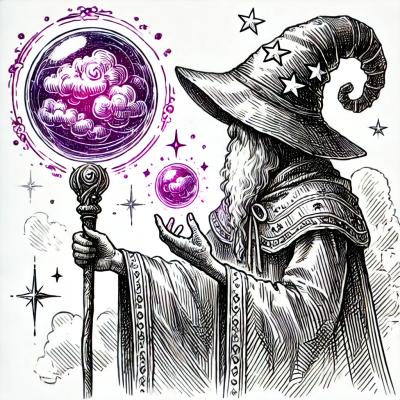
Security News
Cloudflare Adds Security.txt Setup Wizard
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
json-stringify-pretty-compact
Advanced tools
The best of both `JSON.stringify(obj)` and `JSON.stringify(obj, null, indent)`.
The json-stringify-pretty-compact npm package is designed to provide a more compact and human-readable JSON stringification. It aims to strike a balance between readability and compactness by using a more intelligent formatting approach compared to the default JSON.stringify method.
Compact and Pretty JSON Stringification
This feature allows you to convert a JavaScript object into a JSON string that is both compact and easy to read. The output is more human-friendly compared to the default JSON.stringify method.
const stringify = require('json-stringify-pretty-compact');
const obj = { foo: 'bar', baz: [1, 2, 3], nested: { a: 1, b: 2 } };
console.log(stringify(obj));
Custom Indentation
This feature allows you to specify the number of spaces to use for indentation, giving you control over the readability of the output.
const stringify = require('json-stringify-pretty-compact');
const obj = { foo: 'bar', baz: [1, 2, 3], nested: { a: 1, b: 2 } };
console.log(stringify(obj, { indent: 4 }));
Maximum Line Length
This feature allows you to set a maximum line length for the output, ensuring that the JSON string does not exceed a specified width, which can be useful for maintaining readability in narrow spaces.
const stringify = require('json-stringify-pretty-compact');
const obj = { foo: 'bar', baz: [1, 2, 3], nested: { a: 1, b: 2 } };
console.log(stringify(obj, { maxLength: 80 }));
The prettyjson package is another alternative that focuses on making JSON data more readable. It offers more customization options for colors and indentation but may not be as compact as json-stringify-pretty-compact.
The json-pretty-print package provides basic pretty-printing capabilities for JSON data. It is simpler and may not offer the same level of compactness and customization as json-stringify-pretty-compact.
The output of JSON.stringify comes in two flavors: compact and pretty. The former is usually too compact to be read by humans, while the latter sometimes is too spacious. This module trades performance for a compromise between the two. The result is a pretty compact string, where “pretty” means both “kind of” and “nice”.
{
"bool": true,
"short array": [1, 2, 3],
"long array": [
{"x": 1, "y": 2},
{"x": 2, "y": 1},
{"x": 1, "y": 1},
{"x": 2, "y": 2}
]
}
While the “pretty” mode of JSON.stringify puts every item of arrays and objects on its own line, this module puts the whole array or object on a single line, unless the line becomes too long (the default maximum is 80 characters). Making arrays and objects multi-line is the only attempt made to enforce the maximum line length; if that doesn’t help then so be it.
npm install json-stringify-pretty-compact
const stringify = require("json-stringify-pretty-compact");
stringify(obj, options = {})
It’s like JSON.stringify(obj, options.replacer, options.indent)
, except that objects and arrays are on one line if they fit (according to options.maxLength
).
options
:
stringify(obj, {maxLength: 0, indent: indent})
gives the exact same result as JSON.stringify(obj, null, indent)
. (However, if you use a replacer
, integer keys might be moved first.)
stringify(obj, {maxLength: Infinity})
gives the exact same result as JSON.stringify(obj)
, except that there are spaces after colons and commas.
Want more options? Check out @aitodotai/json-stringify-pretty-compact!
MIT.
Version 3.0.0 (2021-02-20)
JSON.stringify
. It’s slightly stricter, but I doubt you’ll notice a difference."type": "commonjs", "exports": "./index.js", "types": "index.d.ts"
to package.json. I doubt you’ll notice any difference from this either.FAQs
The best of both `JSON.stringify(obj)` and `JSON.stringify(obj, null, indent)`.
The npm package json-stringify-pretty-compact receives a total of 802,173 weekly downloads. As such, json-stringify-pretty-compact popularity was classified as popular.
We found that json-stringify-pretty-compact demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
Security News
The Socket Research team breaks down a malicious npm package targeting the legitimate DOMPurify library. It uses obfuscated code to hide that it is exfiltrating browser and crypto wallet data.
Security News
ENISA’s 2024 report highlights the EU’s top cybersecurity threats, including rising DDoS attacks, ransomware, supply chain vulnerabilities, and weaponized AI.