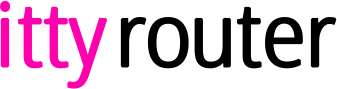

It's an itty bitty router. Like... super tiny, with zero dependencies. For reals.
Did we mention it supports route/query params like in Express.js?
Installation
yarn add itty-router
or if you've been transported back to 2017...
npm install itty-router
Features
Examples
Kitchen Sink
import { Router } from 'itty-router'
const router = Router()
router.get('/todos/:id', console.log)
router
.get('/todos/oops', () => console.log('you will never see this, thanks to upstream /todos/:id'))
.get('/features/chainable-route-declaration', () => console.log('yep!'))
.get('/features/:optionals?', () => console.log('check!'))
router.post('/todos', () => console.log('posted a todo'))
router.future('/todos', () => console.log(`this caught using the FUTURE method!`))
router.handle({ method: 'GET', url: 'https://foo.com/todos/13?foo=bar' })
Usage
1. Create a Router
import { Router } from 'itty-router'
const router = Router()
2. Register Route(s)
.{methodName}(route:string, handler1:function, handler2:function, ...)
The "instantiated" router translates any attribute (e.g. .get
, .post
, .patch
, .whatever
) as a function that binds a "route" (string) to route handlers (functions) on that method type (e.g. router.get --> GET
, router.post --> POST
). When the url fed to .handle({ url })
matches the route and method, the handlers are fired sequentially. Each is given the original request/context, with any parsed route/query params injected as well. The first handler that returns (anything) will end the chain, allowing early exists from errors, inauthenticated requests, etc. This mechanism allows ANY method to be handled, including completely custom methods (we're very curious how creative individuals will abuse this flexibility!). The only "method" currently off-limits is handle
, as that's used for route handling (see below).
router.get('/todos/:user/:item?', (req) => {
let { params, query, url } = req
let { user, item } = params
console.log('GET TODOS from', url, { user, item })
})
3. Handle Incoming Request(s)
.handle(request = { method:string = 'GET', url:string })
The only requirement for the .handle(request)
method is an object with a valid full url (e.g. https://example.com/foo
). The method
property is optional and defaults to GET
(which maps to routes registered with router.get()
). This method will return the first route handler that actually returns something. For async/middleware examples, please see below.
router.handle({
method: 'GET',
url: 'https://example.com/todos/jane/13',
})
Examples
Within a Cloudflare Function
import { Router } from 'itty-router'
const router = Router()
router
.get('/foo', () => new Response('Foo Index!'))
.get('/foo/:id', ({ params }) => new Response(`Details for item ${params.id}.`))
.get('*', () => new Response('Not Found.', { status: 404 })
addEventListener('fetch', event => event.respondWith(router.handle(event.request)))
Multiple Route Handlers as Middleware
import { Router } from 'itty-router'
const router = Router()
const withUser = (req) => {
req.user = { name: 'Mittens', age: 3 }
}
const requireUser = (req) => {
if (!req.user) return new Response('Not Authenticated', { status: 401 })
}
const showUser = (req) => new Response(JSON.stringify(req.user))
router
.get('/pass/user', withUser, requireUser, showUser)
.get('/fail/user', requireUser, showUser)
router.handle({ url: 'https://example.com/pass/user' })
router.handle({ url: 'https://example.com/fail/user' })
Testing & Contributing
- fork repo
- add code
- run tests (and add your own)
yarn test
- submit PR
- profit
Entire Router Code (latest...)
const Router = () =>
new Proxy({}, {
get: (o, k) => k === 'handle'
? async (q) => {
for ([p, hs] of o[(q.method || 'GET').toLowerCase()] || []) {
if (m = (u = new URL(q.url)).pathname.match(p)) {
q.params = m.groups
q.query = Object.fromEntries(u.searchParams.entries())
for (h of hs) {
if ((s = await h(q)) !== undefined) return s
}
}
}
}
: (p, ...hs) => (o[k] = o[k] || []).push([
`^${p
.replace('*', '.*')
.replace(/(\/:([^\/\?]+)(\?)?)/gi, '/$3(?<$2>[^/]+)$3')}$`,
hs
]) && o
})
Special Thanks
This repo goes out to my past and present colleagues at Arundo - who have brought me such inspiration, fun,
and drive over the last couple years. In particular, the absurd brevity of this code is thanks to a
clever [abuse] of Proxy
, courtesy of the brilliant @mvasigh.
This trick allows methods (e.g. "get", "post") to by defined dynamically by the router as they are requested,
drastically reducing boilerplate.
Changelog
Until this library makes it to a production release of v1.x, minor versions may contain breaking changes to the API. After v1.x, semantic versioning will be honored, and breaking changes will only occur under the umbrella of a major version bump.
- v0.9.0 - added support for multiple handlers (middleware)
- v0.8.0 - deep minification pass and build steps for final module
- v0.7.0 - removed { path } from request handler context, travis build fixed, added coveralls, improved README docs
- v0.6.0 - added types to project for vscode intellisense (thanks @mvasigh)
- v0.5.4 - fix: wildcard routes properly supported