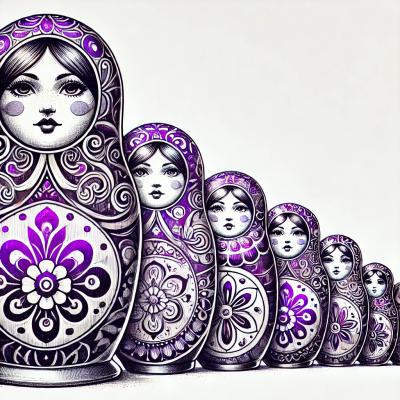
Research
Security News
Threat Actor Exposes Playbook for Exploiting npm to Build Blockchain-Powered Botnets
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
Read this in other languages: English | 简体中文
rc
> beta
> alpha
> other
${xxxx}
{{xxxx}}
and {xxxx}
# use pnpm
pnpm install js-cool
## use npm
npm install --save js-cool
import { osVersion } from 'js-cool'
osVersion()
const { osVersion } = require('js-cool')
osVersion()
<script src="https://unpkg.com/js-cool@latest/dist/index.global.prod.js"></script>
<script>
jsCool.browserVersion()
</script>
The client method returns a browser result object
Since: 1.0.1
Arguments: none
Returns: object
Example:
import { client } from 'js-cool'
client.get(['device', 'browser', 'engine', 'os']) // { device: 'xxx', browser: 'xxx', os: 'xxx', engine: 'xxx' }
client.get('device') // { device: 'xxx' }
declare class Client {
matchMap: Record<InfoKeys, boolean>
root: Window & typeof globalThis
navigator: Navigator
constructor(options: ClientOptions)
get(names?: InfoTypes | InfoTypes[]): Partial<{
device: InfoKeys | undefined
os: InfoKeys | undefined
browser: InfoKeys | undefined
engine: InfoKeys | undefined
language: any
network: any
orientation: string | undefined
}>
getInfoByType(infoKey: InfoKey): InfoKeys | undefined
getOrientationStatus(): 'vertical' | 'horizontal' | undefined
getNetwork(): any
getLanguage(): any
}
Collection of common regular expressions
Since: 1.0.1
Arguments: none
Returns: none
Example:
pattern.number.test('333') // true
declare const pattern: {
any: RegExp
number: RegExp
string: RegExp
postcode: RegExp
url: RegExp
username: RegExp
float: RegExp
email: RegExp
mobile: RegExp
chinese: RegExp
tel: RegExp
qq: RegExp
pass: RegExp
json: RegExp
arrjson: RegExp
array: RegExp
isjson: RegExp
textarea: RegExp
}
Remove all attributes of HTML tags
Since: 1.0.1
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
string | string with html tags | string | - | true | - |
Returns: string
Example:
clearAttr('<div id="testID">test</div>')
// '<div>test</div>'
declare function clearAttr(string: string): string
Remove HTML tags
Since: 1.0.1
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
string | string with html tags | string | - | true | - |
Returns: string
Example:
clearHtml('<div>test<br />string</div>')
// 'teststring'
declare function clearHtml(string: string): string
Escaping HTML Special Characters
Since: 5.5.0
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
string | string with html tags | string | - | true | - |
Returns: string
Example:
escape('<div>test<br />string</div>')
// '<div>test<br />string</div>'
declare function escape(string: string): string
Restore HTML Special Characters
Since: 5.5.0
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
string | string | string | - | true | - |
Returns: string
Example:
unescape('<div>test<br />string</div>')
// '<div>test<br />string</div>'
declare function unescape(string: string): string
Get the number in the string
Since: 1.0.1
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
string | pass in a string with a number | string | - | true | - |
Returns: string
Example:
getNumber('Chrome123.33')
// '123.33'
getNumber('234test.88')
// '234.88'
declare function getNumber(string: string): string
Converts humped strings to -spaced and all lowercase Dash pattern
Since: 1.0.1
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
string | the string to be converted | string | - | true | - |
Returns: string
Example:
camel2Dash('jsCool') // js-cool
declare function camel2Dash(string: string): string
Converts -spaced and all lowercase Dash patterns to humped strings
Since: 1.0.1
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
string | the string to be converted | string | - | true | - |
Returns: string
Example:
dash2Camel('js-cool') // jsCool
declare function dash2Camel(string: string): string
Generate random hexadecimal colors
Support for custom color value ranges starting with version 5.17.0, which can be used to customize the generation of darker, lighter, warmer colors, etc.
Since: 5.5.0
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
min | the minimum value of the random numbers | number / [number, number, number] | - | false | - |
max | the maximum value of the random numbers | number / [number, number, number] | - | false | - |
Returns: string
Example:
randomColor()
// #bf444b
randomColor(200)
// #d6e9d7
randomColor(200, 255)
// #d3f9e4
randomColor([0, 0, 0], [255, 255, 255])
// #e2f2f3
declare function randomColor(
min?: number | [number, number, number],
max?: number | [number, number, number]
): string
Get a random number
Since: 5.0.0
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
min | the minimum value of the random number | number | - | false | 1 |
max | the maximum value of the random number | number | - | false | 10 |
Returns: number
Example:
randomNumber() // 8
randomNumber(0.1, 0.9) // 0.8
declare function randomNumber(min?: number, max?: number): number
Generate n random integers that sum to a fixed sum
Since: 5.4.0
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
n | Number of generated integers | number | - | false | 1 |
sum | Sum of generated integers | number | - | false | 100 |
noZero | Generate integers that are not zero | boolean | - | false | true |
Returns: Array<number>
Example:
randomNumbers()
// [8]
randomNumbers(4, 5)
// [1, 1, 2, 1]
randomNumbers(4, 5, false)
// [0, 1, 2, 2]
declare function randomNumbers(n?: number, sum?: number): number[]
Get a random string
v5.4.0
widthSpecialChar
changed tooptions
, still compatible with old usage, widthSpecialChar=true equivalent to { charTypes: ['uppercase', 'lowercase', 'number', 'special'] }
Since: 5.0.0
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
len | the length of the random string that needs to be obtained | number | - | false | 32 |
options | randomString options | RandomStringOptions boolean | - | false | { charTypes: ['uppercase', 'lowercase', 'number'] } |
Returns: string
Example:
// 1. No parameters are passed, a 32-bit (possibly) string containing upper and lower case letters and numbers is generated by default
randomString()
// PVSjz902EqYbmxaLtvDnggtnlvt5uFTZ
// 2. Generate a 16-bit random string
randomString(16)
// coTgZy0mqqMJ1sMM
// 3. Same effect as #2 above
randomString({
length: 16
})
// ngCI5aPqJm84t90d
// 4. Generate containing special characters (old way of passing values, not recommended)
randomString(true)
// 0Uby@op3B-sK5]dHl4S|15As.OlHiNXd
// 5. Same effect as #4 above (recommended)
randomString({
charTypes: ['uppercase', 'lowercase', 'number', 'special']
})
// m,2^vpkrE,F,DbcSFk0=vr&@DJ27j9XK
// 6. Same effect as #4 above, Limit string length to 16 bits
randomString(16, true)
// dXz[J_sYM^3d8fnA
// 7. Generate a 16-bit random number
randomString({
length: 16,
charTypes: 'number'
})
// 7450026301030286
// 8. Elimination of confusing characters: oOLl,9gq,Vv,Uu,I1
randomString({
length: 16,
noConfuse: true
})
// 8DEGna8ppC4mqyew
// 9. The generated random string must contain at least 1 character of each type of character specified, e.g. to generate a 16-bit password that must contain upper and lower case letters, numbers, and special characters.
