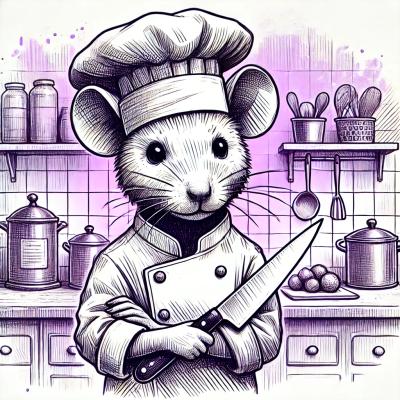
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
json-to-pretty-yaml
Advanced tools
The json-to-pretty-yaml npm package is a utility that allows you to convert JSON objects to YAML format with pretty-printing. This can be useful for configuration files, data serialization, and more.
Convert JSON to YAML
This feature allows you to convert a JSON object to a YAML string. The resulting YAML string is formatted in a human-readable way.
const jsonToPrettyYaml = require('json-to-pretty-yaml');
const jsonObject = { name: 'John', age: 30, city: 'New York' };
const yamlString = jsonToPrettyYaml.stringify(jsonObject);
console.log(yamlString);
Pretty-print YAML
This feature allows you to pretty-print the YAML string with a specified indentation level. This can make the YAML output more readable.
const jsonToPrettyYaml = require('json-to-pretty-yaml');
const jsonObject = { name: 'John', age: 30, city: 'New York' };
const yamlString = jsonToPrettyYaml.stringify(jsonObject, { indent: 4 });
console.log(yamlString);
js-yaml is a popular package for parsing and dumping YAML. It provides more comprehensive YAML support, including schema validation and custom types. Compared to json-to-pretty-yaml, js-yaml offers more advanced features but may be more complex to use for simple JSON to YAML conversions.
The yaml package is another library for parsing and stringifying YAML. It supports a wide range of YAML features and is highly configurable. It is similar to js-yaml in terms of functionality but offers a different API. It is more feature-rich compared to json-to-pretty-yaml but also more complex.
A node module to convert JSON to pretty YAML
npm install --save json-to-pretty-yaml
index.js
const fs = require('fs');
const YAML = require('json-to-pretty-yaml');
const json = require('input.json');
const data = YAML.stringify(json);
fs.writeFile('output.yaml', data);
input.json
{
"a": 1,
"b": 2,
"c": [
{
"d": "cool",
"e": "new"
},
{
"f": "free",
"g": "soon"
}
]
}
output.yaml
a: 1
b: 2
c:
- d: "cool"
e: "new"
- f: "free"
g: "soon"
npm test
FAQs
A node module to convert JSON to YAML
The npm package json-to-pretty-yaml receives a total of 2,123,592 weekly downloads. As such, json-to-pretty-yaml popularity was classified as popular.
We found that json-to-pretty-yaml demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.