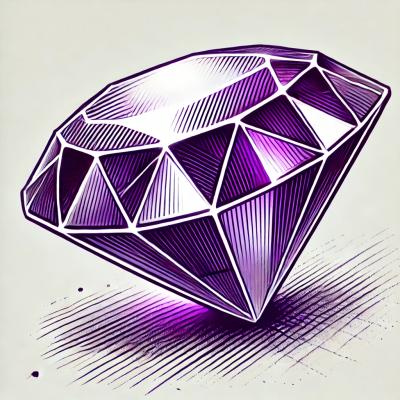
Security News
RubyGems.org Adds New Maintainer Role
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
jrfscheduler
Advanced tools
jrfscheduler is a async/await scheduled task scheduler.
$ npm i jrfscheduler
You can set one of six types of schedules.
The task is performed on the set date once
let schedule = new JrfScheduler();
let now = new Date();
schedule.schedule = {
date: new Date(now.getTime() + 600)
};
The task is performed every time at a specified interval.
the format string: Xms - X milliseconds, Xs - X seconds, Xm - X minutes, Xh - X hours, Xd - X days.
For example: "1d, 2h, 3ms"
let schedule = new JrfScheduler();
schedule.schedule = {
interval: '100ms'
};
The task is performed every day at a specified time.
let schedule = new JrfScheduler();
schedule.schedule = {
times: [
{h: 0, m: 2, s: 33},
{h: 13, m: 12, s: 35},
{h: 22, m: 43, s: 49}
]
};
The task is performed on the specified days of the week at the specified time.
1(mon) - 7(sun)
let schedule = new JrfScheduler();
schedule.schedule = {
daysOfWeek: [
{
dayOfWeek: 1,
times: [
{h: 0, m: 2, s: 33},
{h: 13, m: 12, s: 35},
{h: 22, m: 43, s: 49}
]
},
{
dayOfWeek: 3,
times: [
{h: 0, m: 2, s: 33},
{h: 13, m: 12, s: 35},
{h: 22, m: 43, s: 49}
]
},
{
dayOfWeek: 7,
times: [
{h: 0, m: 2, s: 33},
{h: 13, m: 12, s: 35},
{h: 22, m: 43, s: 49}
]
},
]
};
The task is executed on the specified day of the month at the specified time.
let schedule = new JrfScheduler();
schedule.schedule = {
daysOfMonth: [
{
dayOfMonth: 1,
times: [
{h: 0, m: 2, s: 33},
{h: 13, m: 12, s: 35},
{h: 22, m: 43, s: 49}
]
},
{
dayOfMonth: 12,
times: [
{h: 0, m: 2, s: 33},
{h: 13, m: 12, s: 35},
{h: 22, m: 43, s: 49}
]
},
{
dayOfMonth: 27,
times: [
{h: 0, m: 2, s: 33},
{h: 13, m: 12, s: 35},
{h: 22, m: 43, s: 49}
]
},
]
};
The task is executed on the specified days at the specified time of the specified months.
let schedule = new JrfScheduler();
schedule.schedule = {
months: [
{
month: 1,
daysOfMonth: [
{
dayOfMonth: 1,
times: [
{h: 0, m: 2, s: 33},
{h: 13, m: 12, s: 35},
{h: 22, m: 43, s: 49}
]
},
{
dayOfMonth: 12,
times: [
{h: 0, m: 2, s: 33},
{h: 13, m: 12, s: 35},
{h: 22, m: 43, s: 49}
]
},
{
dayOfMonth: 27,
times: [
{h: 0, m: 2, s: 33},
{h: 13, m: 12, s: 35},
{h: 22, m: 43, s: 49}
]
}
]
},
{
month: 12,
daysOfMonth: [
{
dayOfMonth: 1,
times: [
{h: 0, m: 2, s: 33},
{h: 13, m: 12, s: 35},
{h: 22, m: 43, s: 49}
]
},
{
dayOfMonth: 12,
times: [
{h: 0, m: 2, s: 33},
{h: 13, m: 12, s: 35},
{h: 22, m: 43, s: 49}
]
},
{
dayOfMonth: 27,
times: [
{h: 0, m: 2, s: 33},
{h: 13, m: 12, s: 35},
{h: 22, m: 43, s: 49}
]
}
]
}
]
};
Scheduler can have one of three statuses.
