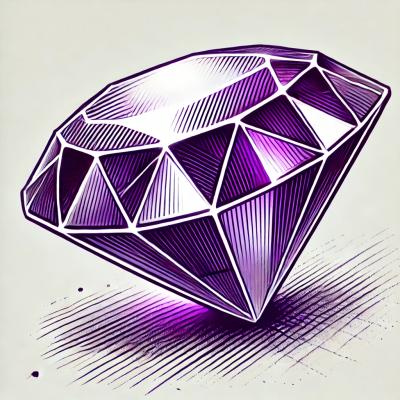
Security News
RubyGems.org Adds New Maintainer Role
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
isomorphic-git
Advanced tools
isomorphic-git is a pure JavaScript implementation of Git that works in both Node.js and browser environments. It provides a wide range of Git functionalities, allowing developers to perform Git operations without relying on native Git installations.
Clone a Repository
This feature allows you to clone a Git repository. The code sample demonstrates how to clone the isomorphic-git repository into a local directory named '/tutorial'.
const git = require('isomorphic-git');
const fs = require('fs');
(async () => {
await git.clone({
fs,
dir: '/tutorial',
url: 'https://github.com/isomorphic-git/isomorphic-git',
singleBranch: true,
depth: 1
});
console.log('Cloned the repository!');
})();
Commit Changes
This feature allows you to commit changes to a repository. The code sample demonstrates how to commit changes in the '/tutorial' directory with a commit message 'Initial commit'.
const git = require('isomorphic-git');
const fs = require('fs');
(async () => {
await git.commit({
fs,
dir: '/tutorial',
author: {
name: 'Your Name',
email: 'you@example.com'
},
message: 'Initial commit'
});
console.log('Committed changes!');
})();
Push to Remote
This feature allows you to push changes to a remote repository. The code sample demonstrates how to push changes from the '/tutorial' directory to the 'main' branch of the remote repository 'origin'.
const git = require('isomorphic-git');
const fs = require('fs');
(async () => {
await git.push({
fs,
dir: '/tutorial',
remote: 'origin',
ref: 'main',
token: 'your-github-token'
});
console.log('Pushed to remote!');
})();
Fetch from Remote
This feature allows you to fetch changes from a remote repository. The code sample demonstrates how to fetch changes from the 'origin' remote repository into the '/tutorial' directory.
const git = require('isomorphic-git');
const fs = require('fs');
(async () => {
await git.fetch({
fs,
dir: '/tutorial',
remote: 'origin'
});
console.log('Fetched from remote!');
})();
List Files in a Commit
This feature allows you to list files in a specific commit. The code sample demonstrates how to read the commit object for the 'HEAD' commit and log the tree of files.
const git = require('isomorphic-git');
const fs = require('fs');
(async () => {
const commit = await git.readCommit({
fs,
dir: '/tutorial',
oid: 'HEAD'
});
console.log(commit.commit.tree);
})();
NodeGit is a native Node.js binding to the libgit2 library, providing a comprehensive set of Git functionalities. Unlike isomorphic-git, NodeGit relies on native bindings and is not designed to work in browser environments.
Simple-git is a lightweight wrapper around the Git command line interface, providing a simple API for common Git operations. It requires Git to be installed on the system and is not designed to work in browser environments, unlike isomorphic-git.
Dugite provides a simplified interface for using Git in Node.js applications. It bundles a specific version of Git with the package, ensuring consistent behavior across different environments. However, it does not support browser environments like isomorphic-git.
A pure JavaScript implementation of git for node and browsers!
It works with any modern browser (see list above) and uses BrowserFS to emulate the node 'fs' module. This means client-side JavaScript can be used to read and write to the web the same way you've been editing websites on your desktop since 2008 - using git.
Isomorphic-git does not impliment every feature found in the canonical git implementation. But it does aim for 100% compatibility. This means it does all its operations by modifying files in a ".git" directory just like the git you are used to. You can even use the isogit
CLI to operate on existing git repositories on your desktop or server.
Table of Contents generated with DocToc
git()
API
isomorphic-git
?If you're only using isomorphic-git
in Node, you already have a fs
module, so you can skip this step. If you're writing code for the browser though, you'll need something that emulates the fs
API. isomorphic-git
will look for a global "fs" variable. At the time of writing, the most complete option is BrowserFS.