randomString({
length: 16,
strict: true
})
// PFYAPD5KFqOHIADL
declare function randomString(len?: number, options?: RandomStringOptions | boolean): string
declare function randomString(
len?: RandomStringOptions | boolean,
options?: RandomStringOptions | boolean
): string
declare type RandomStringCharType = 'uppercase' | 'lowercase' | 'number' | 'special'
declare interface RandomStringOptions {
length?: number
charTypes?: RandomStringCharType | ArrayOneMore<RandomStringCharType>
/**
* Elimination of confusing characters: oOLl,9gq,Vv,Uu,I1
*/
noConfuse?: boolean
/**
* The generated random string must contain each of the listed character types
*/
strict?: boolean
}
shuffling algorithm, Reordering arrays or strings
Since: 5.4.0
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
value | arrays or strings | array string | - | true | - |
size | new array or string length | number | - | false | - |
Returns: array | string
Example:
const str = 'abcde'
const arr = [1, 2, 3]
shuffle(str)
// cdbse
shuffle(arr)
// [3, 1, 2]
shuffle(arr, 2)
// [3, 2]
declare function shuffle(value: string, size?: number): string
declare function shuffle<T extends unknown[] = unknown[]>(value: T, size?: number): T
Generating Browser Fingerprints
Since: 5.2.0
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
domain | key string | string | - | false | location.host |
Returns: string
Example:
fingerprint('www.saqqdy.com') // wc7sWJJA8
declare function fingerprint(domain?: string): string | null
Get the length of the string, Chinese counts as 2 characters
Since: 1.0.1
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
str | input string | string | - | true | - |
Returns: number
Example:
getCHSLength('测试') // 2
declare function getCHSLength(str: string): number
Intercept string, Chinese counts as 2 bytes
Since: 1.0.1
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
str | the string to be intercepted | string | - | true | - |
len | length | number | - | false | - |
hasDot | output with dot | boolean | - | false | false |
Returns: string
Example:
cutCHSString('测试', 1) // 测
cutCHSString('测试', 1, true) // 测...
declare function cutCHSString(str: string, len?: number, hasDot?: boolean): string
First letter capitalized
Since: 1.0.1
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
string | the string to be converted | string | - | true | - |
Returns: string
Example:
upperFirst('saqqdy') // Saqqdy
declare function upperFirst(string: string): string
Determine if a string is a number
Since: 1.0.1
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
str | the string to be tested | string | - | true | - |
Returns: boolean
Example:
isDigitals('2.11') // true
isDigitals('2.3x') // false
declare function isDigitals(str: string): boolean
Determine if a function is defined
Since: 1.0.1
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
name | function name | string | - | true | - |
Returns: boolean
Example:
isExitsFunction('test') // false
isExitsFunction('console.log') // true
declare function isExitsFunction(name: string): boolean
Determine if a variable is defined
Since: 1.0.1
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
name | variable name | string | - | true | - |
Returns: boolean
Example:
isExitsVariable('test') // false
isExitsVariable('window') // true
declare function isExitsVariable(name: string): boolean
Determine if 2 objects are equal
Since: 5.12.0
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
a | source | any | - | true | - |
b | compare | any | - | true | - |
Returns: boolean
Example:
isEqual({ a: 22, b: {} }, { b: {}, a: 22 })
// true
isEqual([1, 2], [2, 1])
// false
isEqual(NaN, NaN)
// true
declare function isEqual<T, P>(a: T, b: P): boolean
Determine if window object
Since: 5.0.0
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
target | any target | any | - | true | - |
Returns: boolean
Example:
isWindow({}) // false
isWindow(window) // true
declare function isWindow<T = any>(target: T): target is Window
Determine if target is an plain object
Since: 5.0.0
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
target | any target | any | - | true | - |
Returns: boolean
Example:
isPlainObject({}) // true
isPlainObject(window) // false
type Primitive = bigint | boolean | null | number | string | symbol | undefined
type JSONValue = Primitive | PlainObject | JSONArray
// eslint-disable-next-line @typescript-eslint/consistent-indexed-object-style
interface PlainObject {
[key: string]: JSONValue
}
interface JSONArray extends Array<JSONValue> {}
declare function isPlainObject(target: unknown): target is PlainObject
Determine if dark color mode
Since: 5.5.0
Arguments: none
Returns: boolean
Example:
isDarkMode() // false
declare function isDarkMode(): boolean
Determine if target is an object
Since: 5.0.0
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
target | any target | any | - | true | - |
Returns: boolean
Example:
isObject({}) // true
declare function isObject<T = any>(target: T): target is Object
Determine if target is Date
Since: 5.15.0
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
target | any target | any | - | true | - |
Returns: boolean
Example:
const now = new Date()
isDate(now)
// true
declare function isDate<T = any>(target: T): target is Date
Determine if target is RegExp
Since: 5.15.0
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
target | any target | any | - | true | - |
Returns: boolean
Example:
isRegExp(/\d/) // true
declare function isRegExp<T = any>(target: T): target is RegExp
Determine if it is an array
Since: 1.0.2
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
target | any target | any | - | true | - |
Returns: boolean
Example:
isArray([]) // true
declare function isIterable(target: any): target is any[]
Determine if it is iterable
Since: 5.7.0
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
target | any target | any | - | true | - |
Returns: boolean
Example:
isIterable([]) // true
declare function isIterable<T = any>(target: T | Iterable<T>): target is Iterable<T>
Determine if it is running on the browser side
Since: 4.5.0
Arguments: none
Returns: boolean
Example:
function test() {
if (!inBrowser) return null
// ...
