
Ultra-small (~390 bytes) library for TTL date math and converting ms durations to and from strings.
Features
- Convert string durations to ms/seconds.
- Convert ms to human-readable string durations.
- Add durations to dates.
- Tiny. The entire library is ~390 bytes, and tree-shakeable to be even smaller.
Comparison to other top-rated libraries
1: minified and gzipped
Performance
The only function most folks care about in terms of raw performance is string to ms conversion. In this, itty stacks up pretty well, being significantly faster than ms, but falling to the insanely-optimized @lukeed/ms.
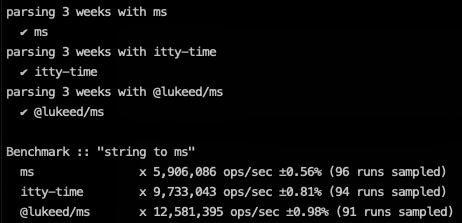
Moral of the story, probably don't use ms.
Use Luke's if you want the absolute fastest parsing, or itty if you want some of the other functions as well. If you're byte-counting, itty wins again, but if you're byte-counting that hard, you're probably better off with raw ms math if you can stomach it.
What does it do?
TTL math is a maintenance nightmare. It's a pain to write, a pain to read, and when you update the math later, you'll probably forget to update the comment, causing all sorts of mayhem. Instead of maintaining math in your code like this:
const TTL = 2 * 7 * 24 * 60 * 60 * 1000
Use a simple converter so you can use plain english time descriptions like this:
const TTL = seconds('2 weeks')
That's it!
Differences between itty-time and ms (the library)
Aside from the smaller size and faster speed, do note that itty-time's ms
and seconds
functions require writing out the actual unit name like "3 hours"
, rather than supporting abbreviations like "3h"
or "3hrs"
. We do this for two reasons:
- This improves readability. We like this.
- Supporting fewer variations keeps itty-time smaller.
API
ms(duration: TimeString) => number

Converts string durations to milliseconds (great for time math).
import { ms } from 'itty-time'
ms('2 weeks')
seconds(duration: TimeString) => number

Convert string durations to seconds (great for expiry systems that use seconds, like Cloudflare KV).
import { seconds } from 'itty-time' ~200 bytes
seconds('2 weeks')
duration(ms: number) => string | Array<TimeParts>

Converts a duration number to a string.
import { duration } from 'itty-time'
duration(3750000)
duration(3750000, { parts: 2 })
duration(3750000, { join: ' --> ' })
duration(3750000, { join: false })
datePlus(duration: TimeString, from = new Date) => Date

Need to add/subtract time from a date? Find the date two weeks from now? Set an alarm for 30 minutes before your birthday? Use this.
import { datePlus } from 'itty-time'
datePlus('2 weeks')
datePlus('-30 minutes', new Date('2024/11/1'))