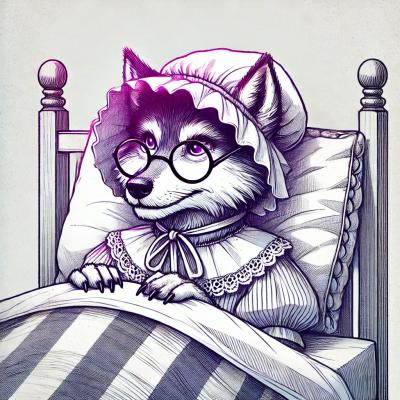
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Mocking framework for javascript, inspired by googlemock C++ framework. This project is still under construction ...
jsmock is published on npm
> npm install --save-dev jsmock
const Mock = require('jsmock').Mock;
let foo = {
bar: (a, b) => {
return a + b
}
};
let fooMock = new Mock(foo);
Now fooMock is a mock object wrapping foo. All functions of original object have been replaced and any call to foo.bar will cause an expection to be thrown.
expect(foo.bar.bind(foo)).to.throw(Error);
Expectation of function call is defined by calling expectCall on mock object.
fooMock.expectCall('bar');
By default this will setup an expectation of single call to foo.bar function with any arguments. As there is no action specified yet, nothing will be returned and no side effects will be observed.
Matcher validates that call of mocked function is valid for given expectation. If no explicit matcher is specified expectation will be executed for any call to mocked function. Matcher can be specified as a predicate or simply as an arguments list to be verified against actual call.
fooMock.expectCall('bar', (a,b) => a > b);
foo.bar(3,2); // OK - 3 > 2
foo.bar(1,4); // KO - excpetion thrown
fooMock.expectCall('bar', 1, 8);
foo.bar(1, 8); // OK
foo.bar(1, 0); // KO
Mathcher can be specified directly in arguments of the expectCall method or by calling matching function on mock object. Note that expectCall returns mock object making call chain possible:
fooMock.expectCall('bar').matching((a,b) => a < b);
fooMock.expectCall('bar').matching(1,4);
Action is an object encapsulating function to be executed instead of original code on mocked object. Besides a function each action specifies also number of times it's expected to be called. Each expectation can have multiple actions defined, which will be executed in order of creation.
fooMock.expectCall('bar')
.willOnce((a,b) => a * b) // First call will return multiplication of arguments
.willRepeteadly((a,b) => b) // All following calls will return second argument
You need to pay attention to order of specifying actions. If an action with unlimited number of expected calls preceeds other actions, it will prevent their execution and cause mock to be invalid.
FAQs
Mocking framework for javascript
The npm package jsmock receives a total of 0 weekly downloads. As such, jsmock popularity was classified as not popular.
We found that jsmock demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.