}
declare const inBrowser: boolean
Determine if it is running on node.js
Since: 5.13.0
Arguments: none
Returns: boolean
Example:
if (inNodeJs) {
//
}
declare const inNodeJs: boolean
Get the window size
Since: 1.0.1
Arguments: none
Returns: { width, height }
Example:
windowSize()
// { width: 1280, height: 800 }
declare interface WindowSizeObj {
width: number
height: number
}
declare function windowSize(): WindowSizeObj
Get the APP version number
deprecated please use 'appVersion' instead
Since: 1.0.1
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
appName | app name | string | - | true | - |
withApp | whether to bring the name | boolean | - | false | - |
userAgent | ua or any ua like string, may not be passed | string | - | false | navigator.userAgent |
Returns: string | boolean | null
Example:
// navigator.userAgent => '5.0 (Macintosh; Intel Mac OS X 10_15_7) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/114.0.0.0 Safari/537.36 AppName/1.0.0-beta.8'
getAppVersion('Chrome') // 114.0.0.0
getAppVersion('Safari', true) // Safari/537.36
declare function getAppVersion(
appName: string,
withApp?: boolean,
userAgent?: string
): string | boolean | null
Get the app version number
Since: 5.1.0
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
appName | app name | string | - | true | - |
ua | ua or any ua like string, may not be passed | string | - | false | navigator.userAgent |
ignoreCase | whether to ignore case | boolean | true /false | false | true |
Returns: string | null
Example:
// navigator.userAgent => '5.0 (Macintosh; Intel Mac OS X 10_15_7) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/114.0.0.0 Safari/537.36 AppName/1.0.0-beta.8'
appVersion('Chrome') // 114.0.0.0
appVersion('Safari') // 537.36
appVersion('appname', false) // null
appVersion('appname') // 1.0.0-beta.8
declare function appVersion(appName: string): string | null
declare function appVersion(appName: string, ua: string): string | null
declare function appVersion(appName: string, ua: boolean): string | null
declare function appVersion(appName: string, ua: string, ignoreCase: boolean): string | null
Get the phone system version
deprecated: please use 'osVersion' instead
Since: 1.0.1
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
osName | system type string Android, iPod, iWatch or iPhone | string | - | true | - |
withOS | whether to bring the name | string | - | false | - |
userAgent | ua or any ua like string, may not be passed | string | - | false | navigator.userAgent |
Returns: string | boolean | null
Example:
getOsVersion('iPhone')
// '13.2.3'
getOsVersion('iPhone', true)
// 'iPhone/13.2.3'
declare function getOsVersion(
osName: string,
withOS?: boolean,
userAgent?: string
): string | boolean | null
get the system version
Since: 5.1.0
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
ua | ua or any ua like string, may not be passed | string | - | false | navigator.userAgent |
Returns: OsVersion | null
Example:
// ipad => 'Mozilla/5.0 (iPad; CPU OS 13_3 like Mac OS X) AppleWebKit/605.1.15 (KHTML, like Gecko) CriOS/87.0.4280.77 Mobile/15E148 Safari/604.1'
osVersion() // \{ name: 'iOS', version: '13.3' \}
// iphone => 'Mozilla/5.0 (iPhone; CPU iPhone OS 13_2_3 like Mac OS X) AppleWebKit/605.1.15 (KHTML, like Gecko) Version/13.0.3 Mobile/15E148 Safari/604.1'
osVersion() // \{ name: 'iOS', version: '13.2.3' \}
// mac os => 'Mozilla/5.0 (Macintosh; Intel Mac OS X 10_15_7) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/114.0.0.0 Safari/537.36'
osVersion() // \{ name: 'MacOS', version: '10.15.7' \}
// windows => 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/114.0.0.0 Safari/537.36'
osVersion() // \{ name: 'Windows', version: '10.0' \}
// windows xp => 'Mozilla/5.0 (Windows NT 5.2; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/114.0.0.0 Safari/537.36'
osVersion() // \{ name: 'Windows', version: 'XP' \}
// windows phone => 'Mozilla/5.0 (Windows Phone OS 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/98.0.4758.82 Safari/537.36'
osVersion() // \{ name: 'WindowsPhone', version: '10.0' \}
declare interface OsVersion {
name: 'Windows' | 'MacOS' | 'Android' | 'iOS' | 'WindowsPhone' | 'Debian' | 'WebOS'
version: string
}
declare function osVersion(ua?: string): OsVersion | null
Get the browser name and version
Since: 5.2.0
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
ua | ua or any ua like string, may not be passed | string | - | false | navigator.userAgent |
Returns: BrowserVersion | null
Example:
// Mozilla/5.0 (Macintosh; Intel Mac OS X 10_15_7) Ap…KHTML, like Gecko) Chrome/114.0.0.0 Safari/537.36
browserVersion() // \{ name: 'Chrome', version: '114.0.0.0' \}
declare interface BrowserVersion {
name: 'Windows' | 'MacOS' | 'Android' | 'iOS' | 'WindowsPhone' | 'Debian' | 'WebOS'
version: string
}
declare function browserVersion(ua?: string): BrowserVersion | null
v5.8.0 support compare tag version
Version number size comparison, tag version: rc
> beta
> alpha
> other
Since: 4.7.0
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
input | input version | string | - | true | - |
compare | compare version | string | - | true | - |
Returns: 0 | 1 | -1
Example:
compareVersion('1.11.0', '1.9.9')
// => 1: 1=Version 1.11.0 is newer than 1.9.9
compareVersion('1.11.0', '1.11.0')
// => 0: 0=Versions 1.11.0 and 1.11.0 are the same
compareVersion('1.11.0', '1.99.0')
// => -1: -1=Version 1.11.0 is older than 1.99.0
compareVersion('1.0.0.0.0.10', '1.0')
// => -1
// compare tag version: rc > beta > alpha > other
compareVersion('1.11.0', '1.11.0-beta.1')
// => -1
compareVersion('1.11.0-beta.1', '1.11.0')
// => -1
compareVersion('1.11.0-beta.10', '1.11.0-beta.10')
// => 0
compareVersion('1.11.0-alpha.10', '1.11.0-beta.1')
// => -1
compareVersion('1.11.0-alpha.10', '1.11.0-rc.1')
// => -1
compareVersion('1.11.0-tag.10', '1.11.0-alpha.1')
// => -1
compareVersion('1.11.0-tag.10', '1.11.0-tag.1')
// => 1
compareVersion('1.11.0-release.10', '1.11.0-tag.1')
// => 1
declare function compareVersion(input: string, compare: string): 0 | 1 | -1
parse url params. (If covert is passed true: Scientific notation, binary, octal and hexadecimal types of data are not converted, like: 0b111, 0o13, 0xFF, 1e3, -1e-2)
Since: 5.0.0
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
url | url string (like: ?key1=value1&key2=value2) | string | - | true | - |
covert | Converts a specific string to a corresponding value | boolean | true /false | false | false |
Returns: object
Example:
parseUrlParam(
'?key1=100&key2=true&key3=null&key4=undefined&key5=NaN&key6=10.888&key7=Infinity&key8=test'
)
// \{"key1":"100","key2":"true","key3":"null","key4":"undefined","key5":"NaN","key6":"10.888","key7":"Infinity","key8":"test"\}
parseUrlParam(
'?key1=100&key2=true&key3=null&key4=undefined&key5=NaN&key6=10.888&key7=Infinity&key8=test',
true
)
// \{"key1":100,"key2":true,"key3":null,"key5":NaN,"key6":10.888,"key7":Infinity,"key8":"test"\}
declare function parseUrlParam(url: string, covert?: boolean): Record<string, unknown>
Splice URL parameters (single layer only)
Since: 5.3.0
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
params | json object | object | - | true | - |
covert | Convert a null value type (null/undefined/) to an empty string | boolean | true /false | false | false |
withQuestionsMark | Splicing a question mark | boolean | true /false | false | true |
Returns: string
Example:
spliceUrlParam({ key1: '100', key2: 'true', key3: 'null', key4: 'undefined', key4: '测试' })
// ?key1=100&key2=true&key3=null&key4=undefined&key5=%E6%B5%8B%E8%AF%95
spliceUrlParam({ key1: '100', key2: 'true', key3: 'null', key4: 'undefined' }, true)
// ?key1=100&key2=true&key3=&key4=
spliceUrlParam({ key1: '100', key2: 'true', key3: 'null', key4: 'undefined' }, true, false)
// key1=100&key2=true&key3=&key4=
declare function spliceUrlParam(
params: Record<string, unknown>,
covert?: boolean,
withQuestionsMark?: boolean
): string | null
Secure parsing of JSON strings
support BigInt since
v5.17.1
Since: 5.16.0
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
data | JSON string | string | - | true | - |
covert | Whether to convert data | boolean | true /false | false | true |
Returns: Object
Example:
safeParse('100')
// 100
safeParse('{"a":"undefined","b":"NaN","c":"Infinity","d":"9007199254740993"}')
// { b: NaN, c: Infinity, d: 9007199254740993n }
declare function safeParse(data: string, covert?: boolean): any
Secure stringify of JSON Object
support BigInt since
v5.17.1
Since: 5.16.0
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
data | JSON Object | any | - | true | - |
covert | Whether to convert data | boolean | true /false | false | true |
Returns: string
Example:
safeStringify(100)
// "100"
safeStringify(undefined)
// "undefined"
safeStringify(NaN)
// "NaN"
safeStringify(Infinity)
// "Infinity"
safeStringify({ a: undefined, b: NaN, c: Infinity, d: BigInt(Number.MAX_SAFE_INTEGER) + 2n })
// {"a":"undefined","b":"NaN","c":"Infinity","d":"9007199254740993"}
declare function safeStringify(data: any, covert?: boolean): string
Get the URL parameter in the form of a directory
It will be refactored and renamed getDirParams in the next major release.
