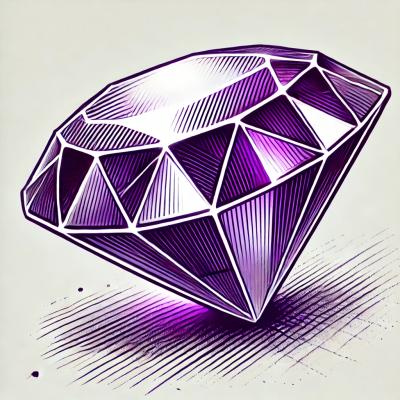
Security News
RubyGems.org Adds New Maintainer Role
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
json-parser
Advanced tools
JSON parser to parse JSON object and MAINTAIN comments.
This is a very low level module. For most situations, recommend to use comment-json
instead.
2.x
json-parser@2.x
brings some breaking changes by completely refactoring with Symbol
s
$ npm i json-parser
const {parse} = require('json-parser')
parse(text, reviver? = null, remove_comments? = false)
: object | string | number | boolean | null
string
The string to parse as JSON. See the JSON object for a description of JSON syntax.Function() | null
Default to null
. It acts the same as the second parameter of JSON.parse
. If a function, prescribes how the value originally produced by parsing is transformed, before being returned.boolean = false
If true, the parsed JSON Object won't contain commentsReturns object | string | number | boolean | null
corresponding to the given JSON text.
content
/**
before-all
*/
// before-all
{
// before
/* before */
"foo" /* after-prop:foo */ : // after-comma:foo
1 // after-value:foo
// after-value:foo
, // after-comma:foo
// after-comma: foo
"bar": [ // before
// before
"baz" // after-value:0
// after-value:0
, // after-comma: 0
"quux"
// after-value:1
] // after-value:bar
// after-value:bar
}
// after-all
console.log(parse(content))
And the result will be:
{
// Comments before the JSON object
[Symbol.for('before-all')]: [{
type: 'BlockComment',
value: '\n before-all\n ',
inline: false
}, {
type: 'LineComment',
value: ' before-all',
inline: false
}],
...
[Symbol.for('after-prop:foo')]: [{
type: 'BlockComment',
value: ' after-prop:foo ',
inline: true
}],
// The real value
foo: 1,
bar: [
"baz",
"quux,
// The property of the array
[Symbol.for('after-value:0')]: [{
type: 'LineComment',
value: ' after-value:0',
inline: true
}, ...],
...
]
}
There are SEVEN kinds of symbol properties:
// comment tokens before the JSON object
Symbol.for('before-all')
// comment tokens before any properties/items inside an object/array
Symbol.for('before')
// comment tokens after property key `prop` and before colon(`:`)
Symbol.for(`after-prop:${prop}`)
// comment tokens after the colon(`:`) of property `prop` and before property value
Symbol.for(`after-colon:${prop}`)
// comment tokens after the value of property `prop`/the item of index `prop`
// and before the key-value/item delimiter(`,`)
Symbol.for(`after-value:${prop}`)
// comment tokens after the comma of `prop`-value pair
// and before the next key-value pair/item
Symbol.for(`after-comma:${prop}`)
// comment tokens after the JSON object
Symbol.for('after-all')
And the value of each symbol property is an array of CommentToken
interface CommentToken {
type: 'BlockComment' | 'LineComment'
// The content of the comment, including whitespaces and line breaks
value: string
// If the start location is the same line as the previous token,
// then `inline` is `true`
inline: boolean
}
console.log(parse(content, null, true))
And the result will be:
{
foo: 1,
bar: [
"baz",
"quux"
]
}
const parsed = parse(`
// comment
1
`)
console.log(parsed === 1)
// false
If we parse a JSON of primative type with remove_comments:false
, then the return value of parse()
will be of object type.
The value of parsed
is equivalent to:
const parsed = new Number(1)
parsed[Symbol.for('before-all')] = [{
type: 'LineComment',
value: ' comment',
inline: false
}]
Which is similar for:
Boolean
typeString
typeFor example
const parsed = parse(`
"foo" /* comment */
`)
Which is equivalent to
const parsed = new String('foo')
parsed[Symbol.for('after-all')] = [{
type: 'BlockComment',
value: ' comment ',
inline: true
}]
But there is one exception:
const parsed = parse(`
// comment
null
`)
console.log(parsed === null) // true
MIT
FAQs
JSON parser to parse JSON object and MAINTAIN comments.
We found that json-parser demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.
Security News
Research
Socket's threat research team has detected five malicious npm packages targeting Roblox developers, deploying malware to steal credentials and personal data.