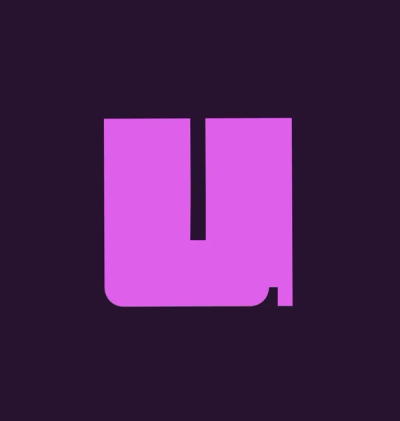
Product
Socket Now Supports uv.lock Files
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
jasmine-auto-spies
Advanced tools
Create automatic spies from classes in jasmine tests, also for promises and observables
2.x
and above requires RxJS 6.0 and above.3.x
and above requires TypeScript 2.8 and above.Creating spies has never been EASIER! 💪👏
If you need to create a spy from any class, just do:
const myServiceSpy = createSpyFromClass(MyService);
THAT'S IT!
If you're using TypeScript, you get EVEN MORE BENEFITS:
const myServiceSpy: Spy<MyService> = createSpyFromClass(MyService);
Now you can autocomplete AND have an auto spy for each method, returning Observable / Promise specific control methods.
✅ Keep you tests DRY - no more repeated spy setup code, no need for separate spy files
✅ Type completion for both the original Class and the spy methods
✅ Automatic return type detection by using a simple decorator
npm install -D jasmine-auto-spies
// my-spec.js
import { createSpyFromClass } from 'jasmine-auto-spies';
import { MyService } from './my-service';
import { MyComponent } from './my-component';
describe('MyComponent', ()=>{
let myServiceSpy;
let componentUnderTest;
beforeEach(()=>{
myServiceSpy = createSpyFromClass(MyService);
componentUnderTest = new MyComponent(myServiceSpy);
});
it('should get data on init', ()=>{
const fakeData = [{fake: 'data'}];
myServiceSpy.getData.and.returnWith(fakeData);
componentUnderTest.init();
expect(myServiceSpy.getData).toHaveBeenCalled();
expect(componentUnderTest.compData).toEqual(fakeData);
});
});
// my-component.js
export class MyComponent{
constructor(myService){
this.myService = myService;
}
init(){
this.compData = this.myService.getData();
}
}
// my-service.js
export class MyService{
getData{
return [
{ ...someRealData... }
]
}
}
Set these 2 properties in your tsconfig.json
-
{
"compilerOptions": {
"experimentalDecorators": true,
"emitDecoratorMetadata": true
}
}
// my-spec.ts
import { Spy, createSpyFromClass } from 'jasmine-auto-spies';
import { MyService } from './my-service';
let myServiceSpy: Spy<MyService>;
beforeEach( ()=> {
myServiceSpy = createSpyFromClass( MyService );
});
it('should Do something' ()=> {
myServiceSpy.getName.and.returnValue('Fake Name');
... (the rest of the test) ...
});
// my-service.ts
class MyService{
getName(): string{
return 'Bonnie';
}
}
Promise
returning methodUse the resolveWith
or rejectWith
methods -
import { Spy, createSpyFromClass } from 'jasmine-auto-spies';
let myServiceSpy: Spy<MyService>;
beforeEach(() => {
myServiceSpy = createSpyFromClass(MyService);
});
it(() => {
myServiceSpy.getItems.and.resolveWith(fakeItemsList);
// OR
myServiceSpy.getItems.and.rejectWith(fakeError);
});
Observable
returning methodUse the nextWith
or throwWith
and other methods -
import { Spy, createSpyFromClass } from 'jasmine-auto-spies';
let myServiceSpy: Spy<MyService>;
beforeEach(() => {
myServiceSpy = createSpyFromClass(MyService);
});
it(() => {
myServiceSpy.getProducts.and.nextWith(fakeProductsList);
// OR
myServiceSpy.getProducts.and.nextOneTimeWith(fakeProductsList); // emits one value and completes
// OR
myServiceSpy.getProducts.and.throwWith(fakeError);
// OR
myServiceSpy.getProducts.and.complete();
});
calledWith()
to configure conditional return valuesYou can setup the expected arguments ahead of time
by using calledWith
like so:
myServiceSpy.getProducts.calledWith(1).returnValue(true);
and it will only return this value if your subject was called with getProducts(1)
.
myServiceSpy.getProductsPromise.calledWith(1).resolveWith(true);
// OR
myServiceSpy.getProducts$.calledWith(1).nextWith(true);
// OR ANY OTHER ASYNC CONFIGURATION METHOD...
throwOnMismatch()
to turn a conditional stub into a mockmyServiceSpy.getProducts
.calledWith(1)
.returnValue(true)
.throwOnMismatch();
is equal to:
myServiceSpy.getProducts.and.returnValue(true);
expect(myServiceSpy.getProducts).toHaveBeenCalledWith(1);
But the difference is that the error is being thrown during getProducts()
call and not in the expect(...)
call.
If you need to manually add methods that you want to be spies by passing an array of names as the second param of the createSpyFromClass
function:
let spy = createSpyFromClass(MyClass, ['customMethod1', 'customMethod2']);
This is good for times where a method is not part of the prototype
of the Class but instead being defined in its constructor.
class MyClass {
constructor() {
this.customMethod1 = function() {
// This definition is not part of MyClass' prototype
};
}
}
FAQs
Create automatic spies from classes in jasmine tests, also for promises and observables
The npm package jasmine-auto-spies receives a total of 9,057 weekly downloads. As such, jasmine-auto-spies popularity was classified as popular.
We found that jasmine-auto-spies demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
Research
Security News
Socket researchers have discovered multiple malicious npm packages targeting Solana private keys, abusing Gmail to exfiltrate the data and drain Solana wallets.
Security News
PEP 770 proposes adding SBOM support to Python packages to improve transparency and catch hidden non-Python dependencies that security tools often miss.