Since: 1.0.1
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
url | http url | object | - | true | - |
Returns: object
Example:
//
declare interface DirParamType {
path?: string[]
host?: string
}
declare function getDirParam(url: string): DirParamType
Get a single query parameter (behind "#")
Since: 5.0.0
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
key | key name | string | - | true | - |
url | pass in the url string | string | - | false | location.href |
Returns: string
Example:
getQueryParam('key1')
// key1 => xxx
getQueryParam('key1', 'https://test.com?key1=100#/home?key1=200')
// key1 => 200
declare function getQueryParam(key: string): string | undefined
declare function getQueryParam(key: string, url: string): string | undefined
Get all query parameters (behind "#"). (If covert is passed true: Scientific notation, binary, octal and hexadecimal types of data are not converted, like: 0b111, 0o13, 0xFF, 1e3, -1e-2)
Since: 5.0.0
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
url | pass in the url string | string | - | false | location.href |
covert | Converts a specific string to a corresponding value | boolean | true /false | false | false |
Returns: object
Example:
getQueryParams('https://test.com?key1=100#/home?key1=200')
// \{"key1":"200"\}
getQueryParams('https://test.com?key1=100#/home?key1=200', true)
// \{"key1":200\}
getQueryParams(true)
// \{"key1":200\}
declare function getQueryParams(url?: string, covert?: boolean): Record<string, unknown> | null
Get a single URL parameter (from the "location.search", before "#")
Since: 5.0.0
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
key | key name | string | - | true | - |
url | pass in the url string | string | - | false | location.href |
Returns: string
Example:
getUrlParam('key1')
// key1 => xxx
getUrlParam('key1', 'https://test.com?key1=100#/home?key1=200')
// key1 => 100
declare function getUrlParam(key: string): string | undefined
declare function getUrlParam(key: string, url: string): string | undefined
Get all URL parameters (from the "location.search", before "#"). (If covert is passed true: Scientific notation, binary, octal and hexadecimal types of data are not converted, like: 0b111, 0o13, 0xFF, 1e3, -1e-2)
Since: 5.0.0
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
url | pass in the url string | string | - | false | location.href |
covert | Converts a specific string to a corresponding value | boolean | true /false | false | false |
Returns: object
Example:
getUrlParams('https://test.com?key1=100#/home?key1=200')
// \{"key1":"100"\}
getUrlParams('https://test.com?key1=100#/home?key1=200', true)
// \{"key1":100\}
getUrlParams(true)
// \{"key1":100\}
declare function getUrlParams(url?: string, covert?: boolean): Record<string, unknown> | null
Get the cache, if the deposited is Object, the retrieved is also Object, no need to convert again
Since: 1.0.2
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
name | cache key name | string | - | true | - |
Returns: any
Example:
import { getCache, setCache } from 'js-cool'
const data1 = 100
const data2 = { a: 10 }
const data3 = null
setCache('data1', data1)
setCache('data2', data2)
setCache('data3', data3)
getCache('data1') // 100
getCache('data2') // {a:10}
getCache('data3') // null
getCache('data4') // null
declare function getCache(name: string): any
Set the cache, if the deposited is Object, the retrieved is also Object, no need to convert again
Since: 1.0.2
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
name | cache key name | string | - | true | - |
value | cache data, can be passed directly into Object | any | - | true | - |
seconds | cache time (seconds) | number | - | false | - |
Returns: void
Example:
import { getCache, setCache } from 'js-cool'
const data1 = 100
const data2 = { a: 10 }
const data3 = null
setCache('data1', data1)
setCache('data2', data2, 10)
getCache('data1') // 100
getCache('data2') // {a:10}
setTimeout(() => {
getCache('data2') // null
}, 15000)
declare function setCache<T = unknown>(name: string, value: T, seconds?: number | string): void
Delete localStorage
Since: 1.0.2
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
name | cache key name | string | - | true | - |
Returns: void
Example:
delCache('data')
declare function delCache(name: string): void
Get the session, if the deposited is Object, the retrieved is also Object, no need to convert again
Since: 1.0.2
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
name | session key name | string | - | true | - |
Returns: any
Example:
const data1 = 100
const data2 = { a: 10 }
const data3 = null
setSession('data1', data1)
setSession('data2', data2)
setSession('data3', data3)
getSession('data1') // 100
getSession('data2') // {a:10}
getSession('data3') // null
getSession('data4') // null
declare function getSession(name: string): any
Set the session, if the deposited is Object, the retrieved is also Object, no need to convert again
Since: 1.0.2
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
name | session key name | string | - | true | - |
value | session data, can be passed directly into Object | any | - | true | - |
seconds | session time (seconds) | number | - | false | - |
Returns: void
Example:
import { getSession, setSession } from 'js-cool'
const data1 = 100
const data2 = { a: 10 }
const data3 = null
setSession('data1', data1)
setSession('data2', data2, 10)
getSession('data1') // 100
getSession('data2') // {a:10}
setTimeout(() => {
getSession('data2') // null
}, 15000)
declare function setSession<T = unknown>(name: string, value: T, seconds?: number | string): void
Delete sessionStorage
Since: 1.0.2
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
name | session key name | string | - | true | - |
Returns: void
Example:
delSession('data')
declare function delSession(name: string): void
Get cookie by name
Since: 1.0.2
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
name | cookie key name | string | - | true | - |
Returns: any
Example:
getCookie('data1') // 100
declare function getCookie(name: string): string
Get all cookies
Since: 5.6.0
Arguments: 'none'
Returns: object
Example:
getCookies()
// { token: 'xxx', name: 'saqqdy' }
declare function getCookies(): Record<string, string>
Set cookie
Since: 1.0.2
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
name | cookie key name | string | - | true | - |
value | cookie data, can be passed directly into Object | any | - | true | - |
seconds | cookie time (seconds) | number | - | false | - |
path | cookie path | string | - | false | / |
samesite | SameSite type | string | Strict /Lax /None | false | None |
Returns: void
Example:
import { getCookie, setCookie } from 'js-cool'
const data1 = 100
const data2 = 200
setCookie('data1', data1)
setCookie('data2', data2, 10)
getCookie('data1') // 100
getCookie('data2') // 200
setTimeout(() => {
