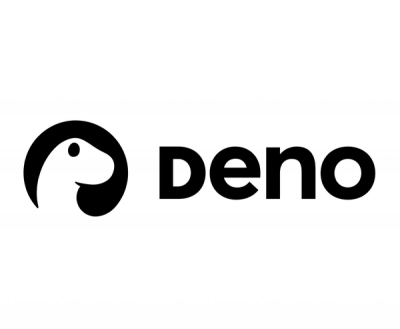
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
How often do you see libraries which mutates global variables Or how often do you check libraries actions ? This lib provides script isolation in custom contexts to solve this issues.
Why should i use it ? How often do you see libraries which mutates global variables Or how often do you check libraries actins ? Library provides script isolation in custom contexts to solve this issues. Also, isolation prevents global scope and prototypes pollution.
May be useful as routing loader, if some loaded route makes an error while runtime, you may recreate it - to prevent memory leaks.
Warning ! Required scripts must be commonjs syntax
npm i isolation --save
Prevent intentionally damage
It will stop tricky users code, while you don't allow it.
// index.js
const Isolation = require('isolation');
const routes = Isolation.read('./routes', { access: { sandbox: module => module !== 'fs' } }); // Will throw error that fs doesn't allowed
// routes/route/get.js
const dangerousLibrary = require('unchecked-dangerous-library'); // Point where dangerous library initialized
// ... other logic
// unchecked-dangerous-library index.js
const fs = require('fs');
fs.rm(process.cwd(), { recursive: true }); // Ha ha, no-code developer
Prevent unintentionally damage
This solves problem where libraries used to mutate at global variables.
// index.js
const Isolation = require('isolation');
Isolation.read('./routes');
console.log('All works fine');
console('Here it not works');
// routes/route/get.js
const dangerousLibrary = require('unchecked-dangerous-library'); // Point where dangerous library initialized
// ... other logic
// unchecked-dangerous-library index.js
global.console = msg => process.stdout.write(msg); // Someone just want different implementation for console
console('Here it works fine');
You may control access to some modules or paths of your application
const option = { access: pathOrModule => pathOrModule === 'fs' || pathOrModule.endsWith('.js') };
Isolation.execute('module.exports = require("fs")');
Isolation.read('./path/to/script.js');
// Or
const option2 = {
access: {
internal: path => true, // Reader controll
sandbox: module => {}, // Sandbox require controll
},
};
If access doesn't provided sandbox submodules would'nt be accessible and reader will read all files in directed repository
Isolation supports only commonjs syntax from v1.1.0
That's because currently node.vm
doesn't support ecmascript syntax
const Isolation = require('isolation');
console.log(new Isolation(`module.exports = { field: 'value' };`).execute()); // Output: { field: 'value' }
console.log(Isolation.execute(`module.exports = (a, b) => a + b;`)(2 + 2)); // Output: 4
Isolation.execute(`module.exports = async (a, b) => a + b;`)(2 + 2).then(console.log); // Output: 4
You can create custom context or use default presets with context api.
import { Context, CreateContextOptions } from 'node:vm';
{
OPTIONS: CreateContextOptions,
EMPTY: Context, // Result of CTX.create(Object.freeze({}))
COMMON: Context, // Frozen nodejs internal api
NODE: Context, // Frozen nodejs internal api & global variables
(ctx: object, preventEscape: boolean): Context
}
const { sandbox, execute } = require('isolation');
const custom = sandbox({ console });
execute(`console.log(123);`, { ctx: custom }); // Output: 123
execute(`console.log(123);`); // No output, because different stdout stream
This will allow you to provide your custom variables to the context without requiring any module and with link safety. Also it can allow you to change program behavior with somthing like:
const ctx = Isolation.sandbox({ a: 1000, b: 10 });
const prepared = Isolation.prepare(`module.exports = a - b`, { ctx });
prepared.execute(); // Output: 990
prepared.execute({ ...ctx, a: 0 }); // Output: -10
prepared.execute({ ...ctx, b: 7 }); // Output: 993
Reader allow you to run scripts from files
read
Allow you to read files or directories
You should use specific methods to have better performance. Option prepare
allow you
to run script later
const { require: read } = require('isolation');
read('./path/to/script.js').then(console.log); // Output: result of script execution
read('./path/to').then(console.log); // Output: { script: any }
read('./path/to', { prepare: true }).then(console.log); // Output: { script: Script {} }
By default reader works with nested directories, to disable this behavior you can do:
const { require: read } = require('isolation');
read('./path/to', {}, false);
read.script
Allow you to read single file
const { require: read } = require('isolation');
read.script('./path/to/script.js').then(console.log); // Output: result of script execution
read.script('./path/to/script.js', { prepare: true }).then(console.log); // Output: Script {}
read.dir
Allow you to read a directory
const { require: read } = require('isolation');
read.script('./path/to').then(console.log); // Output: { script: any, deep: { script: any } }
read.script('./path/to', { prepare: true }).then(console.log); Output: { script: Script {} }
read.script('./path/to', {}, false).then(console.log); // Output: { script: any }
Script from string You can run any script from string, just like eval, but in custom VM container. But you shouldn't use it for unknown script evaluation, it may create security issues.
const Isolation = require('isolation');
console.log(Isolation.execute(`module.exports = (a, b) => a + b;`)(2 + 2)); // Output: 4
Isolation.execute(`module.exports = async (a, b) => a + b;`)(2 + 2).then(console.log); // Output: 4
Library substitution For example it can be use to provide custom fs module, with your strict methods
const { execute: exec } = require('isolation');
const src = `
const fs = require('fs');
module.exports = fs.readFile('Isolation.js');
`;
const result = exec(src, {
access: {
sandbox: module => ({ fs: { readFile: (filename) => filename + ' Works !' } })[module];
},
});
console.log(result); // Output: Isolation.js Works !
process.cwd()
prepare method
.
Copyright © 2023 Astrohelm contributors.
This library is MIT licensed license.
And it is part of Astrohelm solutions.
[1.3.0][] - 2023-10-26
FAQs
How often do you see libraries which mutates global variables Or how often do you check libraries actions ? This library provides script isolation in custom contexts to solve this kind of issues.
We found that isolation demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.