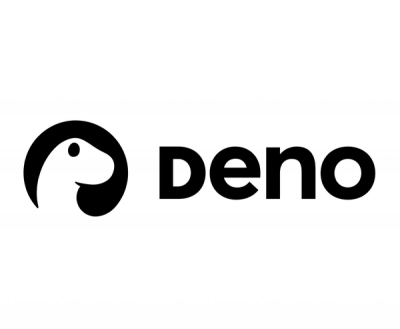
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
jasmine-expect
Advanced tools
Readable tests.
The Jasmine testing framework from Pivotal Labs comes with this default set of matchers;
expect(fn).toThrow(e);
expect(instance).toBe(instance);
expect(mixed).toBeDefined();
expect(mixed).toBeFalsy();
expect(number).toBeGreaterThan(number);
expect(number).toBeLessThan(number);
expect(mixed).toBeNull();
expect(mixed).toBeTruthy();
expect(mixed).toBeUndefined();
expect(array).toContain(member);
expect(string).toContain(substring);
expect(mixed).toEqual(mixed);
expect(mixed).toMatch(pattern);
Using the default Matchers your tests and failure output might look something like this;
it('should expose the expected API', function() {
expect(typeof record.save).toEqual('function');
});
Expected "undefined" to equal "function"
it('should distribute evenly', function() {
expect(basket.items % 2 === 0).toEqual(true);
});
Expected false to equal true
Using Jasmine-Matchers the same tests and failure output can be written like this;
it('should expose the expected API', function() {
expect(record).toHaveMethod('save');
});
Expected member "save" of { save : undefined } to be function.
it('should distribute evenly', function() {
expect(basket).toHaveEvenNumber('items');
});
Expected member "items" of { items : 25 } to be even number.
npm install jasmine-expect --save-dev
bower install jasmine-expect --save-dev
Downloads are available on the releases page.
Include Jasmine Matchers after Jasmine but before your tests.
<script src="/path/to/jasmine-matchers.js"></script>
Integration is easy with the karma-jasmine-matchers plugin.
When using jasmine-node 1.x, provide the path to where Jasmine Matchers is installed as the value for --requireJsSetup
.
jasmine-node --requireJsSetup node_modules/jasmine-expect/index.js test
jasmine-node 2.x has no such hooks that I'm aware of for loading helpers, in this case the following call is needed before the first test in your suite.
require('jasmine-expect');
Jasmine-Matchers-Snippets can be installed with Package Control to ease development with Jasmine Matchers in Sublime Text.
Plugin for Tern to auto-complete matchers in supported editors.
Jasmine Matchers can be used with Facebook's Jest.
// package.json
"unmockedModulePathPatterns": [
"jasmine-expect"
],
// some test
import JasmineExpect from 'jasmine-expect';
// ...
expect(someValue).toBeArrayOfObjects();
Jasmine-Matchers is tested on Travis CI and Sauce Labs against the following environments.
FAQs
Write Beautiful Specs with Custom Matchers
The npm package jasmine-expect receives a total of 30,647 weekly downloads. As such, jasmine-expect popularity was classified as popular.
We found that jasmine-expect demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.