getCookie('data2') // null
}, 15000)
declare function setCookie<T extends string | number | boolean>(
name: string,
value: T,
seconds: string | number,
path?: string,
samesite?: boolean
): void
Delete cookie
Since: 1.0.2
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
name | cookie key name | string | - | true | - |
Returns: void
Example:
delCookie('data')
declare function delCookie(name: string): void
convert strings, numbers to base64
Since: 1.0.1
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
input | the string to be encoded | string /number | - | true | - |
Returns: void
Example:
encodeBase64('data')
declare function encodeBase64(name: string): string
base64 decoding
Since: 1.0.1
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
input | the string to be decoded | string /number | - | true | - |
Returns: void
Example:
decodeBase64('data')
declare function decodeBase64(name: string): string
convert strings, numbers to utf8
Since: 1.0.1
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
input | the string to be encoded | string /number | - | true | - |
Returns: void
Example:
encodeUtf8('data')
declare function encodeUtf8(name: string): string
utf8 decoding
Since: 1.0.1
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
input | the string to be decoded | string /number | - | true | - |
Returns: void
Example:
decodeUtf8('data')
declare function decodeUtf8(name: string): string
stop bubbling
Since: 1.0.2
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
e | dom's event object | Event | - | true | - |
Returns: boolean
Example:
stopBubble(event)
declare function stopBubble(e: Event): boolean
stop default events
Since: 1.0.2
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
e | dom's event object | Event | - | true | - |
Returns: boolean
Example:
stopDefault(event)
declare function stopDefault(e: Event): boolean
event delegate, support multiple delegates
Since: 1.0.2
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
element | js dom object | HTMLElement | - | true | - |
type | The type of the event. No need to add on | string | - | true | - |
handler | Callback method | function | - | true | - |
Returns: void
Example:
addEvent(document, 'click', functionName)
declare function addEvent(element: AnyObject, type: string, handler: AnyFunction): void
removeEvent removes the event delegate created by addEvent
Since: 1.0.2
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
element | js dom object | HTMLElement | - | true | - |
type | The type of the event. No need to add on | string | - | true | - |
handler | Callback method | function | - | true | - |
Returns: void
Example:
removeEvent(document, 'click', functionName)
declare function removeEvent(element: AnyObject, type: string, handler: AnyFunction): void
Get slide to top and bottom return 'top' 'bottom', recommend using limit flow
Since: 1.0.2
Arguments: none
Returns: 'top' | 'bottom' | undefined
Example:
getScrollPosition() // ‘bottom’
declare function getScrollPosition(): string | void
return the next zIndex value
change mix defaults to 0
Since: 1.0.2
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
min | minimum value | number | - | false | 0 |
max | maximum value | number | - | false | - |
Returns: number
Example:
nextIndex() // 1
nextIndex(1000) // 1001
nextIndex(10, 100) // 100
declare function nextIndex(min?: number, max?: number): number
return the next version, Only version types with no more than 3 digits are supported. (Follow the npm version rules)
Since: 5.10.0
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
version | version(like: 1.0.0) | string | - | true | - |
type | version type | major | minor | patch | premajor | preminor | prepatch | prerelease | - | false | patch |
preid | prerelease id | string | - | false | '' |
Returns: string
Example:
nextVersion('1.2.33') // 1.2.34
nextVersion('1.2.33', 'major') // 2.0.0
nextVersion('1.2.33', 'premajor', 'alpha') // 2.0.0-alpha.1
declare function nextVersion(
version: string,
type?: 'major' | 'minor' | 'patch' | 'premajor' | 'preminor' | 'prepatch' | 'prerelease',
preid?: string
): string
punctual setInterval
Since: 5.18.0
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
handler | A function to be executed after the timer expires. | function | - | true | - |
delay | The time, in milliseconds that the timer should wait before the specified function or code is executed. If this parameter is omitted, a value of 0 is used, meaning execute "immediately", or more accurately, the next event cycle. | number | - | true | - |
...args | Additional arguments which are passed through to the function specified by handler. | any[] | - | false | - |
Returns: void
Example:
const printDate = () => console.log(new Date())
punctualTimer(printDate, 1000)
declare function punctualTimer<TArgs extends any[]>(
handler: (args: void) => void,
delay: number,
[...args]?: TArgs
): void
declare function punctualTimer<TArgs extends any[]>(
handler: (...args: TArgs) => void,
delay: number,
[...args]?: TArgs
): void
Convert an object to a promise like api
Since: 5.10.0
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
original | original object | object | - | true | - |
resolver | resolver function | Function | - | true | - |
Returns: T & PromiseLike<T>
Example:
import { promiseFactory, waiting } from 'js-cool'
function promise() {
const stats = {
value: 100
}
const resolver = () =>
new Promise(resolve =>
waiting(2000).then(() => {
stats.value = 200
resolve(stats)
})
)
return promiseFactory(stats, resolver)
}
const res = promise()
// res => 100
const res = await promise()
// res => 200
declare function promiseFactory<T extends object>(
original: T,
resolver: () => Promise<any>
): T & PromiseLike<T>
truncate a few decimal places, not 0 for shortage
Since: 1.0.2
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
number | the number of digits to be processed | number /string | - | true | - |
n | the number of decimal places to keep | number | - | false | 2 |
Returns: string | number
Example:
fixNumber('100.888')
// 100.88
fixNumber('100.8', 2)
// 100.8
fixNumber('100.8888', 3)
// 100.888
declare function fixNumber(number: string | number, n?: number): number
Replacing specific data in a template string, support ${xxxx}
{{xxxx}}
and {xxxx}
Since: 5.9.0
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
tmp | Template string | string | - | true | - |
data | Template data of map function | Function | Object | - | true | - |
Returns: string
Example:
const tmp = "My name is ${name}, I'm ${age} years old."
mapTemplate(tmp, {
name: 'saqqdy',
age: 18
})
// My name is saqqdy, I'm 18 years old.
mapTemplate(tmp, key => ({ name: 'saqqdy', age: 28 })[key])
// My name is saqqdy, I'm 28 years old.
const tmp1 = "My name is {{name}}, I'm {{age}} years old."
mapTemplate(tmp1, {
name: 'saqqdy',
age: 18
})
// My name is saqqdy, I'm 18 years old.
