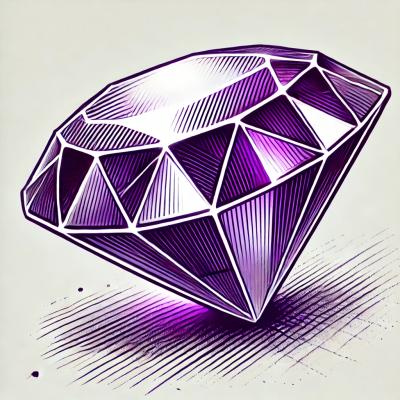
Security News
RubyGems.org Adds New Maintainer Role
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
itty-router
Advanced tools
It's an itty bitty router, designed for Express.js-like routing within Cloudflare Workers (or any ServiceWorker). Like... it's super tiny, with zero dependencies. For reals.
yarn add itty-router
import { Router } from 'itty-router'
// create a router
const router = Router() // this is a Proxy, not a class
// GET index
router.get('/foo', () => new Response('Foo Index!'))
// GET item
router.get('/foo/:id.:format?', request => {
const { id, format = 'csv' } = request.params
return new Response(`Getting item ${id} in ${format} format.`)
}
// 404/Missing as final catch-all route
router.all('*', () => new Response('Not Found.', { status: 404 }))
// attach the router handle to the event handler
addEventListener('fetch', event =>
event.respondWith(router.handle(event.request))
)
/api/:foo/:id?.:format?
)?page=3&foo-bar
){ params: { foo: 'bar' }, query: { page: '3' }}
Router(options = {})
Name | Type(s) | Description | Examples |
---|---|---|---|
base | string | prefixes all routes with this string | Router({ base: '/api' }) |
import { Router } from 'itty-router'
const router = Router() // no "new", as this is not a real ES6 class/constructor!
.{methodName}(route:string, handler1:function, handler2:function, ...)
The "instantiated" router translates any attribute (e.g. .get
, .post
, .patch
, .whatever
) as a function that binds a "route" (string) to route handlers (functions) on that method type (e.g. router.get --> GET
, router.post --> POST
). When the url fed to .handle({ url })
matches the route and method, the handlers are fired sequentially. Each is given the original request/context, with any parsed route/query params injected as well. The first handler that returns (anything) will end the chain, allowing early exists from errors, inauthenticated requests, etc. This mechanism allows ANY method to be handled, including completely custom methods (we're very curious how creative individuals will abuse this flexibility!). The only "method" currently off-limits is handle
, as that's used for route handling (see below).
Special Exception - the "all" channel: Any routes on the "all" channel will match to ANY method (e.g. GET/POST/whatever), allowing for greater middleware support, nested routers, 404 catches, etc.
// register a route on the "GET" method
router.get('/todos/:user/:item?', (req) => {
let { params, query, url } = req
let { user, item } = params
console.log('GET TODOS from', url, { user, item })
})
.handle(request = { method:string = 'GET', url:string })
The only requirement for the .handle(request)
method is an object with a valid full url (e.g. https://example.com/foo
). The method
property is optional and defaults to GET
(which maps to routes registered with router.get()
). This method will return the first route handler that actually returns something. For async/middleware examples, please see below.
router.handle({
method: 'GET', // optional, default = 'GET'
url: 'https://example.com/todos/jane/13', // required
})
// matched handler from step #2 (above) will execute, with the following output:
// GET TODOS from https://example.com/todos/jane/13 { user: 'jane', item: '13' }
// lets save a missing handler
const missingHandler = new Response('That resource was not found.', { status: 404 })
// create a parent router
const parentRouter = Router()
// and a child router
const todosRouter = Router({ base: '/todos' })
// with some routes on it...
todosRouter
.get('/', () => new Response('Todos Index'))
.get('/:id', ({ params }) => new Response(`Todo #${params.id}`))
// then divert ALL requests to /todos/* into the child router
parentRouter
.all('/todos/*', todosRouter.handle) // all /todos/* routes will route through the todosRouter
.all('*', missingHandler)
// GET /todos --> Todos Index
// GET /todos/13 --> Todo #13
// POST /todos --> missingHandler (it will fail to catch inside todosRouter, and be caught by final "all" on parentRouter)
// GET /foo --> missingHandler
// withUser modifies original request, then continues without returning
const withUser = (req) => {
req.user = { name: 'Mittens', age: 3 }
}
// requireUser optionally returns (early) if user not found on request
const requireUser = (req) => {
if (!req.user) return new Response('Not Authenticated', { status: 401 })
}
// showUser returns a response with the user, as it is assumed to exist at this point
const showUser = (req) => new Response(JSON.stringify(req.user))
router.get('/pass/user', withUser, requireUser, showUser) // withUser injects user, allowing requireUser to not return/continue
router.get('/fail/user', requireUser, showUser) // requireUser returns early because req.user doesn't exist
router.handle({ url: 'https://example.com/pass/user' }) // --> STATUS 200: { name: 'Mittens', age: 3 }
router.handle({ url: 'https://example.com/fail/user' }) // --> STATUS 401: Not Authenticated
// withUser modifies original request, then continues without returning
const withUser = (req) => {
req.user = { name: 'Mittens', age: 3 }
}
router.get('*', withUser) // embeds user before all other matching routes
router.get('/user', (req) => new Response(JSON.stringify(req.user))) // user embedded already!
router.handle({ url: 'https://example.com/user' }) // --> STATUS 200: { name: 'Mittens', age: 3 }
yarn dev
yarn verify
const Router = (o = {}) =>
new Proxy(o, {
get: (t, k, c) => k === 'handle'
? async (r, ...args) => {
for ([, p, hs] of t.r.filter(r => r[0] === r.method || 'ALL')) {
if (m = (u = new URL(r.url)).pathname.match(p)) {
r.params = m.groups
r.query = Object.fromEntries(u.searchParams.entries())
for (h of hs) {
if ((s = await h(r, ...args)) !== undefined) return s
}
}
}
}
: (p, ...hs) =>
(t.r = t.r || []).push([
k.toUpperCase(),
`^${(t.base || '')+p
.replace(/(\/?)\*/g, '($1.*)?')
.replace(/\/$/, '')
.replace(/:([^\/\?\.]+)(\?)?/g, '$2(?<$1>[^/\.]+)$2')
}\/*$`,
hs
]) && c
})
This repo goes out to my past and present colleagues at Arundo - who have brought me such inspiration, fun,
and drive over the last couple years. In particular, the absurd brevity of this code is thanks to a
clever [abuse] of Proxy
, courtesy of the brilliant @mvasigh.
This trick allows methods (e.g. "get", "post") to by defined dynamically by the router as they are requested,
drastically reducing boilerplate.
These folks are the real heroes, making open source the powerhouse that it is! Help us out and add your name to this list!
FAQs
A tiny, zero-dependency router, designed to make beautiful APIs in any environment.
The npm package itty-router receives a total of 42,161 weekly downloads. As such, itty-router popularity was classified as popular.
We found that itty-router demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.
Security News
Research
Socket's threat research team has detected five malicious npm packages targeting Roblox developers, deploying malware to steal credentials and personal data.