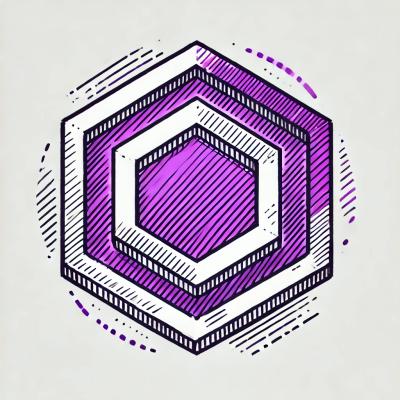
Security News
ESLint is Now Language-Agnostic: Linting JSON, Markdown, and Beyond
ESLint has added JSON and Markdown linting support with new officially-supported plugins, expanding its versatility beyond JavaScript.
isomorphic-webcrypto
Advanced tools
The isomorphic-webcrypto npm package provides a unified API for cryptographic operations that work seamlessly in both Node.js and browser environments. It leverages the Web Cryptography API to offer a consistent interface for tasks such as encryption, decryption, hashing, and key generation.
Encryption and Decryption
This feature allows you to perform encryption and decryption using the AES-GCM algorithm. The code sample demonstrates generating a key, encrypting a message, and then decrypting it back to its original form.
const crypto = require('isomorphic-webcrypto');
async function encryptDecrypt() {
const key = await crypto.subtle.generateKey({
name: 'AES-GCM',
length: 256
}, true, ['encrypt', 'decrypt']);
const data = new TextEncoder().encode('Hello, World!');
const iv = crypto.getRandomValues(new Uint8Array(12));
const encrypted = await crypto.subtle.encrypt({
name: 'AES-GCM',
iv: iv
}, key, data);
const decrypted = await crypto.subtle.decrypt({
name: 'AES-GCM',
iv: iv
}, key, encrypted);
console.log(new TextDecoder().decode(decrypted)); // 'Hello, World!'
}
encryptDecrypt();
Hashing
This feature allows you to create a hash of data using the SHA-256 algorithm. The code sample demonstrates hashing a string and converting the result to a hexadecimal string.
const crypto = require('isomorphic-webcrypto');
async function hashData() {
const data = new TextEncoder().encode('Hello, World!');
const hash = await crypto.subtle.digest('SHA-256', data);
console.log(Buffer.from(hash).toString('hex'));
}
hashData();
Key Generation
This feature allows you to generate a pair of RSA keys for signing and verification. The code sample demonstrates generating an RSA key pair with specific parameters.
const crypto = require('isomorphic-webcrypto');
async function generateKeyPair() {
const keyPair = await crypto.subtle.generateKey({
name: 'RSA-PSS',
modulusLength: 2048,
publicExponent: new Uint8Array([1, 0, 1]),
hash: 'SHA-256'
}, true, ['sign', 'verify']);
console.log(keyPair);
}
generateKeyPair();
The 'crypto' module is a built-in Node.js module that provides cryptographic functionality. It offers a wide range of cryptographic operations, including hashing, encryption, and key generation. Unlike isomorphic-webcrypto, it is not designed to work in browser environments.
The 'node-webcrypto-ossl' package is a Web Cryptography API implementation for Node.js using OpenSSL. It provides a similar API to isomorphic-webcrypto but is specifically tailored for Node.js environments and does not support browsers.
The 'webcrypto-liner' package is a polyfill for the Web Cryptography API, designed to work in environments where the native Web Crypto API is not available. It aims to provide a consistent API across different environments, similar to isomorphic-webcrypto.
webcrypto library for Node, React Native and IE11+
There's a great Node polyfill for the Web Crypto API, but it's not isomorphic yet. This fills the gap until it is.
IE11 and versions of Safari < 11 use an older version of the spec, so the browser implementation includes a webcrypto-shim to iron out the differences. You'll still need to provide your own Promise polyfill.
There's currently no native crypto support in React Native, so the Microsoft Research library is exposed.
npm install isomorphic-webcrypto
There's a simple example below, but there are many more here.
const crypto = require('isomorphic-webcrypto')
// or
import crypto from 'isomorphic-webcrypto'
crypto.subtle.digest(
{ name: 'SHA-256' },
new Uint8Array([1,2,3]).buffer
)
.then(hash => {
// do something with the hash buffer
})
If you need to support other environments (like older browsers), use the Microsoft Research library.
const crypto = require('msrcrypto')
/**
* IMPORTANT: On platforms without crypto, the
* js-only implementation needs another source
* of entropy for operations that require
* random numbers (creating keys, encrypting,
* wrapping keys) This should NOT be Math.random()
*/
crypto.initPrng(randomArrayOf48Bytes)
React Native support is implemented using the Microsoft Research library. The React Native environment only supports Math.random()
, so react-native-securerandom is used to provide proper entropy. This is handled automatically, except for crypto.getRandomValues()
, which requires you wait:
const crypto = require('isomorphic-webcrypto')
crypto.generateKey() // safe (all other methods are safe)
crypto.getRandomValues() // insecure!!!
// Only needed for crypto.getRandomValues
crypto.ensureSecure(err => {
if (err) throw err
crypto.getRandomValues() // safe
// Only wait once, future calls are secure
// No need to wrap every getRandomValues call
})
You should use the webcrypto-shim library directly:
<!-- Any Promise polyfill will do -->
<script src="https://unpkg.com/bluebird"></script>
<script src="https://unpkg.com/webcrypto-shim"></script>
MIT
FAQs
webcrypto library for Node, React Native and IE11+
The npm package isomorphic-webcrypto receives a total of 98,456 weekly downloads. As such, isomorphic-webcrypto popularity was classified as popular.
We found that isomorphic-webcrypto demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
ESLint has added JSON and Markdown linting support with new officially-supported plugins, expanding its versatility beyond JavaScript.
Security News
Members Hub is conducting large-scale campaigns to artificially boost Discord server metrics, undermining community trust and platform integrity.
Security News
NIST has failed to meet its self-imposed deadline of clearing the NVD's backlog by the end of the fiscal year. Meanwhile, CVE's awaiting analysis have increased by 33% since June.