declare function mapTemplate(
tmp: string,
data: ((value: string) => unknown) | Record<string, unknown>
): string
deep copy & merge objects
Since: 1.0.2
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
target | boolean or array or object | boolean /ArrayOneMore<ExtendData> | - | true | - |
...args | array or object | ArrayOneMore<ExtendData> | - | true | - |
Returns: array | object
Example:
extend(true, {}, {})
declare function extend(
target: ExtendObjectData,
...args: ArrayOneMore<ExtendObjectData>
): ExtendObjectData
declare function extend(target: boolean, ...args: ArrayOneMore<ExtendObjectData>): ExtendObjectData
declare function extend(
target: ExtendArrayData,
...args: ArrayOneMore<ExtendArrayData>
): ExtendArrayData
declare function extend(target: boolean, ...args: ArrayOneMore<ExtendArrayData>): ExtendArrayData
declare type ExtendArrayData = any[]
declare type ExtendData = ExtendArrayData | ExtendObjectData
declare type ExtendObjectData = Record<string, any>
deep copy (Buffer, Promise, Set, Map are not supported)
Since: 5.15.0
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
source | source object | any | - | true | - |
Returns: object
Example:
const source = { a: 100, reg: /\d+/g, arr: [1, 2] }
const res = clone(source)
// { a: 100, reg: /\d+/g, arr: [1, 2] }
declare function clone<T = any>(parent: T): T
anti-dither throttling
Since: 1.0.2
Arguments: none
Returns: void
Example:
const delay = new Delay()
delay.register('key', () => {
//
})
declare function delay(): {
map: any
register(id: string, fn: AnyFunction, time: number, boo: boolean): void
destroy(id: string): void
}
Get the target type
Since: 1.0.2
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
target | any target | any | - | true | - |
Returns: string
Example:
getType({}) // object
getType([]) // array
getType(new Promise()) // promise
getType(new Date()) // date
getType(async () => {}) // function
getType(navigator) // navigator
getType(global) // global
getType(window) // window
getType(Symbol('')) // symbol
declare function getType<T = any>(
target: T
):
| 'string'
| 'number'
| 'bigint'
| 'boolean'
| 'symbol'
| 'undefined'
| 'object'
| 'function'
| 'window'
| 'error'
| 'promise'
| 'math'
| 'document'
| 'navigator'
| 'global'
| 'array'
| 'date'
| 'regexp'
| 'null'
Determine file type based on link suffix
Since: 5.11.0
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
url | file url | string | - | true | - |
Returns: object
Example:
getFileType('/name.png')
// { "suffix": "png", "type": "image" }
getFileType('/name.PDF')
// { "suffix": "pdf", "type": "pdf" }
getFileType('/name.xyz')
// { "suffix": "xyz", "type": "other" }
declare function getFileType(url: string): {
suffix: string
type: 'audio' | 'video' | 'image' | 'other' | 'word' | 'txt' | 'excel' | 'pdf' | 'ppt' | 'zip'
}
Sorter factory function
Since: 5.14.0
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
locales | A string with a BCP 47 language tag, or an array of such strings | string Array | - | false | - |
options | An object adjusting the output format. | Intl.CollatorOptions | - | false | - |
Returns: Function
Example:
const items = ['啊我', '波拉', 'abc', 0, 3, '10', ',11', 13, null, '阿吧', 'ABB', 'BDD', 'ACD', 'ä']
items.sort(
sorter('zh-Hans-CN', {
ignorePunctuation: true,
sensitivity: 'variant',
numeric: true
})
)
// [ 0, 3, "10", ",11", 13, "ä", "ABB", "abc", "ACD", "BDD", null, "阿吧", "啊我", "波拉" ]
declare function sorter(
locales?: string | string[],
options?: Intl.CollatorOptions
): <T = string, P = string>(a: T, b: P) => number
Sort Chinese by Chinese phonetic alphabet
Since: 5.14.0
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
a | The first element for comparison. | any | - | true | - |
b | The second element for comparison. | any | - | true | - |
options | An object adjusting the output format. | Intl.CollatorOptions | - | false | - |
Returns: number
Example:
const items = ['啊我', '波拉', 'abc', 0, 3, '10', ',11', 13, null, '阿吧', 'ABB', 'BDD', 'ACD', 'ä']
items.sort(sortPinyin)
// [ ",11", 0, "10", 13, 3, "ä", "ABB", "abc", "ACD", "BDD", null, "阿吧", "啊我", "波拉" ]
items.sort((a, b) => sortPinyin(a, b, { ignorePunctuation: true, numeric: true }))
// [ 0, 3, "10", ",11", 13, "ä", "ABB", "abc", "ACD", "BDD", null, "阿吧", "啊我", "波拉" ]
declare function sortPinyin<T = string, P = string>(
a: T,
b: P,
options?: Intl.CollatorOptions
): number
Data cleaning methods
Since: 1.0.2
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
data | the object to be cleaned | object | - | true | - |
map | the data queue to be cleaned, can be passed as array or object | array /object | - | true | - |
nullFix | the value returned if there is no corresponding property, the default does not return the property | any | - | false | - |
Returns: any
Example:
//
declare function cleanData(data: any, map: any[] | AnyObject, nullFix?: any): any
Several ways of file downloading:
Since: 1.0.5
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
url | url link | string | - | true | - |
filename | file name | string | - | true | - |
type | download type | string | href /open /download /request | false | download |
Returns: void
Example:
download('https://unpkg.com/browse/js-cool@5.2.0/dist/index.global.prod.js')
declare function download(url: string, filename: string, type?: string): void
tree object depth lookup
Since: 5.0.0
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
tree | tree object | array /object | - | true | - |
expression | required Query method | any | - | true | - |
keySet | optional Default subclass name, query name | SearchKeySet | - | true | - |
number | optional Number of lookups, if not passed, query all | number | - | false | - |
Returns: any
Example:
//
declare function searchObject(
tree: object | any[],
expression: any,
keySet: SearchKeySet,
number?: number
): any[]
Open link in new tab (file jump download if browser can't parse)
Since: 1.0.6
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
url | http url | string | - | true | - |
Returns: boolean | undefined
Example:
openUrl('https://www.saqqdy.com/js-cool')
declare function openUrl(url: string): void
copy to clipboard
Since: 5.0.0
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
value | any value | any | - | true | - |
Returns: boolean | undefined
Example:
copy('10000')
declare function copy(value: string): boolean | undefined
Digital thousandths division
Since: 3.0.0
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
num | the number | string /number | - | true | - |
Returns: string
Example:
toThousands(10000) // '10,000'
declare function toThousands(num: string | number): string
return true if the provided predicate function returns true for all elements in a set, otherwise return false
Since: 1.0.9
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
arr | the target array | array | - | true | - |
fn | the judgment method | function | - | true | - |
Returns: boolean
Example:
all([4, 2, 3], x => x > 1)
// true
declare const all: <T = unknown>(arr: T[], fn: AnyFunction) => boolean
Returns true if the provided predicate function returns true for at least one element of a set, false otherwise
Since: 1.0.9
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
arr | the target array | array | - | true | - |
fn | the judgment method | function | - | true | - |
Returns: boolean
Example:
any([0, 1, 2, 0], x => x >= 2)
// true
declare const any: <T = unknown>(arr: T[], fn: AnyFunction) => boolean
generate uuid on browser side, use v4 method
Since: 1.0.9
Arguments: none
Returns: string
Example:
uuid() // '4222fcfe-5721-4632-bede-6043885be57d'
declare const uuid: () => string
Converts a comma-separated string of values (CSV) to a 2D array.
Since: 1.0.9
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
data | csv data | string | - | true | - |
delimiter | delimiter | string | - | false | ',' |
omitFirstRow | the first row is the table header data | boolean | - | false | false |
Returns: string
Example:
CSVToArray('a,b\\nc,d') // `[['a','b'],['c','d']]`
CSVToArray('a;b\\\nc;d', ';') // `[['a','b'],['c','d']]`
CSVToArray('col1,col2\\\na,b\\\nc,d', ',', true) // `[['a','b'],['c','d']]`
declare const CSVToArray: (data: string, delimiter?: string, omitFirstRow?: boolean) => string[][]
Converts a two-dimensional array to a comma-separated string of values (CSV).
