Comparing version 0.1.0 to 0.2.0
## Changelog | ||
Until this library makes it to a production release of v1.x, **minor versions may contain breaking changes to the API**. After v1.x, semantic versioning will be honored, and breaking changes will only occur under the umbrella of a major version bump. | ||
- **v0.5.0** - API change: options.allowOrigins: string --> option.origins: string[] | ||
- **v0.4.0** - now does *not* require a fully-qualified URL (as base+path), to properly mirror native fetch | ||
@@ -5,0 +6,0 @@ - **v0.3.0** - support for URLSearchParams (or raw object) as payload param for GET requests, and vitest as runner - huge thanks to [@danawoodman](https://github.com/danawoodman)! |
interface CorsOptions { | ||
allowOrigin: string; | ||
origins: string[]; | ||
maxAge: number; | ||
@@ -4,0 +4,0 @@ methods: string[]; |
@@ -1,1 +0,1 @@ | ||
var a=Object.defineProperty;var h=Object.getOwnPropertyDescriptor;var g=Object.getOwnPropertyNames;var u=Object.prototype.hasOwnProperty;var A=(s,e)=>{for(var t in e)a(s,t,{get:e[t],enumerable:!0})},w=(s,e,t,r)=>{if(e&&typeof e=="object"||typeof e=="function")for(let n of g(e))!u.call(s,n)&&n!==t&&a(s,n,{get:()=>e[n],enumerable:!(r=h(e,n))||r.enumerable});return s};var C=s=>w(a({},"__esModule",{value:!0}),s);var O={};A(O,{createCors:()=>p});module.exports=C(O);var p=s=>{let{allowOrigin:e="*",maxAge:t=3600,methods:r=["GET"],headers:n={}}=s,i={"content-type":"application/json","Access-Control-Allow-Origin":e,"Access-Control-Allow-Methods":r.join(", "),"Access-Control-Max-Age":t,...n};return{corsify:o=>{if(o.headers.get("access-control-allow-origin"))return o;let{headers:l,status:c,body:d}=o;return new Response(d,{status:c,headers:{...l,...i}})},preflight:o=>{let l=[...new Set(["OPTIONS",...r])];if(o.headers.get("Origin")!==null&&o.headers.get("Access-Control-Request-Method")!==null&&o.headers.get("Access-Control-Request-Headers")!==null){let c={...i,"Access-Control-Allow-Methods":l.join(", "),"Access-Control-Allow-Headers":o.headers.get("Access-Control-Request-Headers")};return new Response(null,{headers:c})}return new Response(null,{headers:{Allow:l.join(", ")}})}}};0&&(module.exports={createCors}); | ||
var d=Object.defineProperty;var h=Object.getOwnPropertyDescriptor;var u=Object.getOwnPropertyNames;var A=Object.prototype.hasOwnProperty;var p=(s,e)=>{for(var t in e)d(s,t,{get:e[t],enumerable:!0})},w=(s,e,t,r)=>{if(e&&typeof e=="object"||typeof e=="function")for(let n of u(e))!A.call(s,n)&&n!==t&&d(s,n,{get:()=>e[n],enumerable:!(r=h(e,n))||r.enumerable});return s};var O=s=>w(d({},"__esModule",{value:!0}),s);var f={};p(f,{createCors:()=>C});module.exports=O(f);var C=s=>{let{origins:e=["*"],maxAge:t=3600,methods:r=["GET"],headers:n={}}=s,l,g={"content-type":"application/json","Access-Control-Allow-Methods":r.join(", "),"Access-Control-Max-Age":t,...n};return{corsify:o=>{let{headers:i,status:c,body:a}=o;return i.get("access-control-allow-origin")||[301,302,308].includes(c)?o:(console.log("corsifying response",{allowOrigin:l}),new Response(a,{status:c,headers:{...i,...g,...l}}))},preflight:o=>{let i=[...new Set(["OPTIONS",...r])],c=o.headers.get("origin");if(l=(e.includes(c)||e.includes("*"))&&{"Access-Control-Allow-Origin":c},console.log("OPTIONS request, setting allowOrigin to",l),o.method==="OPTIONS"){if(o.headers.get("Origin")!==null&&o.headers.get("Access-Control-Request-Method")!==null&&o.headers.get("Access-Control-Request-Headers")!==null){let a={...g,"Access-Control-Allow-Methods":i.join(", "),"Access-Control-Allow-Headers":o.headers.get("Access-Control-Request-Headers"),...l};return new Response(null,{headers:a})}return new Response(null,{headers:{Allow:i.join(", ")}})}}}};0&&(module.exports={createCors}); |
{ | ||
"name": "itty-cors", | ||
"version": "0.1.0", | ||
"version": "0.2.0", | ||
"description": "Simple (and tiny) CORS-handling for any itty-router API. Designed on Cloudflare Workers, but works anywhere.", | ||
@@ -5,0 +5,0 @@ "sourceType": "module", |
@@ -21,2 +21,13 @@ # 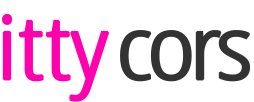 | ||
## !! Breaking API Changes (pre v1.x)!! | ||
You're using an early-access package. Until itty-cors hits a stable v1.0 release, API changes will be broadcasted as minor version bumps. | ||
- **v0.5.0** - `allowOrigins: string` has been replaced with `origins: string[]` to accept an array of origins, and multi-origin support is now correctly implemented. | ||
```js | ||
// previously | ||
const { preflight, corsify } = createCors({ allowOrigin: '*' }) | ||
// now | ||
const { preflight, corsify } = createCors({ origins: ['*'] }) | ||
``` | ||
## Simple Usage | ||
@@ -44,8 +55,8 @@ ```js | ||
.handle(...args) | ||
.then(corsify) // inject CORS headers in all responses | ||
.catch(err => error(500, err.stack)), | ||
.catch(err => error(500, err.stack)) | ||
.then(corsify) // cors should be applied to error responses as well | ||
} | ||
``` | ||
## Per-route Usage (with available options) | ||
## CORS enabled on a single route (and advanced options) | ||
```js | ||
@@ -59,3 +70,3 @@ import { Router } from 'itty-router' | ||
methods: ['GET', 'POST', 'DELETE'], // GET is included by default... omit this if only using GET | ||
allowOrigin: '*', // defaults to allow all (most common). Restrict if needed. | ||
origins: ['*'], // defaults to allow all (most common). Restrict if needed. | ||
maxAge: 3600, | ||
@@ -80,3 +91,3 @@ headers: { | ||
.handle(...args) | ||
.catch(err => error(500, err.stack)), | ||
.catch(err => error(500, err.stack)) | ||
} | ||
@@ -92,3 +103,3 @@ ``` | ||
| --- | --- | --- | --- | | ||
| **allowOrigin** | `string` | `'*'` | By default, all origins are allowed (most common). Modify this to restrict to specific origins. | ||
| **origins** | `string[]` | `['*']` | By default, all origins are allowed (most common). Modify this to restrict to specific origins. | ||
| **maxAge** | `number` | `3600` | Set the expiry of responses | ||
@@ -95,0 +106,0 @@ | **methods** | `string[]` | `['GET']` | Define which methods are allowed. `OPTIONS` will be automatically added. |
Sorry, the diff of this file is not supported yet
15499
59
112