Here's a quick config that works well in most cases:
<script src="https://unpkg.com/browserfs"></script>
<script>
BrowserFS.configure({ fs: "IndexedDB", options: {} }, err => {
if (err) {
alert(err);
} else {
window.fs = BrowserFS.BFSRequire("fs");
}
});
</script>
Besides IndexedDB, BrowserFS supports many different backends with different performance characteristics, as well as advanced configurations such as: multiple mounting points, and overlaying a writeable filesystems on top of a read-only filesystem. You don't need to know about all these features, but familiarizing yourself with the different options may be necessary if you hit a storage limit or performance bottleneck in the IndexedDB backend I suggested above.
If you want, you can just throw in a script tag with the UMD build directly from unpkg
. This will result in three global variables: BrowserFS
, fs
, and git
.
<script src="https://unpkg.com/browserfs"></script>
<script>
BrowserFS.configure({ fs: "IndexedDB", options: {} }, function (err) {
if (err) return console.log(err);
window.fs = BrowserFS.BFSRequire("fs");
});
</script>
<script src="https://unpkg.com/isomorphic-git"></script>
You can install it from npm.
npm install --save isomorphic-git
In the package.json you'll see there are actually 4 different versions:
"main": "dist/for-node/",
"browser": "dist/for-browserify/",
"module": "dist/for-future/",
"unpkg": "dist/bundle.umd.min.js",
This probably deserves a brief explanation.
For more details about each build see ./dist/README.md
isogit
CLIIsomorphic-git comes with a simple CLI tool, named isogit
because isomorphic-git
is a lot to type. It is really just a thin shell that translates command line arguments into the equivalent JS API commands. So you should be able to run any current or future isomorphic-git commands using the CLI.
It always starts with an implicit git('.')
so it defaults to working in the
current working directory. (Note I may change that soon, now that I have a findRoot
function. I may change the default to git(git().findRoot(process.cwd()))
.)
git()
APII may continue to make small changes to the API until the 1.0 release, after which I promise not to make any breaking changes.
Setting the working directory and git directory
For regular repositories (with a .git
directory inside them) you simply pass the directory as the initial argument to git()
.
In this case, the git directory is set implicitly to path.join(workdir, '.git')
.
However, if you are working with bare repositories, that assumption is wrong. In this case, you can use the second version to specify the directories explicitly.
// JS example
import git from 'isomorphic-git'
git('./path/to/repo')
// second way
git()
.gitdir('my-bare-repo')
.workdir('/var/www/website')
# CLI example
cd ./path/to/repo
isogit
# second way
isogit --gitdir=my-bare-repo --workdir=/var/www/website
// Complete API
git(workdir)
// second way
git()
.gitdir(gitdir)
.workdir(workdir)
workdir
- The path to the working directory.The working directory is where your files are checked out. Usually this is the parent directory of ".git" but it doesn't have to be.
gitdir
- The path to the git directory.The git directory is where your git repository history is stored. Usually this is a directory called ".git" inside your working directory.
Initialize a new repository
// JS example
import git from 'isomorphic-git'
git('.').init()
# CLI example
isogit init
// Complete API
git()
.gitdir(gitdir)
.init()
gitdir
- The path to the git directory.Promise<void>
Clone a repository
// JS example
import git from 'isomorphic-git'
git('.')
.depth(1)
.clone('https://cors-buster-jfpactjnem.now.sh/github.com/wmhilton/isomorphic-git')
# CLI example
isogit --depth=1 clone https://github.com/wmhilton/isomorphic-git
// Complete API
git()
.workdir(workdir)
.gitdir(gitdir)
.branch(ref)
.auth(authUsername, authPassword)
.remote(remote)
.depth(depth)
.since(since)
.exclude(exclude)
.relative(relative)
.clone(url)
workdir
- The path to the working directory.gitdir
- The path to the git directory.ref=undefined
] - Which branch to clone. By default this is the designated "main branch" of the repository.authUsername=undefined
] - The username to use with Basic AuthauthPassword=undefined
] - The password to use with Basic Authremote='origin'
] - What to name the remote that is created. The default is 'origin'.url
- The URL of the remote repository.depth=undefined
] - Determines how much of the git repository's history to retrieve.since=undefined
] - Only fetch commits created after the given date. Mutually exclusive with depth
.exclude=[]
] - A list of branches or tags. Instructs the remote server not to send us any commits reachable from these refs.relative=false
] - Changes the meaning of depth
to be measured from the current shallow depth rather than from the branch tip.Promise<void>
Fetch commits
// JS example
import git from 'isomorphic-git'
git('.')
