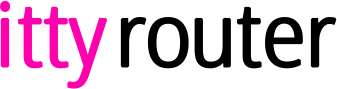

It's an itty bitty router, designed for Express.js-like routing within Cloudflare Workers (or any ServiceWorker). Like... it's super tiny, with zero dependencies. For reals.
Installation
npm install itty-router
Simple Example
import { Router } from 'itty-router'
const router = Router()
router.get('/foo', () => new Response('Foo Index!'))
router.get('/foo/:id.:format?', request => {
const { id, format = 'csv' } = request.params
return new Response(`Getting item ${id} in ${format} format.`)
})
router.all('*', () => new Response('Not Found.', { status: 404 }))
addEventListener('fetch', event =>
event.respondWith(router.handle(event.request))
)
Features
Options API
Router(options = {})
Name | Type(s) | Description | Examples |
---|
base | string | prefixes all routes with this string | Router({ base: '/api' }) |
Usage
1. Create a Router
import { Router } from 'itty-router'
const router = Router()
2. Register Route(s)
.{methodName}(route:string, handler1:function, handler2:function, ...)
The "instantiated" router translates any attribute (e.g. .get
, .post
, .patch
, .whatever
) as a function that binds a "route" (string) to route handlers (functions) on that method type (e.g. router.get --> GET
, router.post --> POST
). When the url fed to .handle({ url })
matches the route and method, the handlers are fired sequentially. Each is given the original request/context, with any parsed route/query params injected as well. The first handler that returns (anything) will end the chain, allowing early exists from errors, inauthenticated requests, etc. This mechanism allows ANY method to be handled, including completely custom methods (we're very curious how creative individuals will abuse this flexibility!). The only "method" currently off-limits is handle
, as that's used for route handling (see below).
Special Exception - the "all" channel: Any routes on the "all" channel will match to ANY method (e.g. GET/POST/whatever), allowing for greater middleware support, nested routers, 404 catches, etc.
router.get('/todos/:user/:item?', (req) => {
let { params, query, url } = req
let { user, item } = params
console.log('GET TODOS from', url, { user, item })
})
3. Handle Incoming Request(s)
.handle(request = { method:string = 'GET', url:string })
The only requirement for the .handle(request)
method is an object with a valid full url (e.g. https://example.com/foo
). The method
property is optional and defaults to GET
(which maps to routes registered with router.get()
). This method will return the first route handler that actually returns something. For async/middleware examples, please see below.
router.handle({
method: 'GET',
url: 'https://example.com/todos/jane/13',
})
Examples
Nested Routers (with 404 handling)
const missingHandler = new Response('That resource was not found.', { status: 404 })
const parentRouter = Router()
const todosRouter = Router({ base: '/todos' })
todosRouter
.get('/', () => new Response('Todos Index'))
.get('/:id', ({ params }) => new Response(`Todo #${params.id}`))
parentRouter
.all('/todos/*', todosRouter.handle)
.all('*', missingHandler)
Middleware
Bonus: Any of these handlers may be awaitable async functions!
const withUser = (req) => {
req.user = { name: 'Mittens', age: 3 }
}
const requireUser = (req) => {
if (!req.user) return new Response('Not Authenticated', { status: 401 })
}
const showUser = (req) => new Response(JSON.stringify(req.user))
router.get('/pass/user', withUser, requireUser, showUser)
router.get('/fail/user', requireUser, showUser)
router.handle({ url: 'https://example.com/pass/user' })
router.handle({ url: 'https://example.com/fail/user' })
Multi-route (Upstream) Middleware
const withUser = (req) => {
req.user = { name: 'Mittens', age: 3 }
}
router.get('*', withUser)
router.get('/user', (req) => new Response(JSON.stringify(req.user)))
router.handle({ url: 'https://example.com/user' })
Testing & Contributing
- fork repo
- add code
- run tests (add your own if needed)
yarn dev
- verify tests run once minified
yarn verify
- commit files (do not manually modify version numbers)
- submit PR
- we'll add you to the credits :)
The Entire Code (for more legibility, see src on GitHub)
const Router = (o = {}) =>
new Proxy(o, {
get: (t, k, c) => k === 'handle'
? async (r, ...a) => {
for ([p, hs] of t.r.filter(i => i[2] === r.method || i[2] === 'ALL')) {
if (m = (u = new URL(r.url)).pathname.match(p)) {
r.params = m.groups
r.query = Object.fromEntries(u.searchParams.entries())
for (h of hs) {
if ((s = await h(r, ...a)) !== undefined) return s
}
}
}
}
: (p, ...hs) =>
(t.r = t.r || []).push([
`^${(t.base || '')+p
.replace(/(\/?)\*/g, '($1.*)?')
.replace(/\/$/, '')
.replace(/:(\w+)(\?)?/g, '$2(?<$1>[^/\.]+)$2')
}\/*$`,
hs,
k.toUpperCase(),
]) && c
})
Special Thanks
This repo goes out to my past and present colleagues at Arundo - who have brought me such inspiration, fun,
and drive over the last couple years. In particular, the absurd brevity of this code is thanks to a
clever [abuse] of Proxy
, courtesy of the brilliant @mvasigh.
This trick allows methods (e.g. "get", "post") to by defined dynamically by the router as they are requested,
drastically reducing boilerplate.
Contributors
These folks are the real heroes, making open source the powerhouse that it is! Help us out and add your name to this list!
Core, Concepts, and Codebase
- @technoyes - three kind-of-a-big-deal errors caught at once. Imagine the look on my face... thanks man!! :)
- @hunterloftis - router.handle() method now accepts extra arguments and passed them to route functions
- @roojay520 - TS interface fixes
- @mvasigh - proxy hacks courtesy of this chap
Documentation Fixes