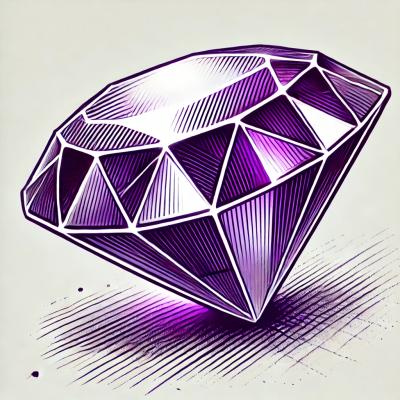
Security News
RubyGems.org Adds New Maintainer Role
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Jade, now known as Pug, is a high-performance template engine heavily influenced by Haml and implemented with JavaScript for Node.js and browsers. It is used to generate HTML dynamically and is known for its clean and minimal syntax.
Template Rendering
Jade allows you to render HTML templates with dynamic data. In this example, a simple template is rendered with a variable 'name' which is replaced with 'World'.
const pug = require('pug');
const html = pug.render('p Hello, #{name}!', { name: 'World' });
console.log(html);
Template Inheritance
Jade supports template inheritance, allowing you to create a base template and extend it in other templates. This example demonstrates how 'index.pug' extends 'layout.pug' and defines a content block.
const pug = require('pug');
const html = pug.renderFile('index.pug');
console.log(html);
// index.pug
// extends layout.pug
// block content
// h1= title
// p Welcome to #{title}
// layout.pug
// doctype html
// html
// head
// title= title
// body
// block content
Mixins
Mixins in Jade allow you to create reusable blocks of code. This example shows a mixin 'list' that generates an unordered list from an array of items.
const pug = require('pug');
const html = pug.renderFile('mixin.pug');
console.log(html);
// mixin.pug
// mixin list(items)
// ul
// each item in items
// li= item
// +list(['Apple', 'Banana', 'Cherry'])
EJS (Embedded JavaScript) is a simple templating language that lets you generate HTML markup with plain JavaScript. It is similar to Jade but uses a more traditional syntax that is closer to HTML, making it easier for those familiar with HTML to use.
Handlebars is a popular templating engine that provides the power necessary to let you build semantic templates effectively with no frustration. It is known for its simplicity and logic-less templates, which makes it different from Jade's more feature-rich approach.
Mustache is a logic-less template syntax. It is named 'logic-less' because it lacks any explicit control flow statements like if and else. This makes it very different from Jade, which supports more complex logic and features.
FAQs
A clean, whitespace-sensitive template language for writing HTML
The npm package jade receives a total of 137,634 weekly downloads. As such, jade popularity was classified as popular.
We found that jade demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.
Security News
Research
Socket's threat research team has detected five malicious npm packages targeting Roblox developers, deploying malware to steal credentials and personal data.