulk Inserts & Updates & Deletes
- User Friendly Coding
// Insert One Doc
site.mongodb.insertOne({
dbName: 'company',
collectionName: 'employess',
doc:{name:'amr',salary:35000}
}, function (err, docInserted) {
if (err) {
console.log(err.message)
} else {
console.log(docInserted)
}
})
// Insert Many Docs
site.mongodb.insertMany({
dbName: 'company',
collectionName: 'employess',
docs:[
{name:'amr',salary:35000} ,
{name:'Gomana',salary:9000} ,
{name:'Maryem',salary:7000}
]
}, function (err, result) {
if (err) {
console.log(err.message)
} else {
console.log(result)
}
})
// Find One Doc
site.mongodb.findOne({
dbName: 'company',
collectionName: 'employees',
where:{},
select : {}
}, function (err, doc) {
if (err) {
console.log(err.message)
} else {
console.log(doc)
}
})
// Find Many Docs
site.mongodb.findMany({
dbName: 'company',
collectionName: 'employees',
where:{},
select : {}
}, function (err, docs) {
if (err) {
console.log(err.message)
} else {
console.log(docs)
}
})
//Update One Doc
site.mongodb.updateOne({
dbName: 'company',
collectionName: 'employees',
where:{salary:7000},
set : {name:'New MARYEM'}
}, function (err, result) {
if (err) {
console.log(err.message)
} else {
console.log(result)
}
})
// Update Many Docs
site.mongodb.updateMany({
dbName: 'company',
collectionName: 'employees',
where:{salary:9000},
set : {salary:9000 * .10}
}, function (err, result) {
if (err) {
console.log(err.message)
} else {
console.log(result)
}
})
// Delete One Doc
site.mongodb.deleteOne({
dbName: 'company',
collectionName: 'employess',
where:{_id: new site.mongodb.ObjectID('df54fdt8h3n48ykd136vg')}
}, function (err, result) {
if (err) {
console.log(err.message)
} else {
console.log(result)
}
})
// Delete Many Docs
site.mongodb.deleteMany({
dbName: 'company',
collectionName: 'employess',
where:{name : /a/}
}, function (err, result) {
if (err) {
console.log(err.message)
} else {
console.log(result)
}
})
Easy Access Client libraries - Required Fonts files auto Added
<link rel="stylesheet" href="/@css/bootstrap3.css" >
<link rel="stylesheet" href="/@css/font-awesome.css" >
<script src="/@js/jquery.js"></script>
<script src="/@js/bootstrap3.js"></script>
<script src="/@js/angular.js"></script>
var hash = site.md5('this content will be hashed as md5')
console.log(hash)
var name = 'absunstar'
if (name.like('*sun*')) {
console.log('yes')
}
##Events
site.on('event name', function() {
console.log('you call event name')
})
site.call('event name')
FAQs
Create High Level Multi-Language Web Site [Fast and Easy]
The npm package isite receives a total of 29 weekly downloads. As such, isite popularity was classified as not popular.
We found that isite demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.
Security News
Research
Socket's threat research team has detected five malicious npm packages targeting Roblox developers, deploying malware to steal credentials and personal data.