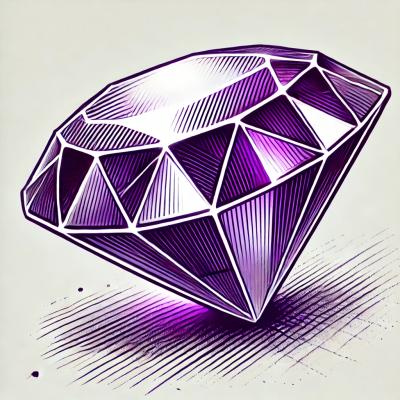
Security News
RubyGems.org Adds New Maintainer Role
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
jest-docblock
Advanced tools
The jest-docblock package is used to parse and manipulate docblocks - comments at the top of a file that are often used to provide metadata about the file, such as descriptions, authors, and licenses. It is commonly used in the Jest testing framework to handle such metadata in test files.
Parsing docblocks
This feature allows you to parse the contents of a file to extract the metadata contained within a docblock. The parse function returns an object where each key is a tag from the docblock.
const { parse } = require('jest-docblock');
const contents = `/**
* This is a docblock
*
* @flow
*/
const foo = 'bar';`;
const docblock = parse(contents);
console.log(docblock);
Extracting docblock
This feature allows you to extract the raw docblock as a string from the contents of a file. This can be useful if you want to manipulate the docblock as a whole or use it for documentation purposes.
const { extract } = require('jest-docblock');
const contents = `/**
* This is a docblock
*
* @flow
*/
const foo = 'bar';`;
const docblockString = extract(contents);
console.log(docblockString);
Printing docblock
This feature allows you to take an object representing a docblock and print it back into the docblock format. This is useful for modifying or adding new metadata to a file's docblock.
const { print } = require('jest-docblock');
const docblock = { flow: '' };
const newContents = print(docblock);
console.log(newContents);
The comment-parser package is similar to jest-docblock in that it is used to parse comments in code. It supports parsing of block comments in various formats and is not tied to any specific testing framework, making it more flexible for different use cases.
Doctrine is another JSDoc parsing library that can extract type information and other metadata from JSDoc comments. It is more focused on the JSDoc standard and provides a detailed AST of the parsed comments, which can be more powerful but also more complex than jest-docblock.
jsdoc-to-markdown is a package that takes JSDoc comments and converts them into markdown documentation. While it is not a direct alternative to jest-docblock, it serves a related purpose by transforming code comments into user-friendly documentation.
FAQs
`jest-docblock` is a package that can extract and parse a specially-formatted comment called a "docblock" at the top of a file.
The npm package jest-docblock receives a total of 11,294,070 weekly downloads. As such, jest-docblock popularity was classified as popular.
We found that jest-docblock demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 6 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.
Security News
Research
Socket's threat research team has detected five malicious npm packages targeting Roblox developers, deploying malware to steal credentials and personal data.