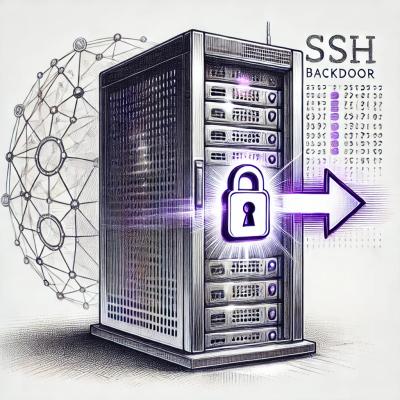
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
json2csvexporter
Advanced tools
This is a simple little library for exporting simple JSON structures to a CSV file. The download will trigger the user to save the file to their system. The library itself does not handle any File I/O.
Currently, json2csvexporter does not support nested JSON data. As such, you will probably need to reduce complex structures into a simple key: value
schema.
To install:
npm i json2csvexporter --save
Table of contents:
In this simple example we take a JSON object and print all of the properties in it to the CSV. There is also an online demo available via RequireBin.
import CSVExportService from 'json2csvexporter';
...
const vehiclesJSON = [
{id: 1, make: 'Toyota', model: 'Corolla', year: 2014},
{id: 2, make: 'Ford', model: 'Mustang', year: 2012},
{id: 3, make: 'Toyota', model: '', year: ''}
];
const exporter = CSVExportService.create();
exporter.downloadCSV(vehiclesJSON);
This will generate the following CSV:
id,make,model,year
1,Toyota,Corolla,2014
2,Ford,Mustang,2012
3,Toyota,,
In this example, we will take the same JSON object from the previous example, but only print a list of properties that we want. We will also decorate the headers with different names.
import CSVExportService from 'json2csvexporter';
...
const csvColumnsList = ['id', 'make', 'model', 'year'];
const csvColumnsMap = {
id: 'ID',
make: 'Make',
model: 'Model Name',
year: 'Year'
};
const vehiclesJSON = [
{id: 1, make: 'Toyota', model: 'Corolla', year: 2014},
{id: 2, make: 'Ford', model: 'Mustang', year: 2012},
{id: 3, make: 'Toyota', model: '', year: ''}
];
const exporter = CSVExportService.create({
columns: csvColumnsList,
headers: csvColumnsMap,
includeHeaders: true,
});
exporter.downloadCSV(vehiclesJSON);
This will generate the following CSV:
ID,Make,Model Name,Year
1,Toyota,Corolla,2014
2,Ford,Mustang,2012
3,Toyota,,
In this example, we will make a TSV file and create formatting functions to style the content of certain properties.
import CSVExportService from 'json2csvexporter';
...
// using the same vehiclesJSON as before...
const exporter = CSVExportService.create({
contentType: 'text/tsv',
filename: 'newformat.tsv',
delimiter: '\t',
formatters: {
id: (id) => {
return `#${id}`;
},
model: (model) => {
return model || 'Unknown model';
},
year: (year) => {
return year || 'Unknown year';
}
}
});
exporter.downloadCSV(vehiclesJSON);
This will generate the following TSV:
id make model year
#1 Toyota Corolla 2014
#2 Ford Mustang 2012
#3 Toyota Unknown model Unknown year
import CSVExportService from 'json2csvexporter';
...
const vehiclesJSON = [
{id: 1, make: 'Toyota', model: 'Corolla', year: 2014},
{id: 2, make: 'Ford', model: 'Mustang', year: 2012},
{id: 3, make: 'Toyota', model: '', year: ''}
];
const exporter = CSVExportService.create();
exporter.dataToString(vehiclesJSON);
This will return the CSV as a JavaScript String. This allows you to further manipulate the data, save to a file, a database or anything else.
Similarly, calling:
exporter.createCSVBlob(vehiclesJSON);
Will return a Blob {size: 76, type: "text/csv"}
, or in other words, a regular JavaScript blob object that is encoded as the type you specified (in this case a plain text/csv). You then manipulate this Blob object however you like.
When creating a new CSVExportService
you can pass in an options objects. These are the options that you can use:
columns
: an Array of Strings. Each element represents a property of the JSON object that should be extracted. If it is not provided, then the exporter will iterate through all of the properties.
contentType
: the content type of the file. Default is text/csv
delimiter
: the delimiter to separate values. The default is a comma.
formatters
: an Object. Each property defines how to format a value corresponding to the same key.
headers
: an Object. Works as a map to stylize keys in the header. E.g. {firstName: 'First Name'}
includeHeaders
: a Boolean. Defaults to true.
devMode
: a Boolean. Useful for debugging. Defaults to false.
The CSVExportService
implements two static methods: create(options)
which returns a new instance of CSVExportService and download(data, options)
which initializes a new service and downloads the data right away.
For more info, dive into the code. It is a very simple class.
![]() IE / Edge | ![]() Firefox | ![]() Chrome | ![]() Safari | ![]() Opera | ![]() iOS Safari |
---|---|---|---|---|---|
IE10, IE11, Edge | 4+ | 13+ | 5+ | 12+ | 7+ |
FAQs
A simple JSON to CSV converter.
The npm package json2csvexporter receives a total of 90 weekly downloads. As such, json2csvexporter popularity was classified as not popular.
We found that json2csvexporter demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.