The scheduler is in a reset state. You need to set the task and schedule.
The scheduler is in a running state.
The scheduler is in a stopped state.
Scheduled task.
The number of times the task was performed on a schedule.
Date of the next start of the task.
Date of the previous start of the task.
History of the scheduler.
[ { act: 'START', datetime: '2018-11-24T14:41:36.729Z' },
{ act: 'RUN_TASK', datetime: '2018-11-24T14:41:36.825Z' },
{ act: 'RUN_TASK', datetime: '2018-11-24T14:41:36.875Z' },
{ act: 'STOP', datetime: '2018-11-24T14:41:36.900Z' },
{ act: 'START', datetime: '2018-11-24T14:41:37.000Z' },
{ act: 'RUN_TASK', datetime: '2018-11-24T14:41:37.726Z' },
{ act: 'STOP', datetime: '2018-11-24T14:41:37.801Z' } ]
The date from which the execution will begin. If null means execution will begin immediately.
End date. If the null scheduler will run indefinitely.
Method | Set status | Allowed with statuses | Description |
---|---|---|---|
start | RUNNING | READY or COMPLETED | Runs the scheduler. Before starting, you need to set the task and schedule. This event is recorded in history. Returns true or false. |
stop | COMPLETED | RUNNING | Stops the scheduler. This event is recorded in history. Returns true or false. |
reset | READY | any | Stops the scheduler. Resets to the original state. |
getInfo | nothing | any | Restores the current state of the scheduler. |
state
{
"datetimeStart": null,
"datetimeFinish": null,
"statusLists": {
"READY": "READY",
"RUNNING": "RUNNING",
"COMPLETED": "COMPLETED"
},
"status": "COMPLETED",
"schedule": {
"times": [
{
"h": 0,
"m": 0,
"s": 0,
"ms": 0
},
{
"h": 18,
"m": 2,
"s": 14,
"ms": 608
},
{
"h": 18,
"m": 2,
"s": 14,
"ms": 658
},
{
"h": 18,
"m": 2,
"s": 15,
"ms": 508
}
]
},
"countRun": 3,
"nextRun": "2018-11-24T21:00:00.000Z",
"prevRun": "2018-11-24T15:02:15.504Z",
"history": [
{
"act": "START",
"datetime": "2018-11-24T15:02:14.508Z"
},
{
"act": "RUN_TASK",
"datetime": "2018-11-24T15:02:14.608Z"
},
{
"act": "RUN_TASK",
"datetime": "2018-11-24T15:02:14.657Z"
},
{
"act": "STOP",
"datetime": "2018-11-24T15:02:14.678Z"
},
{
"act": "START",
"datetime": "2018-11-24T15:02:14.778Z"
},
{
"act": "RUN_TASK",
"datetime": "2018-11-24T15:02:15.504Z"
},
{
"act": "STOP",
"datetime": "2018-11-24T15:02:15.579Z"
}
],
"actLists": {
"START": "START",
"RUN_TASK": "RUN_TASK",
"STOP": "STOP"
}
}
const JrfScheduler = require('jrfscheduler');
function wait(mlsecond = 1000) {
return new Promise(resolve => setTimeout(resolve, mlsecond));
}
async function scheduleInterval() {
let schedule = new JrfScheduler();
schedule.schedule = {
interval: '100ms'
};
schedule.task = async () => {
dateFinish = new Date();
};
let now = new Date();
schedule.datetimeFinish = new Date(now.getTime() + 5000);
let dateFinish = null;
await schedule.start();
await wait(210);
await schedule.stop();
let info = await schedule.getInfo();
console.log(info);
await wait(100);
await schedule.start();
await wait(110);
await schedule.stop();
info = await schedule.getInfo();
console.log(info);
}
scheduleInterval();
FAQs
Async/await scheduler.
The npm package jrfscheduler receives a total of 0 weekly downloads. As such, jrfscheduler popularity was classified as not popular.
We found that jrfscheduler demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.
Security News
Research
Socket's threat research team has detected five malicious npm packages targeting Roblox developers, deploying malware to steal credentials and personal data.