Since: 1.0.9
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
arr | json data | array | - | true | - |
delimiter | delimiter | string | - | false | ',' |
Returns: string
Example:
arrayToCSV([
['a', 'b'],
['c', 'd']
])
// '"a", "b" \n "c", "d"'
arrayToCSV(
[
['a', 'b'],
['c', 'd']
],
';'
)
// '"a"; "b"\n "c"; "d"'
arrayToCSV([
['a', '"b" great'],
['c', 3.1415]
])
// '"a", """b"" great"\n "c",3.1415'
declare function arrayToCSV<T extends unknown[][]>(data: T, delimiter?: string): string
Converts a comma-separated string of values (CSV) to an array of 2D objects. The first line of the string is used as the header line.
Since: 1.0.9
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
data | csv data | string | - | true | - |
delimiter | delimiter | string | - | false | ',' |
Returns: string
Example:
CSVToJSON('col1,col2\\na,b\\\nc,d')
// `[{'col1': 'a', 'col2': 'b'}, {'col1': 'c', 'col2': 'd'}]`
CSVToJSON('col1;col2\\\na;b\\\nc;d', ';')
// `[{'col1': 'a', 'col2': 'b'}, {'col1': 'c', 'col2': 'd'}]`
declare function CSVToJSON(data: string, delimiter?: string): any[]
Converts an array of objects to a comma-separated value (CSV) string containing only the specified columns.
Since: 1.0.9
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
arr | json data | array | - | true | - |
columns | the specified columns | array | - | true | - |
delimiter | delimiter | string | - | false | ',' |
Returns: string
Example:
JSONToCSV([{ a: 1, b: 2 }, { a: 3, b: 4, c: 5 }, { a: 6 }, { b: 7 }], ['a', 'b']) // 'a,b\n "1", "2"\n "3", "4"\n "6",""\n"", "7"'
JSONToCSV([{ a: 1, b: 2 }, { a: 3, b: 4, c: 5 }, { a: 6 }, { b: 7 }], ['a', 'b'], ';') // 'a;b\n "1"; "2"\n "3"; "4"\n "6";""\n""; "7"'
declare const JSONToCSV: (arr: any[], columns: any[], delimiter?: string) => string
Converts RGB component values to color codes.
Since: 1.0.9
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
r | the 1st value of RGB | number | - | true | - |
g | RGB's 2nd value | number | - | true | - |
b | RGB's 3nd value | number | - | true | - |
Returns: string
Example:
RGBToHex(255, 165, 1) // 'ffa501'
declare const RGBToHex: (r: number, g: number, b: number) => string
Find the intersection of multiple arrays
Since: 2.2.1
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
...arr | array targets | array | - | true | - |
Returns: array
Example:
intersect([1, 2], [2, 3, 4], [2, 8], [2, '33']) // [2]
declare function intersect<T = unknown>(...args: T[][]): T[]
Find the concatenation of multiple arrays
Since: 2.2.1
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
...arr | array targets | array | - | true | - |
Returns: array
Example:
union([1, 2], [2, '33']) // [1, 2, '33']
declare function union<T = unknown>(...args: T[][]): T[]
Find the set of differences of multiple arrays that belong to A but not to B/C/D... of the elements of
Since: 2.2.1
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
...arr | array targets | array | - | true | - |
Returns: array
Example:
minus([1, 2], [2, '33'], [2, 4]) // [1]
declare function minus<T = unknown>(...args: T[][]): T[]
Find the complement of multiple arrays
Since: 2.2.1
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
...arr | array targets | array | - | true | - |
Returns: array
Example:
complement([1, 2], [2, '33'], [2]) // [1, '33']
declare function complement<T = unknown>(...args: T[][]): T[]
Whether the array contains the specified element
Since: 2.2.1
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
arr | array target | array | - | true | - |
item | any array member | any | - | true | - |
Returns: boolean
Example:
contains([1, 2], 2) // true
contains([1, 2], 3) // false
declare function contains(arr: any[], item: any): boolean
Array de-duplication
Since: 2.2.1
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
arr | array target | array | - | true | - |
Returns: array
Example:
unique([1, 2, 2, '33']) // [1, 2, '33']
declare function unique<T = unknown>(arr: T[]): T[]
ipv6 address completion
Since: 2.2.2
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
ip | ip address | string | - | true | - |
Returns: string
Example:
fillIPv6('2409:8005:800::2') // '2409:8005:0800:0000:0000:0000:0000:0002'
fillIPv6('2409:8005:800::1c') // '2409:8005:0800:0000:0000:0000:0000:001c'
declare function fillIPv6(ip: string): string
Get array, object property values based on path string
Since: 2.2.4
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
target | target array, object | array /object | - | true | - |
prop | query target, can pass function | string /function | - | true | - |
Returns: any
Example:
const target = {
a: 1,
b: [
{
c: 2
}
]
}
getProperty(target, 'a') // 1
getProperty(target, 'b[0].c') // 2
getProperty(target, () => 'a') // 1
declare function getProperty(
target: any,
prop:
| string
| {
(): string
}
): any
Set array, object property values based on path string
Since: 2.7.0
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
target | target array, object | array /object | - | true | - |
prop | set target, can pass function, 'a' | 'a[1].c' | string /function | - | true | - |
value | value | any | - | true | - |
Returns: any
Example:
const target = {
a: 1,
b: [
{
c: 2
}
]
}
setProperty(target, 'a') // 1
setProperty(target, 'b[0].c') // 2
setProperty(target, () => 'a') // 1
declare function setProperty(
target: any,
prop:
| string
| {
(): string
},
value: any
): any
load resources dynamically, support js, images, css links, css style strings
Since: 2.8.0
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
url | link to the resource, type must be passed when passing in styleString | string | - | true | - |
options | parameters: attrs, props, force | SourceOptions | - | false | - |
Returns: boolean | imageUrl
Example:
loadSource('/source/url', options)
import { ImageAttributes } from 'mount-image'
import { LinkAttributes } from 'mount-css'
import { ScriptAttributes } from 'mount-script'
import { StyleAttributes } from 'mount-style'
declare function loadSource(
url: string,
option: SourceFileType | SourceOptions
): Promise<boolean | string>
declare type SourceFileType = 'js' | 'img' | 'css' | 'style' | string
declare interface SourceOptions {
type: SourceFileType
attrs?: LinkAttributes | StyleAttributes | ScriptAttributes | ImageAttributes
props?: LinkAttributes | StyleAttributes | ScriptAttributes | ImageAttributes
force?: boolean
}
dynamically load css link resources
Since: 2.8.0
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
url | resource url | string | - | true | - |
options | parameters: attrs, props, force | CssOptions | - | false | - |
Returns: boolean
Example:
mountCss('/source/url', options)
declare interface CssOptions {
attrs?: LinkAttributes
props?: LinkAttributes
force?: boolean
}
declare interface HTMLLinkElementEX extends HTMLLinkElement {
onreadystatechange?: any
readyState?: 'loaded' | 'complete'
}
declare type LinkAttributes = Pick<
HTMLLinkElement,
| 'as'
| 'charset'
| 'crossOrigin'
| 'disabled'
| 'href'
| 'hreflang'
| 'imageSizes'
| 'imageSrcset'
| 'integrity'
| 'media'
| 'referrerPolicy'
| 'rel'
| 'rev'
| 'target'
| 'type'
>
declare function mountCss(src: string, option?: CssOptions): Promise<boolean>
load image resource dynamically
Since: 2.8.0
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
url | resource url | string | - | true | - |