.remote('origin')
.depth(1)
.fetch('master')
# CLI example
isogit --remote=origin --depth=1 fetch master
// Complete API
git()
.gitdir(gitdir)
.auth(authUsername, authPassword)
.url(url)
.remote(remote)
.depth(depth)
.since(since)
.exclude(exclude)
.relative(relative)
.fetch(ref)
gitdir
- The path to the git directory.ref=undefined
] - Which branch to fetch from. By default this is the currently checked out branch.authUsername=undefined
] - The username to use with Basic AuthauthPassword=undefined
] - The password to use with Basic Authurl=undefined
] - The URL of the remote git server. The default is the value set in the git config for that remote.remote='origin'
] - If URL is not specified, determines which remote to use.depth=undefined
] - Determines how much of the git repository's history to retrieve.since=undefined
] - Only fetch commits created after the given date. Mutually exclusive with depth
.exclude=[]
] - A list of branches or tags. Instructs the remote server not to send us any commits reachable from these refs.relative=false
] - Changes the meaning of depth
to be measured from the current shallow depth rather than from the branch tip.Promise<void>
Checkout a branch
// JS example
import git from 'isomorphic-git'
git('.')
.checkout('master')
# CLI example
isogit checkout master
// Complete API
git()
.workdir(workdir)
.gitdir(gitdir)
.remote(remote)
.checkout(ref)
workdir
- The path to the working directory.gitdir
- The path to the git directory.ref=undefined
] - Which branch to clone. By default this is the designated "main branch" of the repository.remote='origin'
] - What to name the remote that is created. The default is 'origin'.Promise<void>
List all the tracked files in a repo
// JS example
import git from 'isomorphic-git'
git('.')
.list()
# CLI example
isogit list
// Complete API
git()
.gitdir(gitdir)
.list()
gitdir
- The path to the git directory.Promise<string[]>
- A list of file paths.Add files to the git index (aka staging area)
// JS example
import git from 'isomorphic-git'
git('.')
.add('README.md')
# CLI example
isogit add README.md
// Complete API
git()
.workdir(workdir)
.gitdir(gitdir)
.add(filepath)
workdir
- The path to the working directory.gitdir
- The path to the git directory.filepath
- The path to the file to add to the index.Promise<void>
Remove files from the git index (aka staging area)
// JS example
import git from 'isomorphic-git'
git('.')
.remove('README.md')
# CLI example
isogit remove README.md
// Complete API
git()
.gitdir(gitdir)
.remove(filepath)
gitdir
- The path to the git directory.filepath
- The path to the file to add to the index.Promise<void>
Create a new commit
// JS example
import git from 'isomorphic-git'
git('.')
.author('Mr. Test')
.email('mrtest@example.com')
.signingKey('-----BEGIN PGP PRIVATE KEY BLOCK-----...')
.commit('Added the a.txt file')
# CLI example
isogit --author='Mr. Test' \
--email=mrtest@example.com \
--signingKey="$(cat private.key)" \
commit 'Added the a.txt file'
// Complete API
git()
.gitdir(gitdir)
.author(author.name)
.email(author.email)
.timestamp(author.timestamp)
.datetime(author.date)
.signingKey(privateKeys)
.commit(message)
gitdir
- The path to the git directory.author
- The details about the commit author.author.name=undefined
] - Default is user.name
config.author.email=undefined
] - Default is user.email
config.author.date=new Date()
] - Set the author timestamp field. Default is the current date.author.timestamp=undefined
] - Set the author timestamp field. This is an alternative to using date
using an integer number of seconds since the Unix epoch instead of a JavaScript date object.committer=author
] - The details about the commit author. If not specified, the author details are used.message
- The commit message to use.privateKeys=undefined
] - A PGP private key in ASCII armor format.Promise<void>
Push a branch
// JS example
import git from 'isomorphic-git'
git('.')