options | parameters: attrs, props, force | ImgOptions | - | false | - |
Returns: boolean | imageUrl
Example:
mountImg('/source/url', options)
declare interface HTMLImageElementEX extends HTMLImageElement {
onreadystatechange?: any
readyState?: 'loaded' | 'complete'
}
declare type ImageAttributes = Pick<
HTMLImageElement,
| 'align'
| 'alt'
| 'border'
| 'crossOrigin'
| 'decoding'
| 'height'
| 'hspace'
| 'isMap'
| 'loading'
| 'longDesc'
| 'lowsrc'
| 'name'
| 'referrerPolicy'
| 'sizes'
| 'src'
| 'srcset'
| 'useMap'
| 'vspace'
| 'width'
>
declare interface ImgOptions {
attrs?: ImageAttributes
props?: ImageAttributes
force?: boolean
}
declare function mountImage(src: string, option?: ImgOptions): Promise<boolean | string>
load js link resources dynamically
Since: 2.8.0
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
url | resource url | string | - | true | - |
options | parameters: attrs, props, force | JsOptions | - | false | - |
Returns: boolean
Example:
mountJs('/source/url', options)
declare interface HTMLScriptElementEX extends HTMLScriptElement {
onreadystatechange?: any
readyState?: 'loaded' | 'complete'
}
declare interface JsOptions {
attrs?: ScriptAttributes
props?: ScriptAttributes
force?: boolean
}
declare function mountJs(src: string, option?: JsOptions): Promise<boolean>
declare type ScriptAttributes = Pick<
HTMLScriptElement,
| 'async'
| 'charset'
| 'crossOrigin'
| 'defer'
| 'event'
| 'htmlFor'
| 'integrity'
| 'noModule'
| 'referrerPolicy'
| 'src'
| 'text'
| 'type'
>
load css styles dynamically
Since: 2.8.0
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
url | resource url | string | - | true | - |
options | parameters: attrs, props, force | StyleOptions | - | false | - |
Returns: boolean
Example:
mountStyle('/source/url', options)
declare function mountStyle(css: string, option?: StyleOptions): Promise<boolean>
declare type StyleAttributes = Pick<HTMLStyleElement, 'disabled' | 'media' | 'type'>
declare interface StyleOptions {
attrs?: StyleAttributes
props?: StyleAttributes
}
Image preloading
Since: 5.5.0
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
images | images url | string array | - | true | - |
Returns: void
Example:
preloader('path/of/image')
preloader(['path/of/image'])
declare function preloader(images: string): HTMLImageElement
declare function preloader(images: string[]): Record<string, HTMLImageElement>
v5.8.1 Support throw on timeout
waiting for a while
Since: 5.5.0
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
milliseconds | waiting time (milliseconds) | number | - | true | - |
throwOnTimeout | throw on timeout | boolean | - | false | false |
Returns: Promise<void>
Example:
waiting(2000)
await waiting(2000, true)
// reject
declare function waiting(milliseconds: number, throwOnTimeout?: boolean): Promise<void>
Async await wrapper for easy error handling
v5.7.0 Extend awaitTo to support passing in multiple promises
Since: 5.2.0
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
promise | promise function | Promise Promise[] | - | true | - |
...promises | Promise rest params | Promise[] | - | false | - |
Returns: [Error, undefined]
or [null, data | data[]]
Example:
import { awaitTo as to } from 'js-cool'
// 1. simple use
const [err, data] = await to(new Promise())
if (err) {
// handle request error
}
// 2. Pass in multiple promises
const [err, data] = await to(promise1, promise2)
// or
const [err, data] = await to([promise1, promise2])
declare function awaitTo<T, E = Error>(promise: Promise<T>): Promise<[E, undefined] | [null, T]>
arrayBuffer to base64
Since: 5.13.0
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
input | arrayBuffer data | ArrayBuffer | - | true | - |
mime | image mime | String | - | false | image/png |
Returns: String
Example:
arrayBufferToBase64(arrayBuffer, 'image/png')
// data:image/png;base64,xxxxxxxxxxxx
declare function arrayBufferToBase64(input: ArrayBuffer, mime?: string): string
arrayBuffer to blob
Since: 5.13.0
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
input | arrayBuffer data | ArrayBuffer | - | true | - |
mime | image mime | String | - | false | image/png |
Returns: Blob
Example:
arrayBufferToBase64(arrayBuffer, 'image/png')
// Blob
declare function arrayBufferToBlob(input: ArrayBuffer, mime?: string): Blob
base64 to arrayBuffer
Since: 5.13.0
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
input | base64 string | String | - | true | - |
Returns: ArrayBuffer
Example:
base64ToArrayBuffer(base64)
// ArrayBuffer
declare function base64ToArrayBuffer(input: string): ArrayBuffer
base64 to blob
Since: 5.13.0
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
input | base64 string | String | - | true | - |
Returns: Blob
Example:
base64ToBlob(base64)
// Blob
declare function base64ToBlob(input: string): Blob
base64 to file
Since: 5.13.0
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
input | base64 string | String | - | true | - |
fileName | file name | String | - | true | - |
Returns: File
Example:
base64ToFile(base64, 'image.png')
// File
declare function base64ToFile(input: string, fileName: string): File
blob to arrayBuffer
Since: 5.13.0
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
input | blob data | Blob | - | true | - |
Returns: ArrayBuffer
Example:
blobToArrayBuffer(blob).then(data => {
// ArrayBuffer
})
declare function blobToArrayBuffer(input: Blob): Promise<ArrayBuffer | null>
blob to base64
Since: 5.13.0
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
input | blob data | Blob | - | true | - |
Returns: String
Example:
blobToBase64(blob).then(data => {
// data:image/png;base64,xxxxxxxxxxxx
})
declare function blobToBase64(input: Blob): Promise<string | null>
blob to url
Since: 5.13.0
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
input | blob data | Blob | - | true | - |
Returns: Object
Example:
blobToUrl(blob)
// blob:xxxxxxx
declare function blobToUrl(input: Blob): string
file to base64
Since: 5.13.0
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
input | file data | File | - | true | - |
Returns: String
Example:
fileToBase64(file).then(data => {
// data:image/png;base64,xxxxxxxxxxxx
})
declare function fileToBase64(input: File): Promise<string | null>
svg to blob
Since: 5.13.0
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
input | svg string | String | - | true | - |
Returns: Blob
Example:
svgToBlob(svg)
// Blob
declare function svgToBlob(input: string): Blob
url to blob
Since: 5.13.0
Arguments:
Parameters | Description | Type | Optional | Required | Default |
---|---|---|---|---|---|
input | url | String | - | true | - |
Returns: Blob
Example:
urlToBlob(url).then(blob => {
// Blob
})
declare function urlToBlob(input: string): Promise<Blob | null>
Please open an issue here.
FAQs
Collection of common JavaScript / TypeScript utilities
The npm package js-cool receives a total of 196 weekly downloads. As such, js-cool popularity was classified as not popular.
We found that js-cool demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
Security News
NVD’s backlog surpasses 20,000 CVEs as analysis slows and NIST announces new system updates to address ongoing delays.
Security News
Research
A malicious npm package disguised as a WhatsApp client is exploiting authentication flows with a remote kill switch to exfiltrate data and destroy files.