.auth(process.env.GITHUB_TOKEN)
.remote('origin')
.push('master')
# CLI example
isogit --auth="$GITHUB_TOKEN" --remote=origin push master
// Complete API
git()
.gitdir(gitdir)
.depth(depth)
.remote(remote)
.url(url)
.auth(authUsername, authPassword)
.push(ref)
gitdir
- The path to the git directory.depth=0
] - Determines how much of the git repository's history to retrieve. If not specified it defaults to 0 which means the entire repo history.ref=undefined
] - Which branch to push. By default this is the currently checked out branch of the repository.authUsername=undefined
] - The username to use with Basic AuthauthPassword=undefined
] - The password to use with Basic Authurl=undefined
] - The URL of the remote git server. The default is the value set in the git config for that remote.remote='origin'
] - If URL is not specified, determines which remote to use.Promise<void>
Find the root git directory
// JS example
import git from 'isomorphic-git'
git()
.findRoot('/path/to/some/gitrepo/path/to/some/file.txt')
// returns '/path/to/some/gitrepo'
# CLI example
isogit findRoot /path/to/some/gitrepo/path/to/some/file.txt
# prints /path/to/some/gitrepo
// Complete API
git()
.findRoot(dir)
dir
- Starting at directory {dir}, walk upwards until you find a directory that contains a '.git' directory.Promise<rootdir>
that directory, which is presumably the root directory of the git repository containing {dir}.List all local branches
// JS example
import git from 'isomorphic-git'
git('.').listBranches()
# CLI example
isogit listBranches
// Complete API
git()
.gitdir(gitdir)
.listBranches()
gitdir
- The path to the git directory.Promise<branches[]>
an array of branch names.Reading from git config
// JS example
import git from 'isomorphic-git'
git('.').config('user.name')
// 'Mr. Test'
# CLI example
isogit config user.name
# Mr. Test
// Complete API
git()
.gitdir(gitdir)
.config(path)
gitdir
- The path to the git directory.path
- The key of the git config entry.Promise<value>
- the config valueWriting to git config
// JS example
import git from 'isomorphic-git'
git('.').config('user.name', 'Mr. Test')
# CLI example
isogit config user.name 'Mr. Test'
// Complete API
git()
.gitdir(gitdir)
.config(path, value)
gitdir
- The path to the git directory.path
- The key of the git config entry.value
- A value to store at that path.Promise<void>
Authentication is normally required for pushing to a git repository. It may also be required to clone or fetch from a private repository. Git does all its authentication using HTTPS Basic Authentication. Usually this is straightforward, but there are some things to watch out for.
If you have two-factor authentication (2FA) enabled on your account, you probably cannot push or pull using your regular username and password. Instead, you may have to create a Personal Access Token (or an App Password in Bitbucket lingo) and use that to authenticate.
// This works for basic username / password auth, or the newer username / token auth
// that is often required if 2FA is enabled.
git('.').auth('username', 'password')
// a one-argument version is also supported
git('.').auth('username:password')
// Personal Access Token Authentication
// (note Bitbucket calls theirs App Passwords instead for some reason)
git('.').auth('username', 'personal access token')
git('.').auth('username', 'app password')
git('.').auth('personal access token') // Github (only) lets you leave out the username
If you are using OAuth2 for token-based authentication, then the form
that the Basic Auth headers take is slightly different. To help with
those cases, there is an oauth2()
method that is available as an
alternative to the auth()
method.
// OAuth2 Token Authentication
// This for is for *actual* OAuth2 tokens (not "personal access tokens").
// Unfortunately, all the major git hosting companies have chosen different conventions!
// Lucky for you, I already looked up and codified it for you.
//
// - oauth2('github', token) - Github uses `token` as the username, and 'x-oauth-basic' as the password.
// - oauth2('bitbucket', token) - Bitbucket uses 'x-token-auth' as the username, and `token` as the password.
// - oauth2('gitlab', token) - Gitlab uses 'oauth2' as the username, and `token` as the password.
//
// I will gladly accept pull requests for more companies' conventions.
git('.').oauth2('github', 'token')
git('.').oauth2('gitlab', 'token')
git('.').oauth2('bitbucket', 'token')
// JS example
import git from 'isomorphic-git'
console.log(git().version())
version
- the version string from package.jsonThe high-level makes some assumptions (like you have a file-system and network access) that might not be well suited to your embedded git-based concept thingy. Fear not! I have written this library as a series of layers that should tree-shake very well:
import * as managers from 'isomorphic-git/dist/for-node/commands'
Each command is available as its own file, so hopefully with a bit of finagling you will be able to import individual commands if you only need a few and are willint to sacrifice the fluent API in order to optimize your bundle size.
import * as managers from 'isomorphic-git/dist/for-node/managers'
Managers are a level above models. They take care of implementation performance details like
import * as models from 'isomorphic-git/dist/for-node/models'
Models are the lowest level building blocks.
They generally have very few or no dependencies except for 'buffer'
.
This makes them portable to many different environments so they can be a useful lowest common denominator.
import * as utils from 'isomorphic-git/dist/for-node/utils'
I lied. Utils are actually the lowest level building blocks.
isomorphic-git
?Isomorphic-git would not have been possible without the pioneering work by @creationix and @chrisdickinson. Git is a tricky binary mess, and without their examples (and their modules!) I would not have been able to come even close to finishing this. They are geniuses ahead of their time.
This work is released under The Unlicense
FAQs
A pure JavaScript reimplementation of git for node and browsers
The npm package isomorphic-git receives a total of 271,393 weekly downloads. As such, isomorphic-git popularity was classified as popular.
We found that isomorphic-git demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.
Security News
Research
Socket's threat research team has detected five malicious npm packages targeting Roblox developers, deploying malware to steal credentials and personal data.